Resizing the Uploaded Image in PHP using GD extension Tutorial
In this tutorial, you will learn how to resize the image file before saving it into a directory using PHP and GD Library. This process is really useful when you are developing a web application using PHP Language which has some features that include uploading Image files such as system users avatar, image/gallery thumbnails, and etc.
PHP GD extension/library offers Graphics Drawing toos to manage image data such as cropping, compressing, filtering, and etc.
We will create a simple web application where the user can upload an image and the application itself will convert or resize the image to the specified height and width of the user. The application will be only accepting PNG, JPG, or JPEG files to upload. We will be using GD's imagecreatetruecolor(), imagecreatefrompng(), imagecreatefromjpeg(), imagecopyresampled(), and imagepng() functions in order to meet the simple application's requirement.
Getting Started
Kindly download and install a local web-server such as XAMPP/WAMP to run our PHP Script. Next, open your php.ini file and uncomment the Gd extention. To this, remove the semicolon before the extension. See th image below.
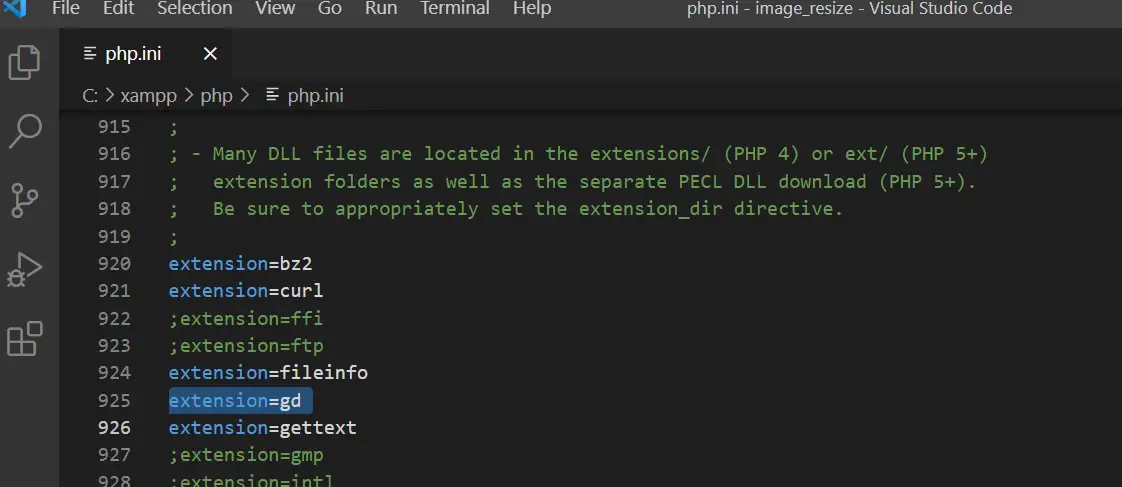
After that, open your XAMP/WAMP's Control Panel and start the Apache.
Also, download Bootstrap for the user interface.
Creating the Interface
We will be only making a simple user interface for this application which contains Form Panel where we can upload the image and specify the size we wanted for the convertion. Copy/Paste the script below and save the file as index.php. Make sure that path of external CSS and Script files are right according to your bootstrap assets located in your end.
- <?php
- session_start();
- ?>
- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8">
- <meta http-equiv="X-UA-Compatible" content="IE=edge">
- <meta name="viewport" content="width=device-width, initial-scale=1.0">
- <link rel="stylesheet" href="./Font-Awesome-master/css/all.min.css">
- <link rel="stylesheet" href="./css/bootstrap.min.css">
- <style>
- :root{
- --bs-success-rgb:71, 222, 152 !important;
- }
- html,body{
- height:100%;
- width:100%;
- }
- .display-img{
- object-fit:scale-down;
- object-position:center center;
- height:35vh;
- }
- </style>
- </head>
- <body>
- <main>
- <nav class="navbar navbar-expand-lg navbar-dark bg-dark bg-gradient" id="topNavBar">
- <div class="container">
- <a class="navbar-brand" href="./">
- Image Resize in PHP
- </a>
- <button class="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target="#navbarNav" aria-controls="navbarNav" aria-expanded="false" aria-label="Toggle navigation">
- </button>
- <div class="collapse navbar-collapse" id="navbarNav">
- <ul class="navbar-nav">
- <li class="nav-item">
- </li>
- </ul>
- </div>
- </div>
- </nav>
- <div class="container py-3" id="page-container">
- <?php
- if(isset($_SESSION['flashdata'])):
- ?>
- <div class="dynamic_alert alert alert-<?php echo $_SESSION['flashdata']['type'] ?>">
- <?php echo $_SESSION['flashdata']['msg'] ?>
- </div>
- <?php
- unset($_SESSION['flashdata']);
- endif;
- ?>
- <hr>
- <div class="col-12 row row-cols-2 gx-5 py-5">
- <div class="col-md-4">
- <div class="card">
- <div class="card-body">
- <form action="upload_file.php" enctype="multipart/form-data" method="POST">
- <div class="form-group">
- <div class="mb-3">
- <input class="form-control form-control-sm rounded-0" type="file" name="image" id="formFile" accept="image/png,image/jpeg" required>
- </div>
- </div>
- <div class="form-group">
- <input type="number" name="height" id="height" value="50" class="form-control form-control-sm rounded-0 text-end" required>
- </div>
- <div class="form-group">
- <input type="number" name="width" id="width" value="50" class="form-control form-control-sm rounded-0 text-end" required>
- </div>
- <div class="form-group d-flex justify-content-end my-3">
- <div class="col-auto">
- </div>
- </div>
- </form>
- </div>
- </div>
- </div>
- <div class="col-md-8">
- <div class="card h-100">
- <div class="card-body h-100 d-flex justiy-content-center align-items-center">
- <?php
- $original_file = "./upload/original.png";
- $resized_file = "./upload/resized.png";
- ?>
- <?php if(!is_file($original_file) && !is_file($resized_file)): ?>
- <div class="text-center w-100 lh-1">
- </div>
- <?php else: ?>
- <?php
- list($o_img_width,$o_img_height) = getimagesize($original_file);
- list($r_img_width,$r_img_height) = getimagesize($resized_file);
- ?>
- <div class="w-100 row row-cols-2 gx-5 mx-0">
- <div class="col-md-6">
- <div class="card">
- <img src="<?php echo $original_file."?".time() ?>" class='img-top display-img bg-dark' alt="Original Image File">
- <div class="card-body">
- <p class="lh-1 mt-2">
- <br>
- </p>
- </div>
- </div>
- </div>
- <div class="col-md-6">
- <div class="card">
- <img src="<?php echo $resized_file."?".time() ?>" class='img-top display-img bg-dark' alt="Resized Image File">
- <div class="card-body">
- <p class="lh-1 mt-2">
- <br>
- </p>
- </div>
- </div>
- </div>
- </div>
- <?php endif; ?>
- </div>
- </div>
- </div>
- </div>
- </div>
- </main>
- </body>
- </html>
Creating the Main Function
The script shown below is the code process the user file uploaded file. In contains the checking of the validity of the file upload, directory and file creation, and the most important is the script for resizing the uploaded image. Save the script in a new PHP file as upload_filephp.
- <?php
- // creating the upload directory if doesn't exists
- // Extracting the POST Data into variables
- //Image File Uploaded
- $upload = $_FILES['image'];
- // Identifing File Mime Type
- // Valid File types
- $_SESSION['flashdata']['type']="danger";
- $_SESSION['flashdata']['msg']="Image File type is invalid.";
- }else{
- // new size variables
- $new_height = $height; // $height :: $_POST['height']
- $new_width = $width; // $width :: $_POST['width']
- // Get the original size
- // creating a black image as representaion for the image new size
- // Creating the GD Image from uploaded image file
- $gdImg = ($type =='image/png') ? imagecreatefrompng($upload['tmp_name']) : imagecreatefromjpeg($upload['tmp_name']);
- //Resizing the imgage
- // The following scripts are just for saving the image files in a directory only
- //Remove the existing resized file
- // saving the resized file in a directory as a PNG file
- // destroying the created image after saving
- //Remove the previous uploaded original file
- // saving the original file in a directory as a PNG file
- // destroying the created image after saving
- $_SESSION['flashdata']['type']="success";
- $_SESSION['flashdata']['msg']="Image convertion is successfull.";
- }
- }else{
- $_SESSION['flashdata']['type']="danger";
- $_SESSION['flashdata']['msg']="Image File is required.";
- }
- exit;
The source code contains a comment script which can help you to understand more the process.
DEMO VIDEO
There you go. You can now test the source code you created on your end. If you encounter any errors please review the source code scripts above and see if you did miss something. You can also download the working source code I created for this tutorial. The download button is located below.
That ends this tutorial. I hope this will help you with what you are looking for and for your future projects. Explore more on this website for more Tutorials and Free Source Codes.
Happy Coding :)
Add new comment
- 1355 views