How to Load Data in the Listview Using C# and MySQL Database
Submitted by janobe on Thursday, January 3, 2019 - 14:36.
The Listview control is used to display a collection of items. This also provides many ways to arrange and display items with item text and an icon (optionally) is used to determine the type of item. So, now I’m going to teach you how to load data in the ListView using C# and MySQL Database. This is just a simple program but I’m pretty sure that this method will help you when you encounter problem of displaying set of data in the database and can be displayed in the Listview. So let’s get started.
Creating Database
Create a database and named it “salesdb”; Execute the following query to add the table with data in the database.- --
- -- Dumping data for table `tblsales`
- --
- (1, '2018-01-30', 'Cellphone', 1400),
- (2, '2018-02-28', 'Laptop', 800),
- (3, '2018-03-31', 'Desktop', 5052),
- (4, '2019-04-30', 'Ipod', 8030),
- (5, '2019-05-31', 'Tablet', 10000);
Creating Application
Step 1
Open Microsoft Visual Studio 2015 and create a new windows form application.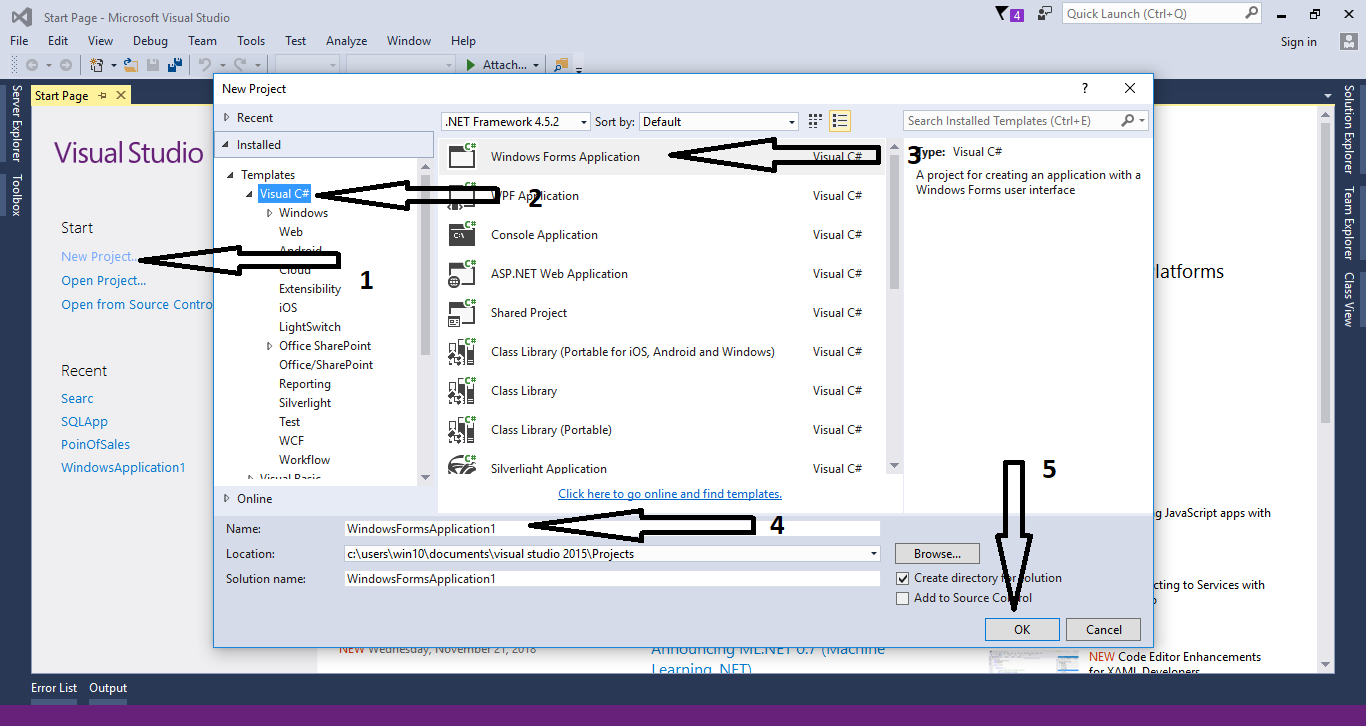
Step 2
Add a ListView inside the Form and do the Form just like this.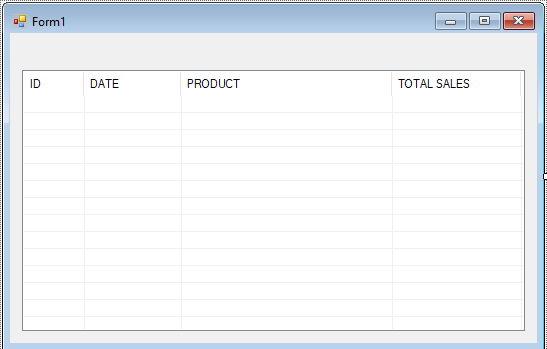
Step 3
Open the code editor and add a namespace to access MySQL Library.- using MySql.Data.MySqlClient;
Step 4
Create a method for retrieving data in the database to be displayed in the ListView.- private void LoadData()
- {
- MySqlConnection con = new MySqlConnection("server=localhost;user id=root;password=;database=salesdb;sslMode=none");
- MySqlCommand cmd;
- MySqlDataAdapter da;
- DataTable dt;
- try
- {
- con.Open();
- cmd.Connection = con;
- cmd.CommandText = "Select * FROM tblsales";
- da.SelectCommand = cmd;
- da.Fill(dt);
- for (int i = 0; i < dt.Rows.Count; i++)
- {
- DataRow dr = dt.Rows[i];
- listitem.SubItems.Add(dr["TRANSDATE"].ToString());
- listitem.SubItems.Add(dr["Product"].ToString());
- listitem.SubItems.Add(dr["TotalSales"].ToString());
- listView1.Items.Add(listitem);
- }
- }
- catch(Exception ex)
- {
- MessageBox.Show(ex.Message);
- }
- finally
- {
- con.Close();
- }
- }
Step 5
Call the method that you have created and put it inside the Load event handler
to perform in the first load of the Form
- private void Form1_Load(object sender, EventArgs e)
- {
- LoadData();
- }
Add new comment
- 4347 views