How to Check if Password is Strong or Weak in Python
In this tutorial, we will program "How to Check if a Password is Strong or Weak in Python." We’ll focus on validating a password to determine whether it meets the requirements for strength. The objective is to safely check if the entered password passes the necessary qualifications. A sample program will be provided to demonstrate the coding process effectively.
This topic is straightforward to understand. Just follow the instructions I provide, and you'll be able to complete it yourself with ease. The program I'll show you demonstrates the correct way to validate a password to determine if it is strong or weak. I'll also provide a simple and efficient method to achieve this effectively. So, let’s start coding!
Getting Started:
First you will have to download & install the Python IDLE's, here's the link for the Integrated Development And Learning Environment for Python https://www.python.org/downloads/.
Creating Main Function
This is the main function of the application. The following code will display a simple GUI in terminal console that will display program. To do this, simply copy and paste these blocks of code into the IDLE text editor.- import re
- def password(v):
- if v == "\n" or v == " ":
- return "Password cannot be a newline or space!"
- if 9 <= len(v) <= 20:
- if re.search(r'(.)\1\1', v):
- return "Weak Password: Same character repeats three or more times in a row"
- if re.search(r'(..)(.*?)\1', v):
- return "Weak password: Same string pattern repetition"
- else:
- return "Strong Password!"
- else:
- return "Password length must be 9-20 characters!"
- def main():
- while True:
- print("\n================= Check if Password is Strong or Weak =================\n\n")
- mypass=input("Please enter your password here: ")
- print(password(mypass))
- opt = input("\nDo you want to try again?(yes/no): ")
- if opt.lower() == 'yes':
- ret=False
- elif opt.lower() == 'no':
- ret=True
- print("Exiting program....")
- else:
- print("Please enter yes/no:")
- break
- if ret == False:
- continue
- if __name__ == '__main__':
- main()
This program evaluates a user-entered password for strength, marking it as "Strong" if it meets the criteria of being 9 to 20 characters long, containing no repeated characters in a row or repeated patterns, and not consisting solely of newlines or spaces. If the password fails any of these checks, it is marked "Weak," with an explanation provided. The program runs continuously, prompting the user to try again until they choose to exit.
Output:
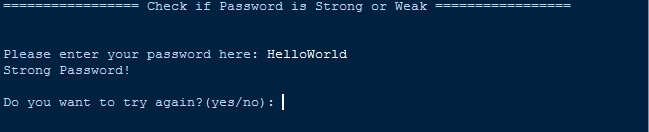
There you have it we successfully created How to Check if Password is Strong or Weak in Python. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for Python Language