Ajax Login Form with Bootstrap, jQuery, and PHP MySQL
Submitted by alpha_luna on Saturday, August 13, 2016 - 13:19.
In this tutorial, we are going to explain how to create Ajax Login Form with Bootstrap, jQuery, and PHP MySQL. For my Registration tutorial post using Ajax and jQuery, this is my continuation tutorial that I am going to create a simple yet useful login form with Bootstrap. The users type their email and password to log in and all the process will be done without web page refresh. Kindly download the source code below, and let’s start to code.
Read also: PHP Forms Submit without Page Refresh using jQuery and Ajax
Database Configuration
This file helps us to modify the database name.
Bootstrap Link
Kindly copy and paste this two files to load Bootstrap to your HEAD tag of your web page.
Login PHP Query
It contains PHP source code, this query verifies the email and password of the user. If the login information is a success it will display the ok message or if failed the information given from the user it will display the wrong details alert or message.
Wrong information in Login Form.
If the user successfully login before jump to the home page.
This is the home page.
Read also: PHP Forms Submit without Page Refresh using jQuery and Ajax
That's it, kindly click the "Download Code" button below for the full source code. And try it to customize your Login Form. Enjoy coding.
Hope that this simple yet useful tutorials that I created may help you to your future projects.
Share us your thoughts and comments below. Thank you so much for dropping by and reading this tutorial post. For more updates, don’t hesitate and feel free to visit this website more often and please share this with your friends or email me at [email protected]. Practice Coding. Thank you very much.
- <?php
- $database_host = "localhost";
- $database_user = "root";
- $database_password = "";
- $database_name = "login_ajax";
- try{
- $db_con = new PDO("mysql:host={$database_host};dbname={$database_name}",$database_user,$database_password);
- $db_con->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
- }
- catch(PDOException $display_message){
- echo $display_message->getMessage();
- }
- ?>
Markup Login Form
This source code below, where the user types their email and password to log in.
- <div class="signin-form">
- <div class="container">
- <form class="form-signin" method="post" id="login-form">
- <div id="error">
- <!-- error will be shown here ! -->
- </div>
- <div class="form-group">
- <input type="email" class="form-control" placeholder="Email address . . . . ." name="user_email" id="user_email" autofocus="autofocus" />
- </div>
- <div class="form-group">
- <input type="password" class="form-control" placeholder="Password . . . . . " name="password" id="password" />
- </div>
- <hr />
- <div class="form-group">
- <button type="submit" class="btn btn-primary" name="btn-login" id="btn-login">
- </button>
- </div>
- </form>
- </div>
- </div>
- <?php
- require_once 'dbconfig.php';
- {
- $password = ($user_password);
- try
- {
- $statement = $db_con->prepare("SELECT * FROM tbl_users WHERE user_email=:email");
- $row = $statement->fetch(PDO::FETCH_ASSOC);
- $count = $statement->rowCount();
- if($row['user_password']==$password){
- echo "Successfully Login"; // log in
- $_SESSION['user_session'] = $row['user_id'];
- }
- else{
- echo "Email or password does not exist."; // wrong details
- }
- }
- catch(PDOException $e){
- echo $e->getMessage();
- }
- }
- ?>
Output
Email and password validation.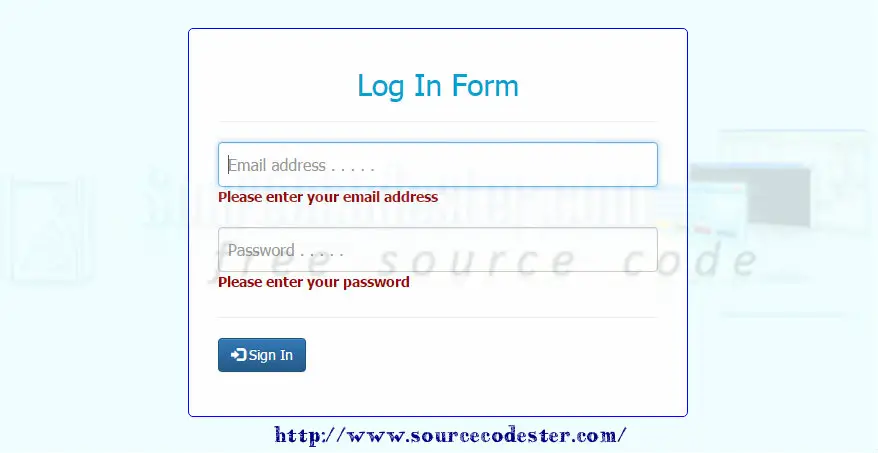
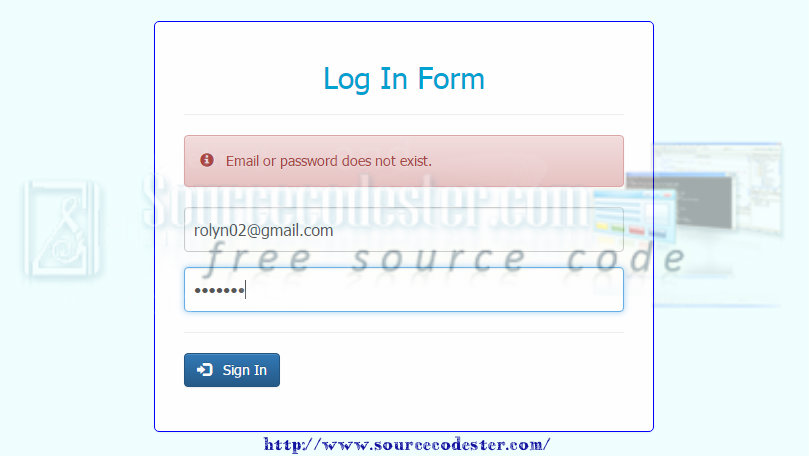
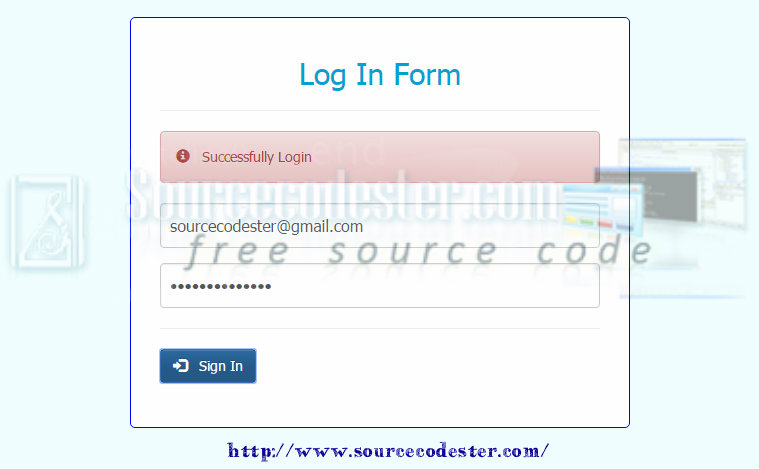
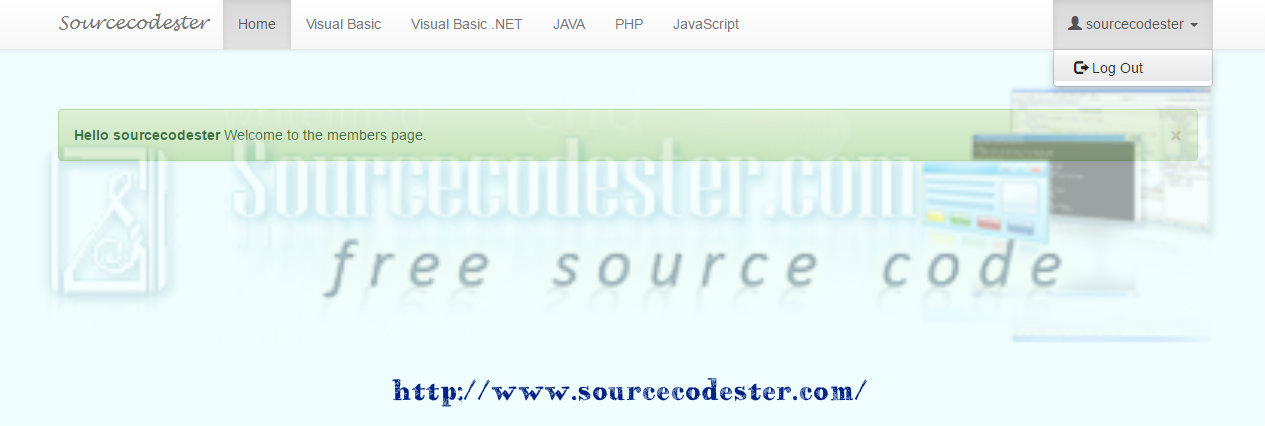
Add new comment
- 1130 views