AngularJS Login with PHP/MySQLi
Submitted by nurhodelta_17 on Friday, January 5, 2018 - 18:39.
Getting Started
I've used CDN for Bootstrap, Angular JS and Angular Route so you need internet connection for them to work.Creating our Database
First, we are going to create our database and insert sample users to test our app. 1. Open phpMyAdmin. 2. Click databases, create a database and name it as angular. 3. After creating a database, click the SQL and paste the below codes. See image below for detailed instruction.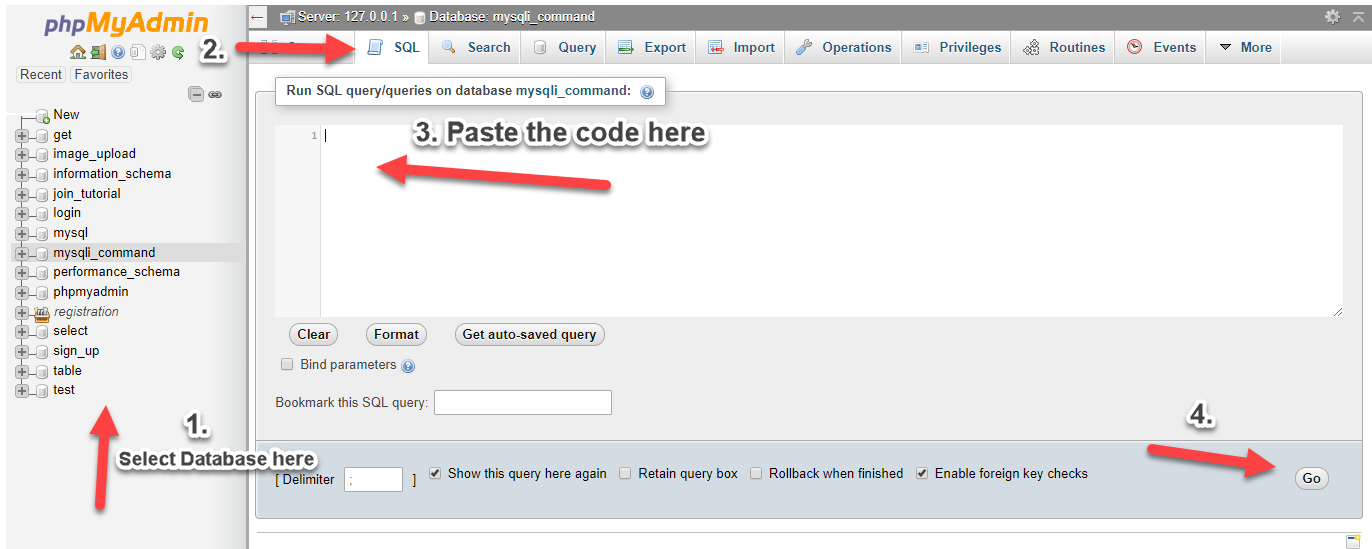
index.html
This is the main page of our app.login.html
This contains our login form.- <div class="col-md-4 col-md-offset-4">
- <div class="login-panel panel panel-primary">
- <div class="panel-heading">
- </h3>
- </div>
- <div class="panel-body">
- <form role="form" name="logform">
- <fieldset>
- <div class="form-group">
- <input class="form-control" placeholder="Username" type="text" autofocus ng-model="user.username" required>
- </div>
- <div class="form-group">
- <input class="form-control" placeholder="Password" type="password" ng-model="user.password" required>
- </div>
- </fieldset>
- </form>
- </div>
- </div>
- <div class="alert alert-danger text-center" ng-show="errorLogin">
- {{ errorMsg }}
- </div>
- <div class="alert alert-success text-center" ng-show="successLogin">
- {{ successMsg }}
- </div>
- </div>
angular.js
This is the main angular js script.- var app = angular.module('app', ['ngRoute']);
- app.config(function($routeProvider){
- $routeProvider
- .when('/', {
- templateUrl: 'login.html',
- controller: 'loginCtrl'
- })
- .when('/home', {
- templateUrl: 'home.html'
- })
- .otherwise({
- redirectTo: '/'
- });
- });
loginCtrl.js
This is the controller for our login.html.- 'use strict';
- app.controller('loginCtrl', function($scope, loginService){
- $scope.errorLogin = false;
- $scope.successLogin = false;
- $scope.login = function(user){
- loginService.login(user, $scope);
- }
- $scope.clearMsg = function(){
- $scope.errorLogin = false;
- $scope.successLogin = false;
- }
- });
loginService.js
This is our service that sends post request to our PHP API for login.- 'use strict';
- app.factory('loginService', function($http){
- return{
- login: function(user, $scope){
- var validate = $http.post('login.php', user);
- validate.then(function(response){
- if(response.data.error == true){
- $scope.successLogin = false;
- $scope.errorLogin = true;
- $scope.errorMsg = response.data.message;
- }
- else{
- $scope.errorLogin = false;
- $scope.successLogin = true;
- $scope.successMsg = response.data.message;
- }
- });
- }
- }
- });
login.php
Lastly, this is our PHP api/code in checking our login.- <?php
- $conn = new mysqli("localhost", "root", "", "angular");
- if ($conn->connect_error) {
- }
- $username = $user->username;
- $password = $user->password;
- $sql = "SELECT * FROM members WHERE username='$username' AND password='$password'";
- $query = $conn->query($sql);
- if($query->num_rows>0){
- $out['message'] = 'Login Successful';
- }
- else{
- $out['error'] = true;
- $out['message'] = 'Invalid Login';
- }
- ?>
Add new comment
- 183 views