How to Display All Happy Numbers in Python
In this tutorial, we’ll learn how to program "How to Display All Happy Numbers in Python." We’ll focus on identifying and displaying all the possible happy numbers. The objective is to accurately verify and display the actual happy numbers. A sample program will be provided to demonstrate the coding process, making it straightforward and easy to implement. So, let’s get started!
This topic is straightforward to understand. Just follow the instructions I provide, and you'll be able to complete it with ease. The program I'll show you demonstrates the proper way to detect whether a number is a happy number. I'll also provide a simple and efficient method to achieve this effectively. So, let’s start coding!
Getting Started:
First you will have to download & install the Python IDLE's, here's the link for the Integrated Development And Learning Environment for Python https://www.python.org/downloads/.
Creating Main Function
This is the main function of the application. The following code will display a simple GUI in terminal console that will display program. To do this, simply copy and paste these blocks of code into the IDLE text editor.- def isHappyNumber(num):
- rem = sum = 0;
- while(num > 0):
- rem = num%10;
- sum = sum + (rem*rem);
- num = num//10;
- return sum;
- while True:
- print("\n================= Display All Happy Numbers =================\n\n")
- print("Display of happy numbers between 1 and 100: ");
- for i in range(1, 101):
- result = i;
- while(result != 1 and result != 4):
- result = isHappyNumber(result);
- if(result == 1):
- print(i),
- print(" "),
- opt = input("\nDo you want to try again?(yes/no): ")
- if opt.lower() == 'yes':
- ret=False
- elif opt.lower() == 'no':
- ret=True
- print("Exiting program....")
- else:
- print("Please enter yes/no:")
- break
- if ret == False:
- continue
This program identifies and displays all happy numbers between 1 and 100. A happy number is defined as a number that, when repeatedly replaced by the sum of the squares of its digits, eventually becomes 1. If the number results in a cycle involving 4, it is considered unhappy.
The program uses the isHappyNumber function to compute the sum of the squares of the digits of a given number. For each number between 1 and 100, it repeatedly applies this function until the result is either 1 (indicating the number is happy) or 4 (indicating it is unhappy). Happy numbers are printed as the result.
The program runs in a loop, allowing the user to repeat the process or exit based on their input.
Output:
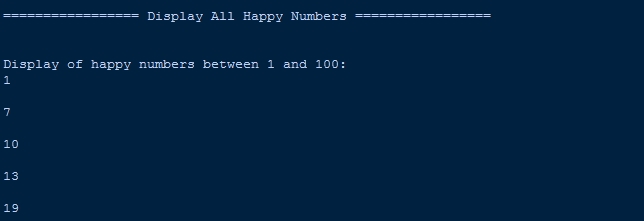
There you have it we successfully created How to Display All Happy Numbers in Python. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for Python Language
Add new comment
- 43 views