Facebook Style Alert Confirm Box Using CSS and jQuery
Submitted by janobe on Monday, June 27, 2016 - 08:04.
This time, I will show you how to make a Facebook Style Alert Confirm Box using CSS and jQuery. This method is usually used for confirming or deleting something in a website. A dialog box will then appear to inform the user whether he decides to delete or not the thread that he have created.
Use the following jQuery Plugins as follows:
3. Create a design of the box with a "x" link.
For all students who need a programmer for your thesis system or anyone who needs a source code in any programming languages. You can contact me @ :
Email – [email protected]
Mobile No. – 09305235027 – TNT
- <script type="text/javascript" src="jquery/jquery.min.js"></script>
- <script type="text/javascript" src="jquery/jquery.alerts.js"></script>
Let's begin:
1. Create a landing page and name it "index.php". 2. Create a CSS style for the confirm box.- <style type="text/css">
- /* pop up id */
- #popup_container {
- margin: 1px;
- padding: 1px;
- height: 110px;
- background: #f0ecec;
- border:solid 1px #dedede;
- border-bottom: solid 1px #456FA5;
- color: #000;
- }
- /* pop message */
- #popup_message {
- padding-left: 16px;
- }
- /* pop up panel id */
- #popup_panel {
- text-align: left;
- padding-left:19px;
- padding-bottom: 0;
- }
- /* for the button in the pop up message */
- input{
- background-color:#554df7;
- padding:4px;
- color:#FFFFFF;
- margin-top:21px;
- margin-right:11px;
- }
- #wrap{
- border:1px solid #dedede;
- height:150px;
- width:200px;
- }
- /* for the image in the box */
- #wrap> img {
- margin-top: -5px;
- margin-left: 1px;
- height: 90%;
- width: 99%;
- }
- #wrap> #close a{
- float:right;
- width:10px;
- height:18px;
- text-decoration: none;
- }
- </style>
4. Create a script for calling the method of the alert box.
- <script type="text/javascript">
- $(document).ready( function() {
- $("#del").click( function()
- {
- /* confirm messaage */
- jConfirm('Are you sure you want to hide this thread?', '',
- function(f) {
- if(f==true)
- {
- $("#wrap").fadeOut(1200);
- }
- });
- });
- });
- </script>
Output :
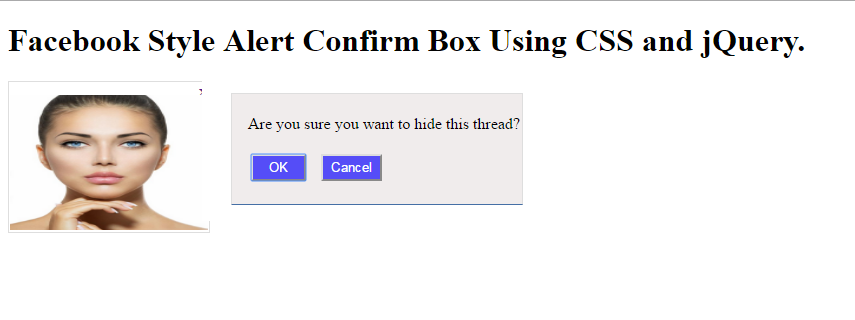
Add new comment
- 75 views