Alarm Clock Using HTML JavaScript
Submitted by alpha_luna on Friday, May 20, 2016 - 16:30.
Language
Alarm Clock Using HTML JavaScript
This is a "24-hour cycle" JavaScript alarm clock that opens any web page (depends on what address you input in Set Alarm Action field) according to the time user set up. This is a very cool system. Try this simple program and you can use it as your alarm. Hope this work will help you in your project.JavaScript Source Code
- <script type="text/javascript">
- var jsalarm={
- padfield:function(f){
- return (f<10)? "0"+f : f
- },
- showcurrenttime:function(){
- var dateobj=new Date()
- var ct=this.padfield(dateobj.getHours())+":"+this.padfield(dateobj.getMinutes())+":"+this.padfield(dateobj.getSeconds())
- this.ctref.innerHTML=ct
- this.ctref.setAttribute("title", ct)
- if (typeof this.hourwake!="undefined"){ //if alarm is set
- if (this.ctref.title==(this.hourwake+":"+this.minutewake+":"+this.secondwake)){
- clearInterval(jsalarm.timer)
- window.location=document.getElementById("musicloc").value
- }
- }
- },
- init:function(){
- var dateobj=new Date()
- this.ctref=document.getElementById("alarm_alarm")
- this.submitref=document.getElementById("submit_submit")
- this.submitref.onclick=function(){
- jsalarm.setalarm()
- this.value="Alarm Set"
- this.disabled=true
- return false
- }
- this.resetref=document.getElementById("reset_reset")
- this.resetref.onclick=function(){
- jsalarm.submitref.disabled=false
- jsalarm.hourwake=undefined
- jsalarm.hourselect.disabled=false
- jsalarm.minuteselect.disabled=false
- jsalarm.secondselect.disabled=false
- return false
- }
- var selections=document.getElementsByTagName("select")
- this.hourselect=selections[0]
- this.minuteselect=selections[1]
- this.secondselect=selections[2]
- for (var i=0; i<60; i++){
- if (i<24) //If still within range of hours field: 0-23
- this.hourselect[i]=new Option(this.padfield(i), this.padfield(i), false, dateobj.getHours()==i)
- this.minuteselect[i]=new Option(this.padfield(i), this.padfield(i), false, dateobj.getMinutes()==i)
- this.secondselect[i]=new Option(this.padfield(i), this.padfield(i), false, dateobj.getSeconds()==i)
- }
- jsalarm.showcurrenttime()
- jsalarm.timer=setInterval(function(){jsalarm.showcurrenttime()}, 1000)
- },
- setalarm:function(){
- this.hourwake=this.hourselect.options[this.hourselect.selectedIndex].value
- this.minutewake=this.minuteselect.options[this.minuteselect.selectedIndex].value
- this.secondwake=this.secondselect.options[this.secondselect.selectedIndex].value
- this.hourselect.disabled=true
- this.minuteselect.disabled=true
- this.secondselect.disabled=true
- }
- }
- </script>
Form Field
- <form action="" method="">
- <div id="jsalarmclock">
- <div>
- </div>
- <div>
- </div>
- <div>
- <input type="text" class="text_menu" id="musicloc" size="55" value="https://www.youtube.com/watch?v=wU2BDZkv17k" />
- <span style="font: normal 11px Tahoma">
- </span>
- </div>
- <input type="submit" class="button_menu" value="Set Alarm!" id="submit_submit" />
- <input type="reset" class="button_menu" value="reset" id="reset_reset" />
- </div>
- </form>
- <style type="text/css">
- body {
- color:blue;
- }
- #jsalarmclock{
- font-family: Tahoma;
- font-weight: bold;
- font-size: 12px;
- }
- #jsalarmclock div{
- margin-bottom: 0.8em;
- }
- #jsalarmclock div.leftcolumn{
- float: left;
- width: 150px;
- font-size: 13px;
- clear: left;
- }
- #jsalarmclock span{
- margin-right: 5px;
- }
- .button_menu {
- width: 100px;
- height: 28px;
- background-color: buttonface;
- border: 1px solid red;
- border-radius: 10px;
- color: blue;
- font-size:18px;
- }
- .select_menu {
- width: 100px;
- height: 28px;
- background-color: buttonface;
- border: 1px solid red;
- border-radius: 10px;
- color: red;
- }
- .text_menu {
- width: 400px;
- height: 28px;
- background-color: buttonface;
- border: 1px solid red;
- border-radius: 10px;
- color: blue;
- }
- </style>
Output:
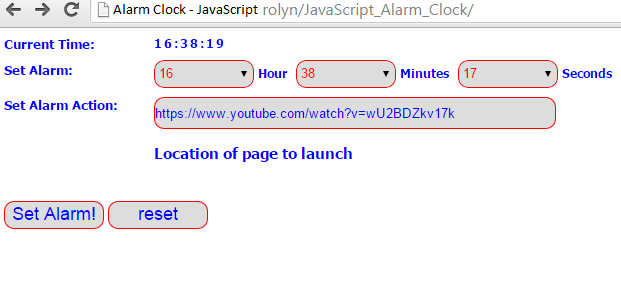
Note: Due to the size or complexity of this submission, the author has submitted it as a .zip file to shorten your download time. After downloading it, you will need a program like Winzip to decompress it.
Virus note: All files are scanned once-a-day by SourceCodester.com for viruses, but new viruses come out every day, so no prevention program can catch 100% of them.
FOR YOUR OWN SAFETY, PLEASE:
1. Re-scan downloaded files using your personal virus checker before using it.
2. NEVER, EVER run compiled files (.exe's, .ocx's, .dll's etc.)--only run source code.
Add new comment
- 2719 views