How to Load Data From Database in a Combobox using C#
Submitted by joken on Tuesday, April 22, 2014 - 20:07.
This tutorial is a continuation of our last topic called “How to Create a Simple Record Navigation in C#”. At this time, I’m going to show you how to load data from Microsoft Access Database into a Combobox using C#. To start with this application, we need to open our project called “student_info” then we will apply the loading of data From Database in a combobox.
To do this, change the input field of “Course :” from textbox to combobox. And this will look like as shown below.
Next, we will create a new sub procedure called “loadDatatoCombobox” that will do the loading if data from database into combobox item. And here’s the following code:
At this time, when the form load, we will need to call the sub procedure that we create while ago to finally populate the combobox dynamically. To do this double click the form and add a single line of code and the form load function will look like as shown below.
And you can now test your application by pressing “F5” or click the start button. Then it should look like as shown below.
And here's e the new modified code used for this application.
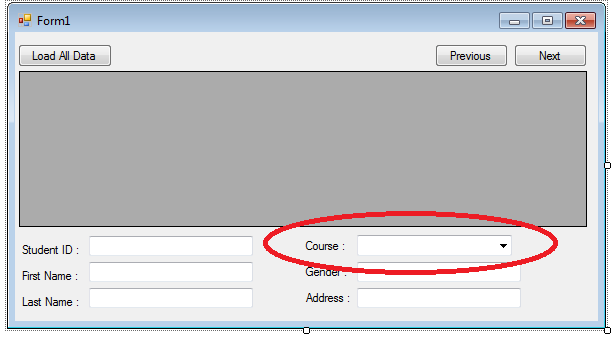
- //create a new datatable
- //create our SQL SELECT statement
- string sql = "Select course from tblstudent";
- try
- {
- conn.Open();
- //then we execute the SQL statement against the Connection using OleDBDataAdapter
- //we fill the result to dt which declared above as datatable
- da.Fill(table);
- //we add new entry to our datatable manually
- //becuase Select Course is not Available in the Database
- table.Rows.Add("Select Course");
- //set the combobox datasource
- comboBox1.DataSource = table;
- //choose the specific field to display
- comboBox1.DisplayMember = "course";
- comboBox1.ValueMember = "course";
- //set default selected value
- comboBox1.SelectedValue = "Select Course";
- }
- catch (Exception ex)
- {
- //this will display some error message if something
- //went wrong to our code above during execution
- MessageBox.Show(ex.ToString());
- }
- private void Form1_Load(object sender, EventArgs e)
- {
- loadDatatoCombobox();
- }
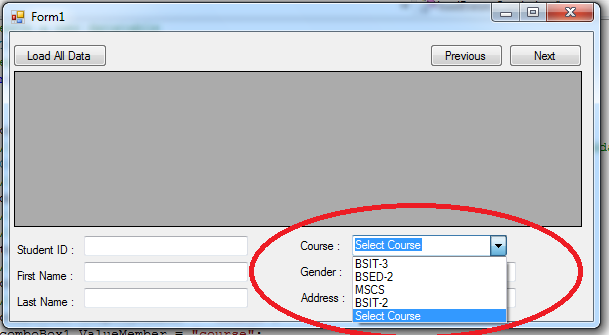
- using System;
- using System.Collections.Generic;
- using System.ComponentModel;
- using System.Data;
- using System.Drawing;
- using System.Linq;
- using System.Text;
- using System.Windows.Forms;
- using System.Data.OleDb;
- namespace student_info
- {
- public partial class Form1 : Form
- {
- //declare new variable named dt as New Datatable
- //this line of code used to connect to the server and locate the database (usermgt.mdb)
- static string connection = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source= " + Application.StartupPath + "/studentdb.mdb";
- int curRecord = 0;
- int totRecord = 0;
- public Form1()
- {
- InitializeComponent();
- }
- private void btnload_Click(object sender, EventArgs e)
- {
- //create a new datatable
- //create our SQL SELECT statement
- string sql = "Select * from tblstudent";
- //then we execute the SQL statement against the Connection using OleDBDataAdapter
- //we fill the result to dt which declared above as datatable
- da.Fill(dt);
- //set the curRecord to zero
- curRecord = 0;
- //get the total number of record available in the database and stored to totRecord Variable
- totRecord = dt.Rows.Count;
- //then we populate the datagridview by specifying the datasource equal to dt
- dataGridView1.DataSource = dt;
- }
- private void dataGridView1_CellClick(object sender, DataGridViewCellEventArgs e)
- {
- //it checks if the row index of the cell is greater than or equal to zero
- if (e.RowIndex >= 0)
- {
- //gets a collection that contains all the rows
- DataGridViewRow row = this.dataGridView1.Rows[e.RowIndex];
- //populate the textbox from specific value of the coordinates of column and row.
- txtid.Text = row.Cells[0].Value.ToString();
- txtfname.Text = row.Cells[1].Value.ToString();
- txtlname.Text = row.Cells[2].Value.ToString();
- comboBox1.Text = row.Cells[3].Value.ToString();
- txtgender.Text = row.Cells[4].Value.ToString();
- txtaddress.Text = row.Cells[5].Value.ToString();
- }
- }
- private void Form1_Load(object sender, EventArgs e)
- {
- loadDatatoCombobox();
- }
- private void loadDatatoCombobox()
- {
- //create a new datatable
- //create our SQL SELECT statement
- string sql = "Select course from tblstudent";
- try
- {
- conn.Open();
- //then we execute the SQL statement against the Connection using OleDBDataAdapter
- //we fill the result to dt which declared above as datatable
- da.Fill(table);
- //we add new entry to our datatable manually
- //becuase Select Course is not Available in the Database
- table.Rows.Add("Select Course");
- //set the combobox datasource
- comboBox1.DataSource = table;
- //choose the specific field to display
- comboBox1.DisplayMember = "course";
- comboBox1.ValueMember = "course";
- //set default selected value
- comboBox1.SelectedValue = "Select Course";
- }
- catch (Exception ex)
- {
- //this will display some error message if something
- //went wrong to our code above during execution
- MessageBox.Show(ex.ToString());
- }
- }
- private void Navigate()
- {
- txtid.Text = dt.Rows[curRecord][0].ToString();
- txtfname.Text = dt.Rows[curRecord][1].ToString();
- txtlname.Text = dt.Rows[curRecord][2].ToString();
- comboBox1.Text = dt.Rows[curRecord][3].ToString();
- txtgender.Text = dt.Rows[curRecord][4].ToString();
- txtaddress.Text = dt.Rows[curRecord][5].ToString();
- }
- private void btnprev_Click(object sender, EventArgs e)
- {
- //decrement the curRecord
- curRecord--;
- if (curRecord < 0)
- curRecord = totRecord - 1;
- //call subroutine
- Navigate();
- }
- private void btnnext_Click(object sender, EventArgs e)
- {
- curRecord++;
- if (curRecord >= totRecord)
- curRecord = 0;
- Navigate();
- }
- }
- }
Add new comment
- 2061 views