AngularJS Search Suggestion with PHP/MySQLi and UI-Router
Submitted by nurhodelta_17 on Tuesday, January 16, 2018 - 19:18.
Getting Started
I've used CDN for Bootstrap, Angular JS and UI-Router so you need internet connection for them to work.Creating our Database
First, we're gonna create our MySQL Database where we get our data. 1. Open phpMyAdmin. 2. Click databases, create a database and name it as angular. 3. After creating a database, click the SQL and paste the below codes. See image below for detailed instruction.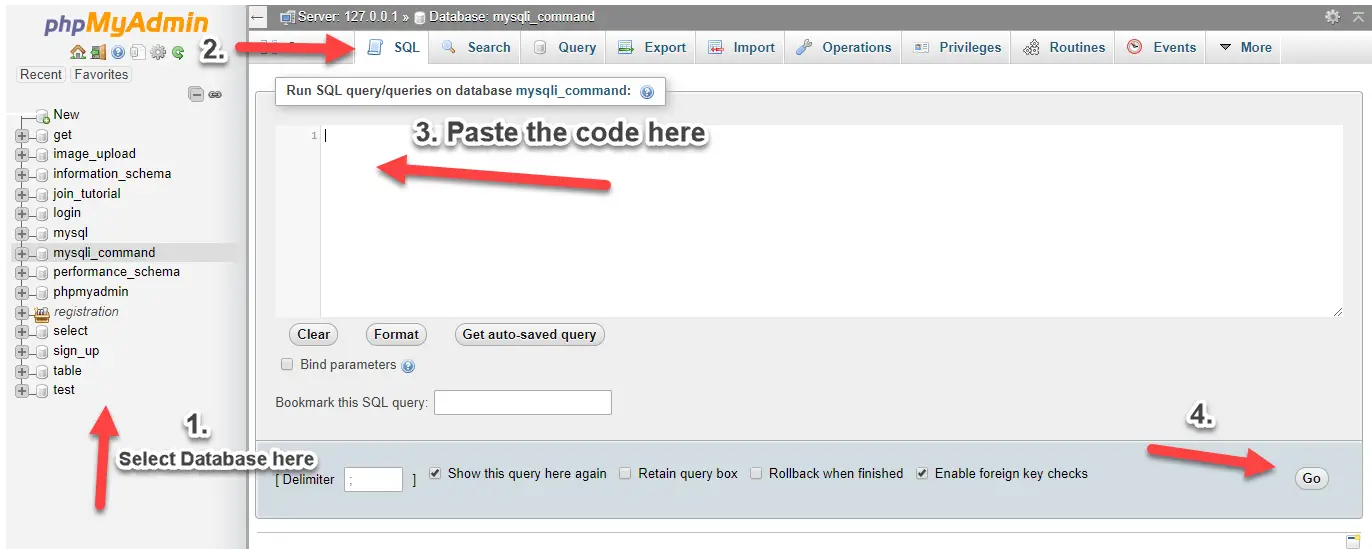
index.html
This is the main view of our app.- <!DOCTYPE html>
- <html ng-app="app">
- <head>
- <meta charset="utf-8">
- <link href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" rel="stylesheet">
- <style type="text/css">
- .sug-list{
- background-color: #4CAF50;
- border: 1px solid #FFFFFF;
- color: #FFFFFF;
- padding: 10px 24px;
- cursor: pointer;
- width: 100%;
- display: block;
- margin-top:1px;
- }
- .sug-list:not(:last-child) {
- border-bottom: none;
- }
- .sug-list a {
- text-decoration:none;
- color: #FFFFFF;
- }
- .no-sug{
- background-color: #4CAF50;
- border: 1px solid #FFFFFF;
- color: #FFFFFF;
- padding: 10px 24px;
- width: 100%;
- display: block;
- margin-top:1px;
- }
- </style>
- </head>
- <body>
- <div class="container">
- </div>
- </body>
- </html>
app.js
This is the main angular js script of our app.- var app = angular.module('app', ['ui.router']);
- app.config(function($stateProvider, $urlRouterProvider) {
- $urlRouterProvider.otherwise('/home');
- $stateProvider
- .state('home', {
- url: '/home',
- templateUrl: 'home.html',
- controller: 'homeCtrl'
- })
- .state('member', {
- url: '/member/{member:json}',
- params: {member: null},
- templateUrl: 'member.html',
- controller: 'memberCtrl'
- })
- });
- app.controller('homeCtrl', function($scope, $http) {
- $scope.hasMember = false;
- $scope.noMember = false;
- $scope.fetch = function(){
- $http.get('fetch.php').success(function(data){
- $scope.memberLists = data;
- });
- }
- $scope.search = function(){
- if($scope.keyword == ''){
- $scope.hasMember = false;
- $scope.noMember = false;
- }
- else{
- $http.post('search.php', {
- 'keyword': $scope.keyword
- })
- .success(function(data){
- if(data.length > 0){
- $scope.members = data;
- $scope.hasMember = true;
- $scope.noMember = false;
- }
- else{
- $scope.noMember = true;
- $scope.hasMember = false;
- }
- });
- }
- }
- });
- app.controller('memberCtrl', function($scope, $stateParams) {
- $scope.details = $stateParams.member;
- });
fetch.php
This is our PHP api that fetches data from our MySQL database.- <?php
- $conn = new mysqli("localhost", "root", "", "angular");
- $sql = "SELECT * FROM members";
- $query = $conn->query($sql);
- while($row=$query->fetch_array()){
- $out[] = $row;
- }
- ?>
home.html
As per our ui-router this is treated as our index view.- <div class="row">
- <div class="col-sm-6 col-sm-offset-1" ng-init="fetch()">
- <table class="table table-bordered table-striped">
- <thead>
- <tr>
- </tr>
- </thead>
- <tbody>
- <tr ng-repeat="memberList in memberLists">
- </tr>
- </tbody>
- </table>
- </div>
- <div class="col-sm-4">
- <input type="text" class="form-control" ng-model="keyword" ng-keyup="search()" placeholder="Search for Firstname, Lastname or Address">
- <div ng-repeat="member in members" ng-show="hasMember" class="sug-list">
- </div>
- <div ng-show="noMember" class="no-sug">
- No Suggestion Found
- </div>
- </div>
- </div>
search.php
This is our PHP api that searches our MySQL database and returning the search match.- <?php
- $conn = new mysqli("localhost", "root", "", "angular");
- $keyword = $data->keyword;
- $out = '';
- }
- else{
- $sql = "SELECT * FROM members WHERE firstname LIKE '%$keyword%' OR lastname LIKE '%$keyword%' OR address LIKE '%$keyword%'";
- $query = $conn->query($sql);
- while($row=$query->fetch_array()){
- $out[] = $row;
- }
- }
- ?>
member.html
Lastly, this is where we view the details of our clicked search result.- <div class="col-sm-4 col-sm-offset-4">
Add new comment
- 93 views