Login with SQL Server 2008 Database in C#
Submitted by donbermoy on Monday, October 26, 2015 - 09:05.
In this tutorial, I will teach you how to create a login program that will have records on the database and will be accessed to login for the security.
So, now let's start this tutorial!
1. Let's start with creating a Windows Form Application for this tutorial by following the following steps in Microsoft Visual Studio 2010: Go to File, click New Project, and choose Windows Application.
2. Add two textboxes named txtuser and txtpass for the validation of username and password, and two buttons named btnLogin and btnClose of the Login and Closing of the form.
3. We will first create a database named Login using the SQL Server 2008 database.
Create a table named tbl_Login and have an entity of username, password, and uname with a varchar datatype.
Then put records inside the table and we will have this validated in our login form.
4. Now, let's do the coding.
We will first import the SQL namespace as we will have the SQL database.
Have the connection string of your sql database, the command, connection, and the data reader.
For the close button for closing the form, have this code below:
.
Call the connection of your database to open it in the Form_Load.
.
Output:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
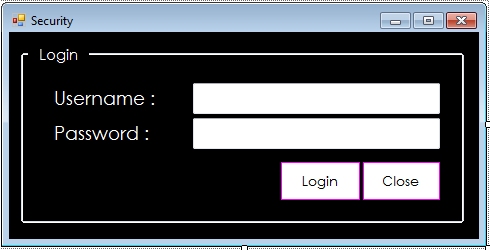
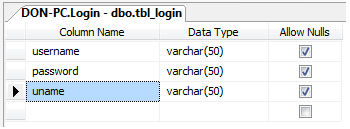
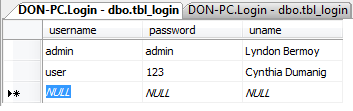
- using System.Data.SqlClient;
Now, we will code for our Login button. Have this code below:
- private void btnLogin_Click(object sender, EventArgs e)
- {
- try
- {
- // query the table to validate the inputted text of the textboxes
- // to match the records in the entity of username and password
- string sql = "select * from tbl_login where username like '" + txtUser.Text + "' and password like '"
- + txtPass.Text + "'";
- dr = cm.ExecuteReader();
- dr.Read();
- if (dr.HasRows) //if the username and password matches
- {
- MessageBox.Show("\tWelcome " + dr.GetValue(2).ToString() + " \n Time&Date Login : " + DateTime.Now.ToString() + "", "Login Security", MessageBoxButtons.OK, MessageBoxIcon.Information);
- dr.Close();
- return;
- }
- else //if record is not found
- {
- MessageBox.Show("Access Denied!", "Message", MessageBoxButtons.OK, MessageBoxIcon.Error);
- dr.Close();
- }
- }
- catch (Exception ex) //if there is an error in the input
- {
- MessageBox.Show(ex.Message, "Message", MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
- }
- }
- private void btnClosed_Click(object sender, EventArgs e)
- {
- Environment.Exit(0);
- }
- .
- private void Form1_Load(object sender, EventArgs e)
- {
- cn.Open();
- // for the password character to have an askterisk
- txtPass.PasswordChar = '*';
- }
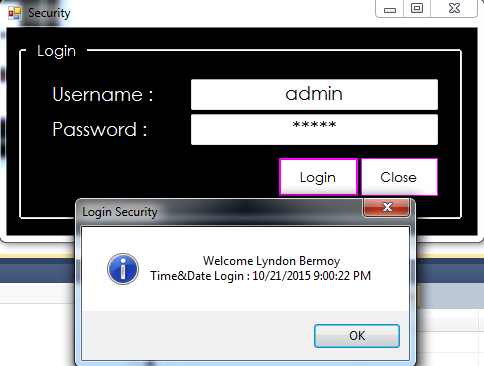