Bootstrap Signup Form with Email Availability Check using jQuery, PHP, and MySQL
Submitted by alpha_luna on Tuesday, August 16, 2016 - 13:56.
Bootstrap Signup Form with Email Availability Check using jQuery PHP and MySQL
In this tutorial, we are going to create Bootstrap Signup Form with Email Availability Check using jQuery PHP and MySQL. This tutorial created to the advanced level of programming. Features of this simple tutorial are to check the email availability, live jQuery validation in every field, and signup process without refreshing the whole page. You can also check the live demo of this simple tutorial, so you can get an idea and you can try this out, let's start coding. Example email - [email protected] Download the source code below and try the existing email to sign up again to check if it is available or not.You can learn:
- Using jQuery Validation
- Email Availability Check
- Using JSON Response
- Signup Using Ajax
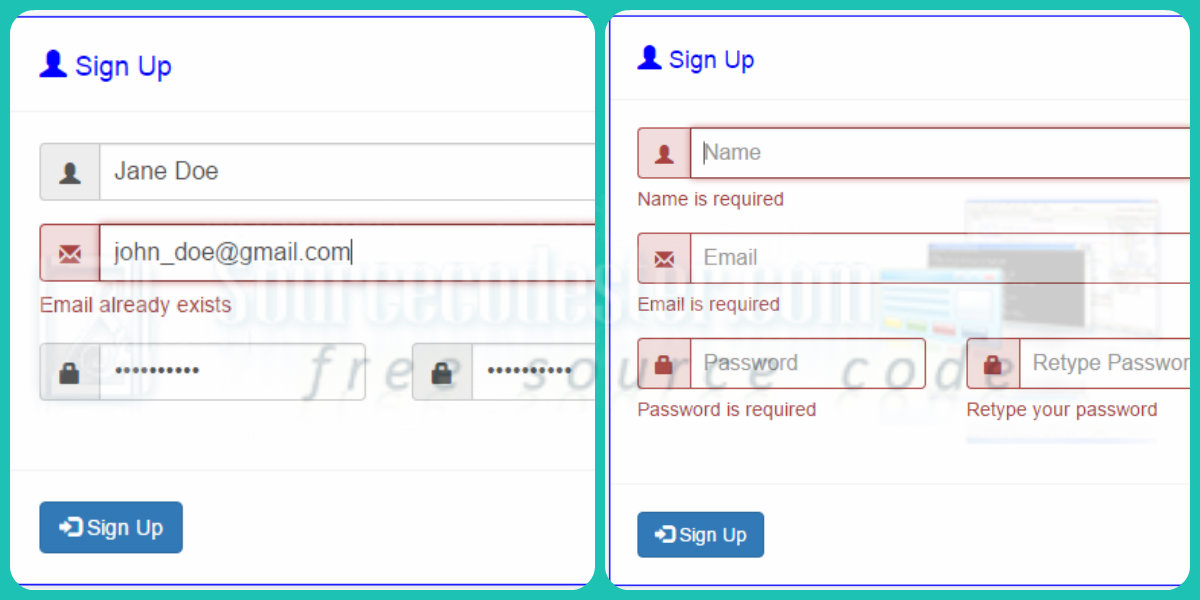
Database Table
For creating a database, kindly copy and paste this following SQL source code into your PHPMyAdmin to insert user details.- --
- -- Table structure for table `users`
- --
- CREATE TABLE `users` (
- `id` INT(11) NOT NULL,
- `name` VARCHAR(60) NOT NULL,
- `email` VARCHAR(60) NOT NULL,
- `password` VARCHAR(255) NOT NULL
- ) ENGINE=MyISAM DEFAULT CHARSET=latin1;
- --
- -- Dumping data for table `users`
- --
Database Connection
This is simple database connection file using PDO extension. Save it as "config.php".- <?php
- try{
- $DataBase_con = new PDO("mysql:host=".DataBasehost.";dbname=".DataBasename,DataBaseuser,DataBasePass);
- }catch(PDOException $sample){
- }
- ?>
INSERT Statement using PDO Extension
It contains PHP source code using PDO extension which you can insert new users details and store it into MySQL Table. Copy and paste and save it as "ajax-signup.php".- <?php
- require_once 'config.php';
- if ($_POST) {
- $name = $_POST['name'];
- $email = $_POST['email'];
- $pass = $_POST['cpassword'];
- // sha256 password hashing
- $statement = $DataBase_con->prepare('INSERT INTO users(name,email,password) VALUES(:name, :email, :pass)');
- $statement->bindParam(':name', $name);
- $statement->bindParam(':email', $email);
- $statement->bindParam(':pass', $password);
- $statement->execute();
- // check for successfull registration
- if ($statement->rowCount() == 1) {
- $response['status'] = 'success';
- $response['message'] = '<span class="glyphicon glyphicon-ok"></span> registered sucessfully, you may login now';
- } else {
- $response['status'] = 'error'; // could not register
- $response['message'] = '<span class="glyphicon glyphicon-info-sign"></span> could not register, try again later';
- }
- }
- ?>
Email Availability Check
This simple PHP source code using PDO extension use to check the email if it is available or not. It will give a message to a new user if it is already taken the email or not.- <?php
- require_once 'config.php';
- $email = $_POST['email'];
- $query = " SELECT email FROM users where email = :email ";
- $statement = $DataBase_con->prepare($query);
- if ($statement->rowCount() == 1) {
- echo 'false'; // email already taken
- } else {
- echo 'true';
- }
- }
- ?>
Output
Email Availability Check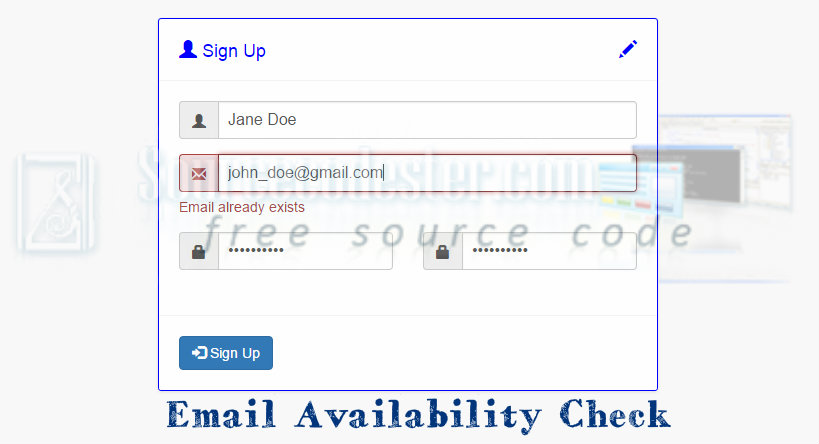
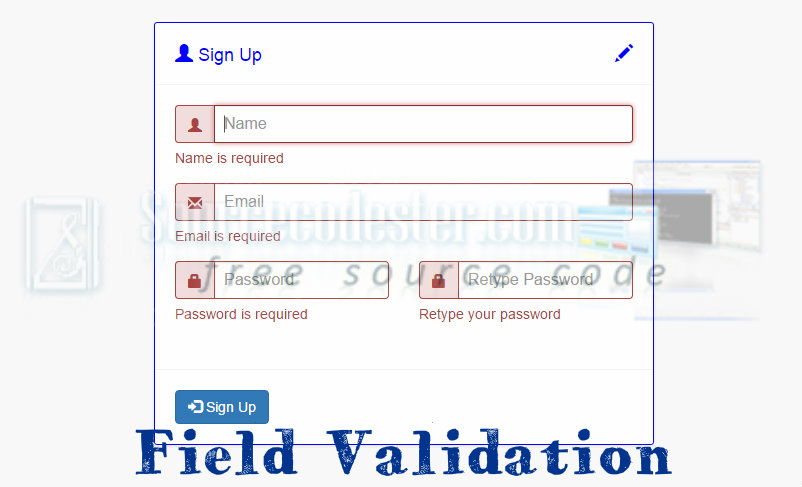