Open a Printer Dialog in Java
Submitted by donbermoy on Monday, November 10, 2014 - 19:30.
This tutorial will teach you how to create a program that will open a printer dialog in Java. The printer dialog helps you to perform printing of documents.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of printDialog.java.
2. Import java.awt.print package. Hence we will use the print class of AWT package and this will access the PageFormat and PrinterJob in the print class.
3. Initialize your variables in your Main, variable printer used for PrinterJob method and pFormat for PageFormat method.
4. To open the printer dialog, have the syntax below:
5. Create a try and catch method. In the try method, this will print the pages with the default format and in catch, this will catch the error during printing.
Output:
Here's the full source code of this tutorial:
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:donbermoy@engineer.com
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import java.awt.print.*; // this is used to access the PageFormat and PrinterJob in the print class
- PrinterJob printer = PrinterJob.getPrinterJob(); // this method calls to setup a job for printing pages
- PageFormat pFormat = printer.defaultPage(); // the page is set to default size format and orientation
- printer.printDialog(); // show the print dialog
- try {
- printer.print(); //if clicking ok in the print dialog, this will print the pages with the default format
- }
- }
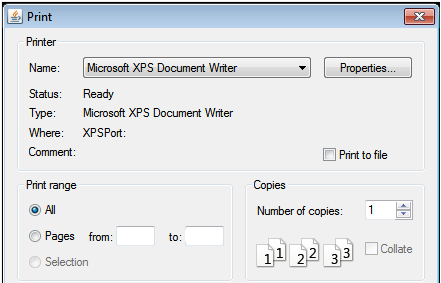
- import java.awt.print.*; // this is used to access the PageFormat and PrinterJob in the print class
- public class printDialog {
- PrinterJob printer = PrinterJob.getPrinterJob(); // this method calls to setup a job for printing pages
- PageFormat pFormat = printer.defaultPage(); // the page is set to default size format and orientation
- printer.printDialog(); // show the print dialog
- try {
- printer.print(); //if clicking ok in the print dialog, this will print the pages with the default format
- }
- }
- }
- }
Add new comment
- 684 views