JPasswordField Component in Java
Submitted by donbermoy on Friday, November 21, 2014 - 09:08.
This is a tutorial in which we will going to create a program that has the JPasswordField Component using Java. The JPasswordField provides text fields for password entry and it does not show the characters that the user types. It will only display "*" for the password character.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of jPasswordFieldComponent.java.
2. Import the javax.swing.* package so that we can access the JFrame, JLabel, JPasswordField, and Box class.
3. Initialize your variable in your Main, variable frame for creating JFrame, and variable row for Box class.
A Box is container that is lightweight component that is used in the BoxLayout as layout manager and will create a single row or column. We have created a Box with its method createHorizontalBox to create a single row because it is horizontal.
4. Now, we will add the components JLabel and JPasswordField to the Box. We will code first to add the JLabel and then next is for the JPasswordField so that the label will be first on the column followed by the password field.
5. Now, add the Box variable to the frame using the default BorderLayout of north position of the getContentPane method. Lastly, set the size, visibility, and the close operation of the frame. Have this code below:
Output:
Here's the full code of this tutorial:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import javax.swing.*; //used to access JFrame, JLabel, JPasswordField, and Box class
- frame.getContentPane().add(row, "North");
- frame.setSize(300, 100);
- frame.setVisible(true);
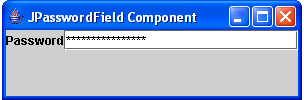
- import javax.swing.*; //used to access JFrame, JLabel, JPasswordField, and Box class
- public class jPasswordFieldComponent {
- frame.getContentPane().add(row, "North");
- frame.setSize(300, 100);
- frame.setVisible(true);
- }
- }
Add new comment
- 50 views