How to Generate a Random Number in Java
Submitted by donbermoy on Tuesday, May 27, 2014 - 16:24.
In this tutorial, we will going to create a program that generates a random number using Java. We will just use the Math.random() function which returns a random number between 0.0 and 1.0
Now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of randomNumber.java.
2. Import javax.swing package. Hence we will use the JOptionPane.showMessageDialog than the System.out.print.
3. Initialize your variable in your Main, i have here variable number as an integer that will be equal to the Math.random() function. Take note: Math.random() returns a random number between 0.0 and 1.0, including 0.0 but not including 1.0 which means this function covers to be in double or decimal data type in nature. I also put the JOptionPane.showMessageDialog for displaying and generating the random number output.
Now, we will create again a random number by multiplying Math.random() result with a number will give a range of random number between, for instance 0.0 to 50 as shown in my code below.
The example below creates random number between 100.0 and 300.0.
Now, we will create an integer random number from 100 to 200.
Output:
Full source code:
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import javax.swing.*;
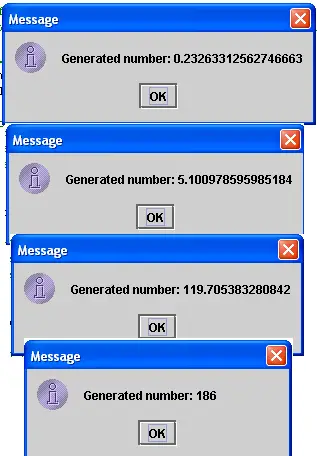
- import javax.swing.*;
- public class randomNumber
- {
- {
- }
- }