How to Insert new Option in Select Tag Dynamically in JavaScript
How to Insert new Option in Select Tag Dynamically in JavaScript
Introduction
In this tutorial we will create a How to Insert new Option in Select Tag Dynamically in JavaScript. This tutorial purpose is to teach you to insert new option in select tag. This will cover all the basic function that will insert new options. I will provide a sample program to show the actual coding of this tutorial.
This tutorial is simple and easy to understand just follow the instruction I provided and you can do it without a problem. This program can be use to any system or application that use selection tag as your choices. I will give my best to provide you the easiest way of creating this program Insert new Option in Select tag. So let's do the coding.
Before we get started:
This is the link for the template that i used for the layout design https://getbootstrap.com/.
Creating The Interface
This is where we will create a simple interface for our application. This code will display the html form with and button. To create this simply copy and write it into your text editor, then save it as index.html.- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- </div>
- </nav>
- <div class="col-md-6 well">
- <hr style="border-top:1px dotted #ccc;"/>
- <div class="col-md-4">
- <div class="form-inline">
- <input type="text" id="option" class="form-control"/>
- </div>
- <br />
- <br />
- <div class="form-group">
- </div>
- </div>
- </div>
- </body>
- </html>
Creating JavaScript Function
This is where the main function of the application is. This code will allow you to add new options in select tag dynamically. To do this just copy and write these block of codes inside the text editor and save it as script.js.- function createOption(){
- let select = document.getElementById('select');
- let option = document.getElementById('option');
- if(option.value == ""){
- alert("Please enter something first!");
- }else{
- let newOption = document.createElement("option");
- let newOptionValue = document.createTextNode(option.value);
- newOption.appendChild(newOptionValue);
- select.insertBefore(newOption, select.lastChildNode);
- option.value = "";
- select.value = "";
- }
- }
- function submitData(){
- let data = document.getElementById('select').value;
- if(data==""){
- alert('Please select an item first');
- }else{
- alert('You select '+data+'');
- }
- }
In the code above we will a create a method called createOption. This function is the one the will create a new options for your select tag. This method use a a function that will create a new text called createTextNode(). Then it will now append the newly created text into the select options using the appendChild() and insertBefore() function.
Output:
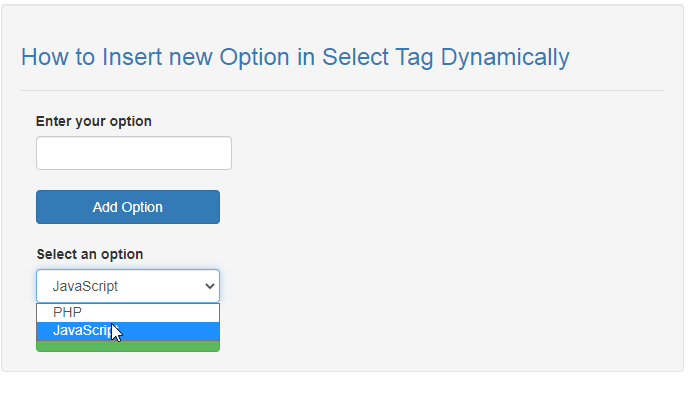
The How to Insert new Option in Select Tag Dynamically in JavaScript source code that I provide can be download below. Please kindly click the download button.
There you have it we successfully created How to Insert new Option in Select Tag Dynamically in JavaScript. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for JavaScript Language