Activity's lifecycle in Android Application.
Submitted by pavel7_7_7 on Tuesday, September 9, 2014 - 10:16.
In this tutorial you can find information about lifecycle of an Android Activity.
Almost all the Activities interact with the user. So, an activity creates a window where you can place any of the user interface elements. Understanding of the Activity's lifecycle is a necessary thing for any Android developer.
The whole lifecycle of the Activity can be presented on the following schema:
This schema shows in a good way what happens with the activity during it's lifecycle.
The most important thing about activity's lifecycle is the fact that Android developer can manage the processes that happens during activity's life. We will create a demo application to see how does the activity works. For this scope, please create new project with blank activity. We will not modify the layout files and simply will add GUI components in the activity class:
The GUI Elements used in this demo application are:
The first thing that happens with the activity is it's creation.
The initialization of all GUI elements is performed in the
method. You can add any modification to this method only after you call the same method from the super class:
After this we can add our code:
The action listener is added to the close button.It will call the finish method for this activity.
After activity is created, onStart() method is called:
After onStart() method onResume() method is called:
Note an important thing that you always need to call method of the super class to avoid any kinds of errors.
Now the activity is running and you can interact with the user. The interaction with the user in this program is presented by close button.
This button closes activity.
Let's take a look what happens when the activity is closed.
Firstly, onPause() method is closed.We will add the message as in the previous cases:
After this activity is stopped:
There is a possibility to restart Activity even if is stopped.This step is controlled by onRestart() method:
The onDestroy() method kills the activity. You have to add any code before calling the super class onDestroy() method:
Now you know the basic things about managing Android Activity's lifecycle. This mechanism of overriding can be used for different purposes. For example, you can save data of application when it's destroyed to a database and restore in onCreate(Bundle savedInstanceState) method.
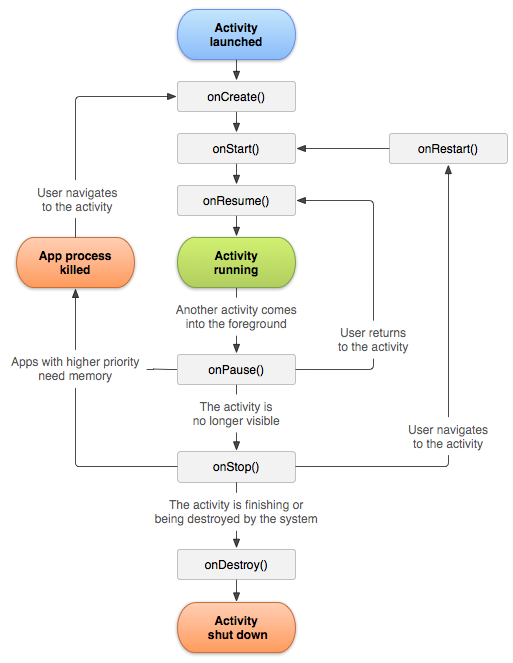
- <?xml version="1.0" encoding="utf-8"?>
- <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
- android:orientation="vertical"
- android:layout_width="fill_parent"
- android:layout_height="fill_parent"
- >
- <TextView
- android:layout_width="fill_parent"
- android:layout_height="wrap_content"
- android:text="@string/hello"
- />
- </LinearLayout>
- LinearLayout linLayout;
- Button closeBtn;
- public void onCreate(Bundle savedInstanceState)
- super.onCreate(savedInstanceState);
- super.onCreate(savedInstanceState);
- linLayout = new LinearLayout(this);
- setContentView(linLayout);
- linLayout.setOrientation(LinearLayout.VERTICAL);
- closeBtn.setText("CLOSE");
- linLayout.addView(closeBtn);
- closeBtn.setOnClickListener(new OnClickListener() {
- @Override
- ((Activity) v.getContext()).finish();
- }
- });
- Toast.makeText(this, "Demo Activity created!", Toast.LENGTH_SHORT).show();
- @Override
- public void onStart() {
- super.onStart();
- Toast.makeText(this, "Demo Activity Started!", Toast.LENGTH_SHORT).show();
- }
- @Override
- public void onResume() {
- super.onResume();
- Toast.makeText(this, "Demo Activity resumed!", Toast.LENGTH_SHORT).show();
- }
- @Override
- public void onPause() {
- super.onPause();
- Toast.makeText(this, "Demo Activity paused!", Toast.LENGTH_SHORT).show();
- }
- @Override
- public void onStop() {
- super.onStop();
- Toast.makeText(this, "Demo Activity stoped!", Toast.LENGTH_SHORT).show();
- }
- @Override
- public void onRestart() {
- super.onRestart();
- Toast.makeText(this, "Demo Activity restarted!", Toast.LENGTH_SHORT).show();
- }
- @Override
- public void onDestroy() {
- Toast.makeText(this, "Demo Activity destroyed!", Toast.LENGTH_SHORT).show();
- super.onDestroy();
- }