Installing ReactJS/Getting Started with ReactJS
Submitted by nurhodelta_17 on Monday, December 18, 2017 - 22:13.
Getting Started
First, we're gonna be needing Node.js to handle the install of our packages. If you don't have Node.js installed, you can use this link to download Node.js.Creating our App Folder
Next, create root folder for our ReactJS app. In your Desktop, create a new folder and name it as reactApp. Open command prompt and navigate to your root folder.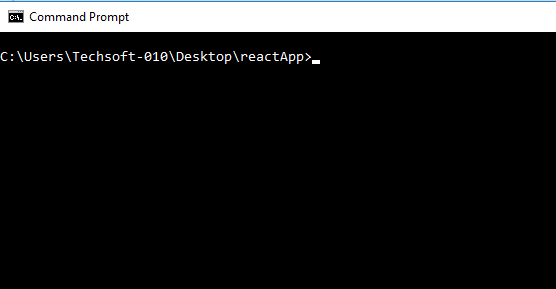
npm init
Installing the Dependencies for ReactJS
Next, we're gonna install babel plugins, webpack and react itself. Open command prompt, navigate to our reactApp folder and type the ff:npm install -g babel
npm install -g babel-cli
npm install webpack --save
npm install webpack-dev-server --save
npm install react --save
npm install react-dom --save
npm install babel-core
npm install babel-loader
npm install babel-preset-react
npm install babel-preset-es2015
Creating our Files
In our root folder, reactApp, create the ff files: webpack.config.js- var config = {
- entry: './main.js',
- output: {
- path:'/',
- filename: 'index.js',
- },
- devServer: {
- inline: true,
- port: 8080
- },
- module: {
- loaders: [
- {
- test: /\.jsx?$/,
- exclude: /node_modules/,
- loader: 'babel-loader',
- query: {
- presets: ['es2015', 'react']
- }
- }
- ]
- }
- }
- module.exports = config;
App.jsx
import React from 'react';
class App extends React.Component {
render() {
return (
Hello World!!!
);
}
}
export default App;
main.js
- import React from 'react';
- import ReactDOM from 'react-dom';
- import App from './App.jsx';
- ReactDOM.render(<App />, document.getElementById('app'));
Preparing our Server
1. Open package.json and removing the ff lines inside "scripts" object. "test" "echo \"Error: no test specified\" && exit 1" 2. Replace the removed line with the ff: "start": "webpack-dev-server --hot" 3. Install webpack-dev-server by opening command prompt, navigating to our reactApp folder and typing the ff:npm install webpack-dev-server -g
Running our Server
Lastly, we run our server by opening command prompt, navigate to our reactApp folder and type:npm start
It will then give you where the project is running which is usually at http://localhost:8080/.
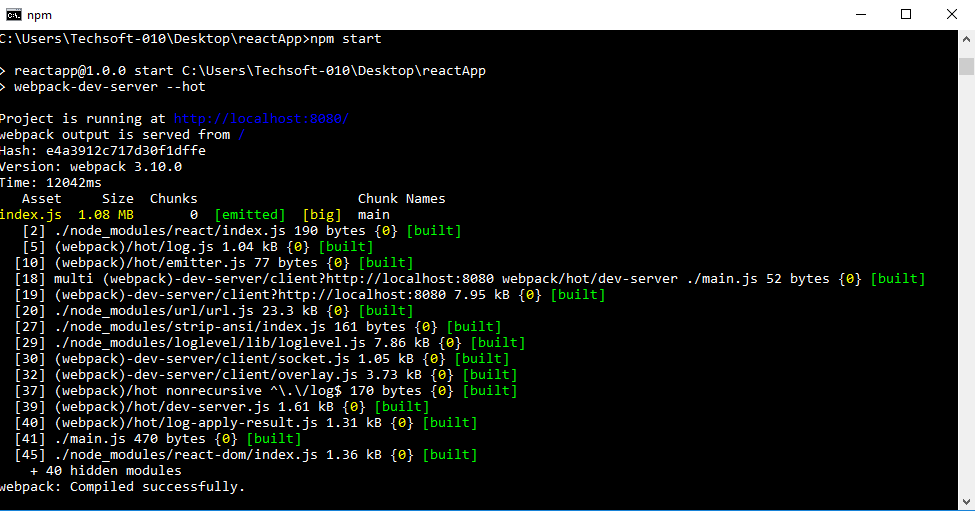