Creating User Account Information in Java - Updating and Deleting Records to Database
Submitted by donbermoy on Friday, May 2, 2014 - 11:23.
This is my final continuation of my other two tutorials: Creating User Account Information in Java - Adding Records to Database and Creating User Account Information in Java - Searching Records to Database. Now, we don't have to modify our code for that. Only add the following code below to your UserSettings.java.
1. This is for the initialization of variables to be used as buttons and labels. Just use JButton to have a button in your form. Here we just add Update, Delete, and Exit Button from the previous tutorial.
3. Lastly, in the actionEvents when clicking this 3 buttons put this code below.
The syntax for updating a record here is the:
Here is the full code of this tutorial:
Best Regards,
Engr. Lyndon R. Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
2. Now in your constructor UserSettings() , add the 3 buttons for update, delete, and exit.
- public UserSettings() {
- btnUpdate.setBounds(80,190,75,35);
- pane.add(btnUpdate);
- btnUpdate.addActionListener(this);
- btnDelete.setBounds(155,190,75,35);
- pane.add(btnDelete);
- btnDelete.addActionListener(this);
- btnExit.setBounds(130,260,75,35);
- pane.add(btnExit);
- }
- if(source == btnUpdate){
- try{
- if (!uname.equals("") && !pass.equals("")&& !name1.equals("") && !name2.equals("")) {
- st= cn.createStatement();
- PreparedStatement ps = cn.prepareStatement("UPDATE Login SET password = '" + txtPass.getText() + "',name1 = '" + txtName1.getText() + "',name2= '" + txtName2.getText()+ "'WHERE username = '" + txtUser.getText() + "'");
- ps.executeUpdate();
- JOptionPane.showMessageDialog(null,"Account has been successfully updated.","Payroll System: User settings",JOptionPane.INFORMATION_MESSAGE);
- txtUser.requestFocus(true);
- clear();
- st.close();
- }
- else{
- JOptionPane.showMessageDialog(null,"Please Fill Up The Empty Fields","Warning",JOptionPane.WARNING_MESSAGE);
- }
- JOptionPane.showMessageDialog(null,"Unable to update!.","Payroll System: User settings",JOptionPane.ERROR_MESSAGE);
- }
- }
- if(source==btnDelete){
- try{
- PreparedStatement ps = cn.prepareStatement("DELETE FROM Login WHERE username ='"+ txtUser.getText() + "'");
- ps.executeUpdate();
- JOptionPane.showMessageDialog(null,"Account has been successfully deleted.","Payroll System: User settings ",JOptionPane.INFORMATION_MESSAGE);
- txtUser.requestFocus(true);
- clear();
- st.close();
- JOptionPane.showMessageDialog(null,"Unable to delete!.","Payroll System: User settings",JOptionPane.ERROR_MESSAGE);}
- }if(source==btnExit){
- dispose();
- }
- }
PreparedStatement ps = cn.prepareStatement("UPDATE Login SET password = '" + txtPass.getText() + "',name1 = '" + txtName1.getText() + "',name2= '" + txtName2.getText()+ "'WHERE username = '" + txtUser.getText() + "'");
The syntax for deleting a record here is the: PreparedStatement ps = cn.prepareStatement("DELETE FROM Login WHERE username ='"+ txtUser.getText() + "'");
And lastly, the dispose() function is used for exiting or closing the form.
Output:
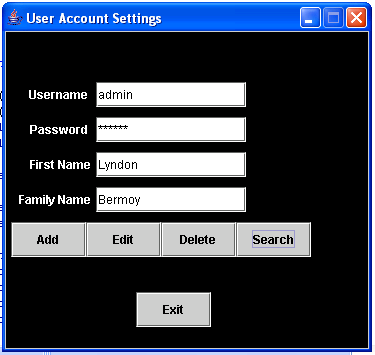
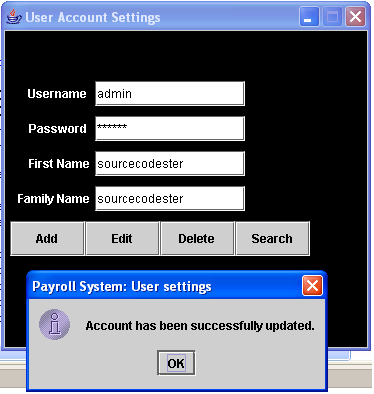
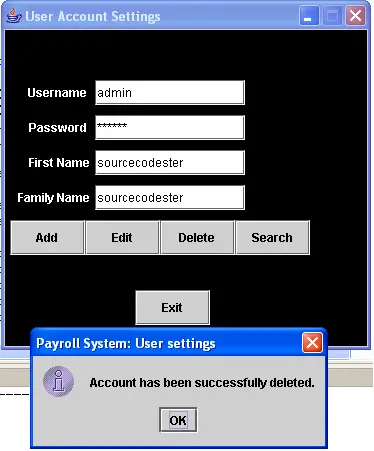
- import javax.swing.*;
- import java.awt.*;
- import java.awt.event.*;
- import java.sql.*;
- import java.lang.*;
- Connection cn;
- Statement st;
- public void clear(){
- txtUser.setText("");
- txtPass.setText("");
- txtName1.setText("");
- txtName2.setText("");
- }
- public UserSettings() {
- super("User Account Settings");
- pane.setLayout(null);
- lblUser.setBounds(5,50,80,25);
- pane.add(lblUser);
- txtUser.setBounds(90,50,150,25);
- pane.add(txtUser);
- lblPass.setBounds(5,85,80,25);
- pane.add(lblPass);
- txtPass.setBounds(90,85,150,25);
- txtPass.setEchoChar('*');
- pane.add(txtPass);
- lblName1.setBounds(5,120,80,25);
- pane.add(lblName1);
- txtName1.setBounds(90,120,150,25);
- pane.add(txtName1);
- lblName2.setBounds(5,155,80,25);
- pane.add(lblName2);
- txtName2.setBounds(90,155,150,25);
- pane.add(txtName2);
- btnNew.setBounds(5,190,75,35);
- pane.add(btnNew);
- btnNew.addActionListener(this);
- btnUpdate.setBounds(80,190,75,35);
- pane.add(btnUpdate);
- btnUpdate.addActionListener(this);
- btnDelete.setBounds(155,190,75,35);
- pane.add(btnDelete);
- btnDelete.addActionListener(this);
- btnSearch.setBounds(230,190,75,35);
- pane.add(btnSearch);
- btnSearch.addActionListener(this);
- btnExit.setBounds(130,260,75,35);
- pane.add(btnExit);
- btnExit.addActionListener(this);
- setContentPane(pane);
- try{
- Class.forName("sun.jdbc.odbc.JdbcOdbcDriver");
- e.printStackTrace();
- }
- e.printStackTrace();
- }
- }
- if(source == btnNew){
- try{
- if (!uname.equals("") && !pass.equals("")&& !name1.equals("") && !name2.equals("")) {
- st= cn.createStatement();
- ps=cn.prepareStatement("INSERT INTO Login" + " (username,password,name1,name2) " + " VALUES(?,?,?,?)");
- ps.setString(1,txtUser.getText());
- ps.setString(2,txtPass.getText());
- ps.setString(3,txtName1.getText());
- ps.setString(4,txtName2.getText());
- ps.executeUpdate();
- JOptionPane.showMessageDialog(null,"New account has been successfully added.","Payroll System: User settings",JOptionPane.INFORMATION_MESSAGE);
- txtUser.requestFocus(true);
- st.close();
- clear();
- }
- else{
- JOptionPane.showMessageDialog(null,"Please Fill Up The Empty Fields","Warning",JOptionPane.WARNING_MESSAGE);
- }
- sqlEx.printStackTrace();
- JOptionPane.showMessageDialog(null,"Unable to save!.","Payroll System: User settings",JOptionPane.ERROR_MESSAGE);}
- }
- if(source == btnSearch){
- try{
- int tmp= 0;
- clear();
- sUser = JOptionPane.showInputDialog(null,"Enter Username to search.","Payroll System: User settings",JOptionPane.QUESTION_MESSAGE);
- st= cn.createStatement();
- while(rs.next()){
- txtUser.setText(rs.getString(1));
- txtPass.setText(rs.getString(2));
- txtName1.setText(rs.getString(3));
- txtName2.setText(rs.getString(4));
- tmp=1;
- }
- st.close();
- if (tmp==0){
- JOptionPane.showMessageDialog(null,"No record found!!.","Payroll System: User settings",JOptionPane.INFORMATION_MESSAGE);
- }
- JOptionPane.showMessageDialog(null,"Unable to search!.","Payroll System: User settings",JOptionPane.ERROR_MESSAGE);
- }
- }
- if(source == btnUpdate){
- try{
- if (!uname.equals("") && !pass.equals("")&& !name1.equals("") && !name2.equals("")) {
- st= cn.createStatement();
- PreparedStatement ps = cn.prepareStatement("UPDATE Login SET password = '" + txtPass.getText() + "',name1 = '" + txtName1.getText() + "',name2= '" + txtName2.getText()+ "'WHERE username = '" + txtUser.getText() + "'");
- ps.executeUpdate();
- JOptionPane.showMessageDialog(null,"Account has been successfully updated.","Payroll System: User settings",JOptionPane.INFORMATION_MESSAGE);
- txtUser.requestFocus(true);
- clear();
- st.close();
- }
- else{
- JOptionPane.showMessageDialog(null,"Please Fill Up The Empty Fields","Warning",JOptionPane.WARNING_MESSAGE);
- }
- JOptionPane.showMessageDialog(null,"Unable to update!.","Payroll System: User settings",JOptionPane.ERROR_MESSAGE);
- }
- }
- if(source==btnDelete){
- try{
- PreparedStatement ps = cn.prepareStatement("DELETE FROM Login WHERE username ='"+ txtUser.getText() + "'");
- ps.executeUpdate();
- JOptionPane.showMessageDialog(null,"Account has been successfully deleted.","Payroll System: User settings ",JOptionPane.INFORMATION_MESSAGE);
- txtUser.requestFocus(true);
- clear();
- st.close();
- JOptionPane.showMessageDialog(null,"Unable to delete!.","Payroll System: User settings",JOptionPane.ERROR_MESSAGE);}
- }if(source==btnExit){
- dispose();
- }
- }
- // public void frameUser(){
- UserSettings panel = new UserSettings();
- panel.setSize(370,350);
- panel.setVisible(true);
- panel.setLocation(350,200);
- panel.setResizable(false);
- }
- }
Add new comment
- 293 views