How to Remove Duplicate Word from a String in Python
In this tutorial, we will learn how to program "How to Remove Duplicate Words from a String in Python." We will focus on identifying and removing any duplicate words from a string. The objective is to safely and accurately detect duplicate words within the string and remove them. A sample program will be provided to demonstrate the coding process.
This topic is very easy to understand. Just follow the instructions I provide, and you'll be able to do it yourself with ease. The program I’ll show you demonstrates the proper way to remove duplicate words from a string. I’ll also provide a simple and efficient method to achieve this. So, let's start coding!
Getting Started:
First you will have to download & install the Python IDLE's, here's the link for the Integrated Development And Learning Environment for Python https://www.python.org/downloads/.
Creating Main Function
This is the main function of the application. The following code will display a simple GUI in terminal console that will display program. To do this, simply copy and paste these blocks of code into the IDLE text editor.- import re
- def removeDupe(input):
- regex = r'\b(\w+)(?:\W+\1\b)+'
- return re.sub(regex, r'\1', input, flags=re.IGNORECASE)
- ret = False
- while True:
- print("\n================= Remove Duplicate Word from a String =================\n\n")
- my_str = "Lets enjoy the code code"
- print("Original String: ", my_str)
- print("\nRemoving Duplicate word: ", removeDupe(my_str))
- opt = input("\nDo you want to try again?(yes/no): ")
- if opt.lower() == 'yes':
- ret=False
- elif opt.lower() == 'no':
- ret=True
- print("Exiting program....")
- else:
- print("Please enter yes/no:")
- break
- if ret == False:
- continue
This Python script removes duplicate words from a given string using regular expressions. The removeDupe() function defines a regex pattern that matches consecutive duplicate words, ignoring case, and replaces them with just one instance of the word. The program demonstrates this with a predefined string "Lets enjoy the code code", prints both the original and the modified string, and then prompts the user to try again or exit. It continues in a loop until the user decides to stop.
Output:
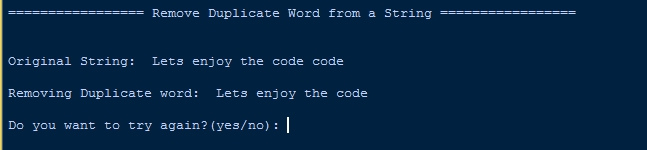
There you have it we successfully created How to Remove Duplicate Word from a String in Python. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for Python Language
Add new comment
- 57 views