How to Shuffle Deck of Cards in Terminal Console using Python
In this tutorial, we’ll learn how to program "How to Shuffle a Deck of Cards in the Terminal Console using Python." We’ll focus on accurately and correctly shuffling a deck of cards. The objective is to achieve a proper shuffle of the cards. I'll provide a sample program to demonstrate the coding process, making it easy to understand and implement. So, let’s get started with coding!
This topic is straightforward to understand. Just follow the instructions I provide, and you'll be able to complete it with ease. The program I'll show you demonstrates the correct way to shuffle a deck of cards. I'll also provide a simple and efficient method to achieve this effectively. So, let’s start coding!
Getting Started:
First you will have to download & install the Python IDLE's, here's the link for the Integrated Development And Learning Environment for Python https://www.python.org/downloads/.
Creating Main Function
This is the main function of the application. The following code will display a simple GUI in terminal console that will display program. To do this, simply copy and paste these blocks of code into the IDLE text editor.- import itertools, random
- deck = list(itertools.product(range(1,14),['Spade','Heart','Diamond','Club']))
- while True:
- print("\n================= Shuffle Deck of Cards =================\n\n")
- random.shuffle(deck)
- print("You got:")
- for i in range(5):
- print(deck[i][0], "of", deck[i][1])
- opt = input("\nDo you want to try again?(yes/no): ")
- if opt.lower() == 'yes':
- ret=False
- elif opt.lower() == 'no':
- ret=True
- print("Exiting program....")
- else:
- print("Please enter yes/no:")
- break
- if ret == False:
- continue
This program simulates a shuffled deck of playing cards and draws a hand of five cards for the user. It generates a standard 52-card deck using itertools.product, where each card is represented by a number (1–13) and a suit (Spade, Heart, Diamond, Club). The deck is then shuffled randomly, and the top five cards are displayed as the user’s hand. After each round, the user can choose to shuffle and draw again or exit the program.
Output:
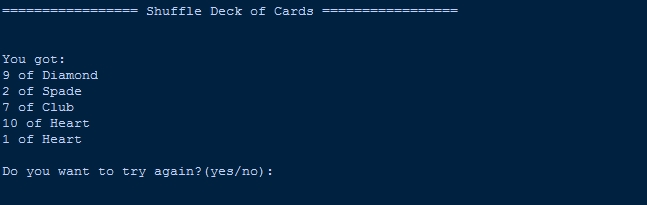
There you have it we successfully created How to Shuffle Deck of Cards in Terminal Console using Python. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for Python Language
Add new comment
- 17 views