Good Day!!!
If you are looking for on
How To Create Import CSV/Excel File To MySQL Database Using PHP then you are at the right place. In this article, we are going to learn on how to import data using CSV/Excel File to MySQL database using PHP Language.
Let's start with:
We are going to make our database.
Creating our Table
To create a database:
- Open the PHPMyAdmin
- Create a database and name it as "import_file".
- After creating a database name, click the SQL and kindly copy the code below.
--
-- Table structure for table `user`
--
We are going to make our database connection.
Database Connection
This PHP Script is our database. Copy and paste this then save it as
"database.php".
We are going to make our form field.
Creating Form Field
This form field that the admin clicks the file button to import data into the database.
<form action="import_query.php" method="post" name="upload_excel" enctype="multipart/form-data">
<input type="file" multiple name="filename" id="filename">
<button type="submit" id="submit" name="submit" data-loading-text="Loading...">Upload
</button>
We are going to create CSV/Excel File in PHP query.
CSV/Excel File - PHP Script
This is the PHP codes for CSV/Excel File to import into MySQL database.
<?php
if (isset($_POST['submit']))
{
include('database.php');
//Import uploaded file to Database
$handle = fopen($_FILES['filename']['tmp_name'], "r");
while (($data = fgetcsv($handle, 1000, ",")) !== FALSE) {
mysql_query("INSERT into user (user_name, first_name, last_name, date_added)
values('$data[0]', '$data[1]', '$data[2]', NOW())");
}
//print "Import done";
echo "<script type='text/javascript'>alert('Successfully Imported a CSV File for User!');</script>";
echo "<script>document.location='index.php'</script>";
//view upload form
}
?>
This table where you can view the data after importing in the database.
<table border="1" cellspacing="5" cellpadding="5">
<thead>
<tr>
<th>UserName</th>
<th>FirstName</th>
<th>LastName</th>
<th>Date Added</th>
</tr>
</thead>
<?php
include ('database.php');
$id=$row['user_id'];
?>
<tbody>
<tr>
<td><?php echo $row['user_name']; ?></td>
<td><?php echo $row['first_name']; ?></td>
<td><?php echo $row['last_name']; ?></td>
<td>
<?php echo date("M d, Y h:i:s a",strtotime($row['date_added'])); ?></td>
</tr>
</tbody>
<?php } ?>
</table>
And, this is our style.
<style type="text/css">
body {
width:700px;
margin:auto;
}
label {
color: blue;
font-size: 18px;
font-weight: bold;
font-family: cursive;
margin-right: 10px;
}
input[type="file"] {
border: blue 1px solid;
padding: 8px;
color: blue;
font-size: 15px;
border-radius: 4px;
margin-right: 10px;
cursor:pointer;
}
button {
font-size: 18px;
border: blue 1px solid;
font-weight: bold;
padding: 8px;
background: azure;
color: blue;
border-radius: 4px;
cursor:pointer;
}
div {
border: blue 1px solid;
padding: 15px;
text-align: center;
border-radius: 4px;
background: azure;
}
table {
width: 100%;
text-align: center;
font-size: 18px;
font-family: cursive;
border: blue 1px solid;
background: azure;
}
th {
color:red;
}
td {
color:blue;
}
</style>
This is the result:
This is the data to be imported in MySQL Database.
After import the data in the MySQL Database. This is the result.
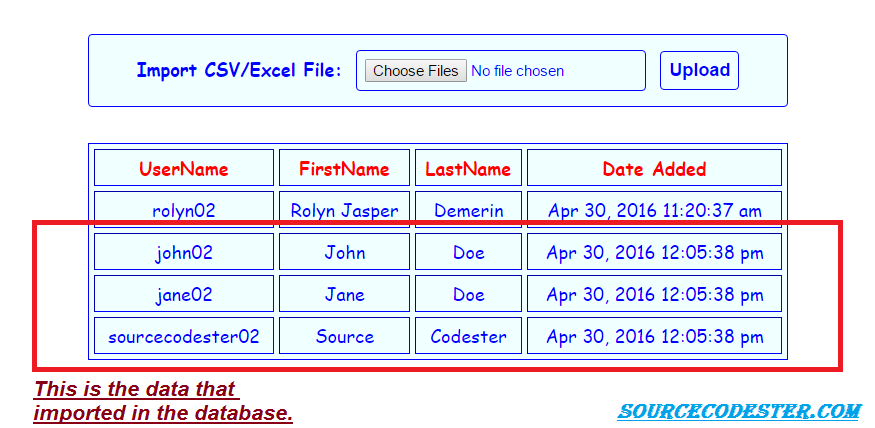
So, this is it, just follow the steps to have this CSV/Excel File Imported To MySQL Database or you can download the full source code below by clicking the
"Download Code" button below.
Share us your thoughts and comments below. Thank you so much for dropping by and reading this tutorial post. For more updates, don’t hesitate and feel free to visit this website more often and please share this with your friends or email me at
[email protected]. Practice Coding. Thank you very much.