How to Count Each Vowels in Terminal Console using Python
In this tutorial, we will program 'How to Count Each Vowel in a Terminal Console using Python.' We will learn how to count all the vowels in a given text. The objective is to determine the total number of each vowel present. I will provide a sample program to demonstrate the actual coding process in this tutorial.
This topic is very easy to understand. Just follow the instructions I provide, and you will be able to do it yourself with ease. The program I will show you covers the basics of programming for identifying vowels in each letter. I will do my best to provide you with a simple method for counting the total vowels. So, let's start coding.
Getting Started:
First you will have to download & install the Python IDLE's, here's the link for the Integrated Development And Learning Environment for Python https://www.python.org/downloads/.
Creating Main Function
This is the main function of the application. The following code will display a simple GUI in terminal console that will display program. To do this, simply copy and paste these blocks of code into the IDLE text editor.- class Vowels:
- def __init__(self, text):
- self.vowel = {'a': 0, 'e': 0, 'i': 0, 'o': 0, 'u': 0}
- self.text = list((text).lower())
- for letter in self.text:
- if letter in self.vowel.keys():
- self.vowel[letter] += 1
- def count_each_vowel(self):
- return self.vowel
- def count_vowels(self):
- return sum(self.vowel.values())
- ret = False
- while True:
- print("\n================= Count Each Vowels =================\n\n")
- text = input("Enter a string: ")
- x = Vowels(text)
- print(f"\nTotal Vowels : {x.count_vowels()} \n")
- print(f"--- Count of each vowel in give string ---")
- for key, value in x.vowel.items():
- print(f"{key} : {value}")
- opt = input("\nDo you want to try again?(yes/no): ")
- if opt.lower() == 'yes':
- ret=False
- elif opt.lower() == 'no':
- ret=True
- print("Exiting program....")
- else:
- print("Please enter yes/no:")
- break
- if ret == False:
- continue
This Python script defines a Vowels class that counts the number of occurrences of each vowel in a given string. The class has two methods: count_each_vowel, which returns a dictionary with the count of each vowel (a, e, i, o, u), and count_vowels, which returns the total number of vowels in the string.
The script runs in a loop, asking the user to input a string. It then creates an instance of the Vowels class, calculates and displays the total number of vowels and the count of each vowel in the string. The loop continues until the user decides to stop.
Output:
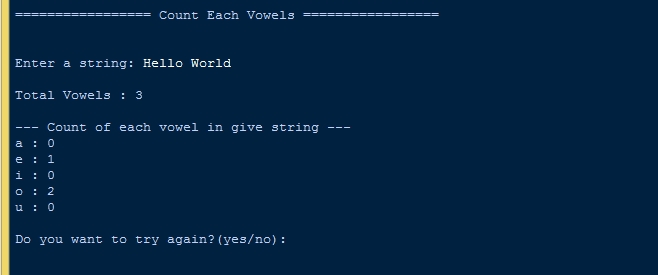
There you have it we successfully created How to Count Each Vowels in Terminal Console using Python. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for Python Language
Add new comment
- 116 views