C# : Autocomplete in a Textbox with SQL Server
Submitted by janobe on Friday, July 22, 2016 - 09:15.
When you search data in the database, it is hard to search accurate data without a hard copy for your basis. So, in this tutorial, I will show you how to create an Autocomplete Textbox using C#.net and SQL Server 2005. This method has the ability to auto suggest and append the corresponding data on the Textbox when the first letter has an exact the same data in the database
Step 3. Go to the Solution Explorer, double-click the “View Code” to fire the code editor.
Step 4. Declare all the classes and variables that are needed.
For all students who need a programmer for your thesis system or anyone who needs a source code in any programming languages. You can contact me @ :
Email – [email protected]
Mobile No. – 09305235027 – tnt
Let's get started:
Step 1. Create a database and name it "dbplace". Step 2. Open Microsoft Visual Studio 2008 and create new Windows Form Application. Then, drag a TextBox on the form and it will look like this.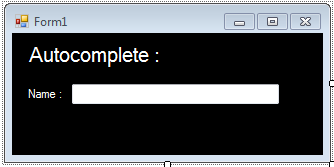
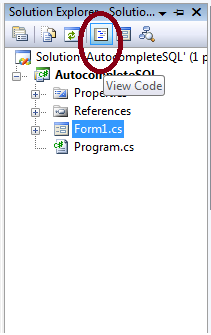
Note: Put using "System.Data.SqlClient;" above the namespace to access sql server library and to avoid errors when declaring the SQL classes.
Step 4. Go back to the design view, double click the Form and do the following code for creating an autocompete TextBox and retrieving data in the SQL database.
- private void Form1_Load(object sender, EventArgs e)
- {
- //Connection between SQL Server to C#
- con.ConnectionString = "Data Source=.\\SQLEXPRESS;Database=dbplace;trusted_connection=true;";
- //set a query for retrieving data in the database
- strquery = "Select p_Country from tblplace";
- //initialize new Sql commands
- //hold the data to be executed.
- cmd.Connection = con;
- cmd.CommandText = strquery;
- //initialize new Sql data adapter
- //fetching query in the database.
- da.SelectCommand = cmd;
- //initialize new datatable
- //refreshes the rows in specified range in the datasource.
- da.Fill(dt);
- textBox1.AutoCompleteCustomSource.Clear();
- foreach (DataRow r in dt.Rows)
- {
- //getting all rows in the specific field|Column
- var rw = r.Field<string>("p_Country");
- //Set the properties of a textbox to make it auto suggest and append.
- textBox1.AutoCompleteMode = AutoCompleteMode.SuggestAppend;
- textBox1.AutoCompleteSource = AutoCompleteSource.CustomSource;
- //adding all rows into the textbox
- textBox1.AutoCompleteCustomSource.Add(rw);
- }
- }