How to Check if the String is a Number in Python
In this tutorial, we’ll learn how to program "How to Check if a String is a Number in Python." We’ll focus on determining whether a given string represents a number (including floats). The objective is to accurately verify if the string qualifies as a number. A sample program will be provided to demonstrate the coding process, making it straightforward and easy to implement. So, let’s get started!
This topic is straightforward to understand. Just follow the instructions I provide, and you'll be able to complete it with ease. The program I'll show you demonstrates the proper way to detect whether a string is a number. I'll also provide a simple and efficient method to achieve this effectively. So, let’s start coding!
Getting Started:
First you will have to download & install the Python IDLE's, here's the link for the Integrated Development And Learning Environment for Python https://www.python.org/downloads/.
Creating Main Function
This is the main function of the application. The following code will display a simple GUI in terminal console that will display program. To do this, simply copy and paste these blocks of code into the IDLE text editor.- def isfloat(num):
- try:
- float(num)
- return True
- except ValueError:
- return False
- while True:
- print("\n================= Check if the String is a Float Number =================\n\n")
- num=input("Enter here: ")
- print(isfloat(num))
- opt = input("\nDo you want to try again?(yes/no): ")
- if opt.lower() == 'yes':
- ret=False
- elif opt.lower() == 'no':
- ret=True
- print("Exiting program....")
- else:
- print("Please enter yes/no:")
- break
- if ret == False:
- continue
This program checks if a given string can be converted to a float number. It defines a function isfloat(num) that attempts to convert the input string into a float and returns True if successful or False if a ValueError occurs. Inside a loop, the program prompts the user to enter a string, checks whether it is a float using the isfloat function, and prints the result. It then asks the user whether to try again or exit, repeating the process if "yes" is selected, and terminating otherwise.
Output:
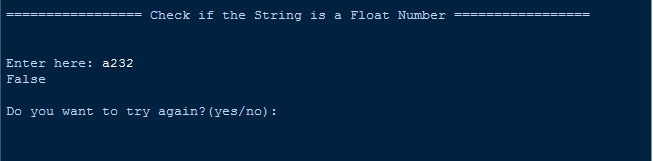
There you have it we successfully created How to Check if the String is a Number in Python. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for Python Language
Add new comment
- 59 views