Palindrome in Java GUI
Submitted by donbermoy on Wednesday, May 7, 2014 - 16:28.
We all know that palindrome is a word or phrase that reads the same backward as forward. Here in this tutorial, we will create a program that can determine if the word inputted is a palindrome or not.
Now, let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of palindrome.java.
2. Import javax.swing package. Hence we will use a GUI (Graphical User Interface) here like the inputting the word.
3. Before coding in the Main, create first a function named isPalindrome as Boolean that has variable word as string as parameter of it.
4. Initialized your variable in your Main, variable Str as string and make it as an input.
5. Lastly, display the output of the program whether the word is a palindrome or not.
Take note that isPalindrome(Str) syntax triggers to access the boolean function that will validate the inputted word of variable Str.
Output:
Here's the full code of this tutorial:
Hope this helps! :)
Best Regards,
Engr. Lyndon R. Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
- import javax.swing.*;
- int left = 0; // index of leftmost unchecked char
- int right = word.length() -1; // index of the rightmost
- while (left < right) { // continue until they reach center
- if (word.charAt(left) != word.charAt(right)) {
- return false; // if chars are different, finished
- }
- left++; // move left index toward the center
- right--; // move right index toward the center
- }
- return true; // if finished, all chars were same
- }
- String Str;
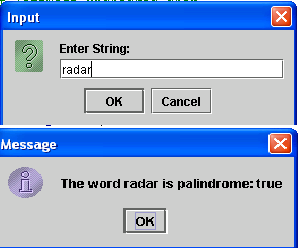
- import javax.swing.*;
- public class palindrome {
- String Str;
- }
- int left = 0;
- int right = word.length() -1;
- while (left < right) {
- if (word.charAt(left) != word.charAt(right)) {
- return false;
- }
- left++;
- right--;
- }
- return true;
- }
- }
Add new comment
- 1112 views