AngularJS Inline Edit using PHP/MySQLi
Submitted by nurhodelta_17 on Thursday, January 18, 2018 - 20:56.
Getting Started
I've used CDN for Bootstrap and Angular JS so you need internet connection for them to work.Creating our Database
First, we are going to create our database and insert sample data. 1. Open phpMyAdmin. 2. Click databases, create a database and name it as angular. 3. After creating a database, click the SQL and paste the below codes. See image below for detailed instruction.- (1, 'Neovic', 'Devierte', 'Silay'),
- (2, 'Julyn', 'Divinagracia', 'E.B.'),
- (3, 'Gemalyn', 'Cepe', 'Bohol'),
- (4, 'Matet', 'Devierte', 'Silay City'),
- (5, 'Tintin', 'Devierte', 'Silay City'),
- (6, 'Bien', 'Devierte', 'Cebu City'),
- (9, 'Janna ', 'Atienza', 'Talisay City'),
- (10, 'Desire', 'Osorio', 'Bacolod City');
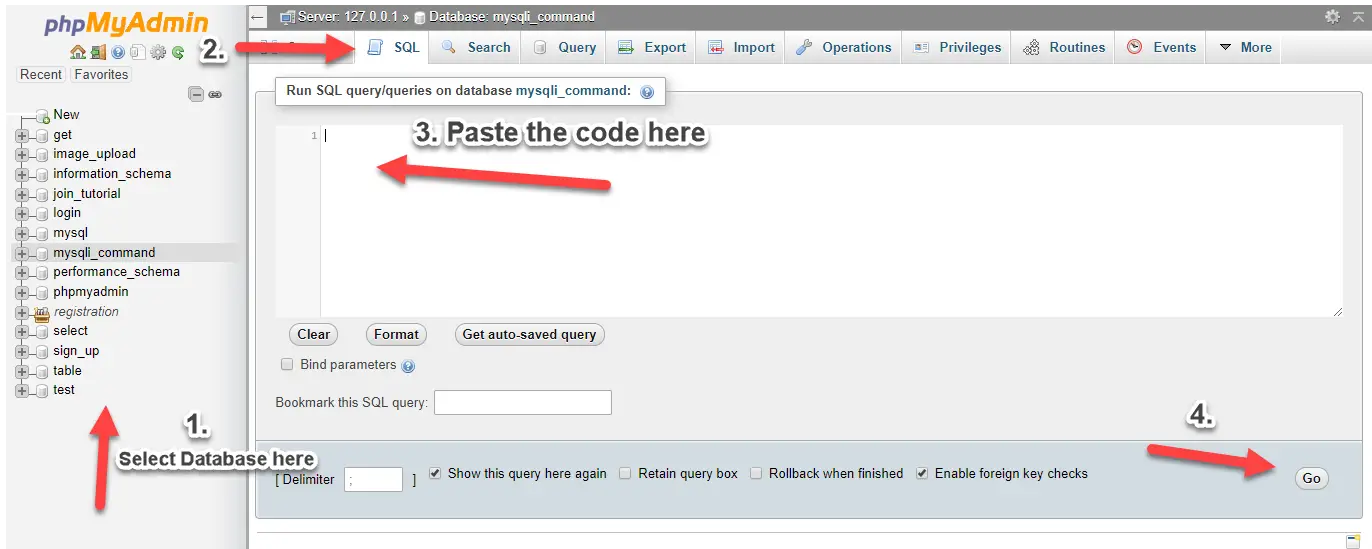
index.html
This is our index which contains our table.- <!DOCTYPE html>
- <html lang="en" ng-app="app">
- <head>
- <meta charset="utf-8">
- <link href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" rel="stylesheet">
- </head>
- <body ng-controller="memberdata" ng-init="fetch()">
- <div class="container">
- <div class="row">
- <div class="col-md-10 col-md-offset-1">
- <div class="alert alert-success text-center" ng-show="success">
- </div>
- <div class="alert alert-danger text-center" ng-show="error">
- </div>
- <table class="table table-bordered table-striped" style="margin-top:10px;">
- <thead>
- <tr>
- </tr>
- </thead>
- <tbody>
- <tr ng-repeat="member in members" ng-include="getTemplate(member)">
- </tr>
- </tbody>
- </table>
- <script type="text/ng-template" id="normal">
- </script>
- <script type="text/ng-template" id="edit">
- <td>
- </td>
- </script>
- </div>
- </div>
- </div>
- </body>
- </html>
angular.js
This contains our angular js script.- var app = angular.module('app', []);
- app.controller('memberdata', function($scope, $http){
- $scope.success = false;
- $scope.error = false;
- $scope.editMode = false;
- $scope.editmember = {};
- $scope.getTemplate = function (member) {
- if (member.memid === $scope.editmember.memid) return 'edit';
- else return 'normal';
- };
- $scope.fetch = function(){
- $http.get('fetch.php').success(function(data){
- $scope.members = data;
- });
- }
- $scope.edit = function(member){
- $scope.editmember = angular.copy(member);
- }
- $scope.save = function(editmember){
- $http.post('save.php', editmember)
- .success(function(data){
- console.log(data);
- if(data.error){
- $scope.error = true;
- $scope.success = false;
- $scope.message = data.message;
- }
- else{
- $scope.fetch();
- $scope.reset();
- $scope.success = true;
- $scope.error = false;
- $scope.message = data.message;
- }
- });
- }
- $scope.reset = function () {
- $scope.editmember = {};
- };
- $scope.clearMessage = function(){
- $scope.success = false;
- $scope.error = false;
- }
- });
fetch.php
This is our PHP api that fetches data from our MySQL Database.- <?php
- $conn = new mysqli('localhost', 'root', '', 'angular');
- $sql = "SELECT * FROM members";
- $query=$conn->query($sql);
- while($row=$query->fetch_array()){
- $output[] = $row;
- }
- ?>
save.php
Lastly, this is our PHP code/api in saving the changes we made to our table.- <?php
- $conn = new mysqli('localhost', 'root', '', 'angular');
- $memid = $data->memid;
- $firstname = $data->firstname;
- $lastname = $data->lastname;
- $address = $data->address;
- $sql = "UPDATE members SET firstname = '$firstname', lastname = '$lastname', address = '$address' WHERE memid = '$memid'";
- $query = $conn->query($sql);
- if($query){
- $out['message'] = "Member updated Successfully";
- }
- else{
- $out['error'] = true;
- $out['message'] = "Cannot update Member";
- }
- ?>
Add new comment
- 490 views