JSplitPane Component in Java
Submitted by donbermoy on Saturday, November 22, 2014 - 23:34.
This is a tutorial in which we will going to create a program that will have a JSplitPane Component in Java. A JSplitPane is used to let the user divides the two components that can also be resized by the user.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of jSpliPaneComponent.java.
2. Import the following packages:
3. Initialize your variable in your Main, variable frame for JFrame, variable text for JTextField, and variable label for JLabel. The text and label components will then be put inside the JSplitPane.
The JSplitPane above is aligned from left to right using the horizontal alignment of the syntax HORIZONTAL_SPLIT. When we have to align them from top to buttom, we will use the VERTICAL_SPLIT. Take note that the text as JTextField and label as the JLabel was put inside the JSplitPane to divide the components into two.
5. Now, add the JSplitPane variable to the frame using the default BorderLayout of Center position of the getContentPane method. Lastly, set the size, visibility, and the close operation of the frame. Have this code below:
Output:
Here's the full code of this tutorial:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import java.awt.*;//used to access the Dimension class
- import javax.swing.*; //used to access the JFrame, JLabel, JTextField, and JSplitPane component
To have the size of the label and text, we will used the setMinimumSize method with the Dimension class having its width and height.
4. Now, we will use the JSplitPane to divide the label and text into two panes. Have this code below:
- frame.getContentPane().add(sp, "Center");
- frame.setSize(450, 200);
- frame.setVisible(true);
- frame.setDefaultCloseOperation(frame.EXIT_ON_CLOSE);
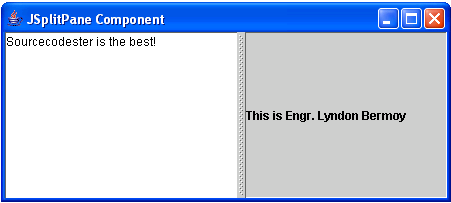
- import java.awt.*;//used to access the Dimension class
- import javax.swing.*; //used to access the JFrame, JLabel, JTextField, and JSplitPane component
- public class jSplitPaneComponent {
- frame.getContentPane().add(sp, "Center");
- frame.setSize(450, 200);
- frame.setVisible(true);
- frame.setDefaultCloseOperation(frame.EXIT_ON_CLOSE);
- }
- }
Add new comment
- 42 views