In this tutorial, we are going to create
Update Multiple Rows in PHP/MySQL with Checkbox. This tutorial will teach the user on how to create a simple program in PHP that can update multiple rows using the checkbox as the selector. The feature of this simple source code it can edit multiple data in the database table using a checkbox as a selector. Hope that this tutorial will help you in your future project.
Creating Database and Inserting Data
Our Database
CREATE TABLE `member` (
`member_id` INT(11) NOT NULL,
`firstname` VARCHAR(100) NOT NULL,
`lastname` VARCHAR(100) NOT NULL,
`middlename` VARCHAR(100) NOT NULL,
`address` VARCHAR(100) NOT NULL,
`email` VARCHAR(100) NOT NULL
) ENGINE=MyISAM DEFAULT CHARSET=latin1;
Example Data
INSERT INTO `member` (`member_id`, `firstname`, `lastname`, `middlename`, `address`, `email`) VALUES
Creating Form Field
Creating a table to view the example data where the user can select using a checkbox as you can see in the image below.
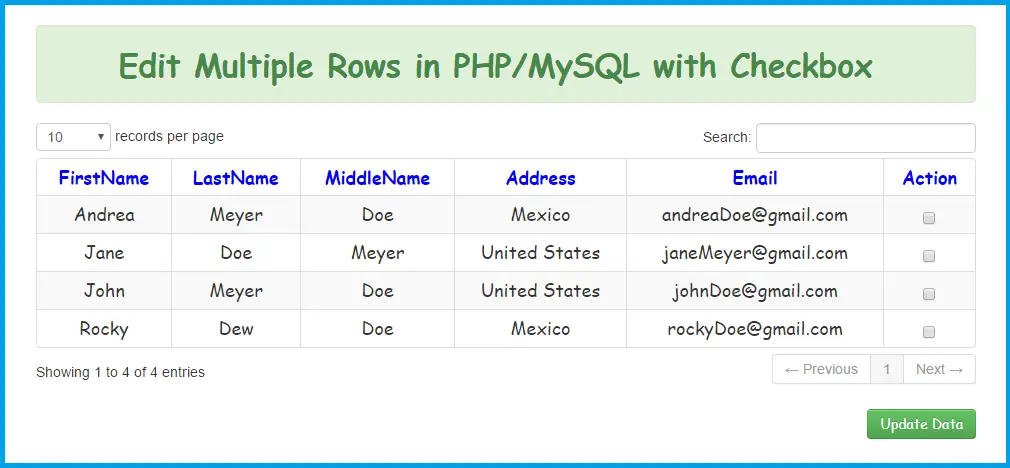
The source code of the image above.
<form action="edit.php" method="post">
<table cellpadding="0" cellspacing="0" border="0" class="table table-striped table-bordered" id="example">
<div class="alert alert-success">
<h2 style="text-align:center; font-family:cursive;">Edit Multiple Rows in PHP/MySQL with Checkbox
</h2>
<th style="text-align:center; font-family:cursive; font-size:18px; color:blue;">FirstName
</th>
<th style="text-align:center; font-family:cursive; font-size:18px; color:blue;">LastName
</th>
<th style="text-align:center; font-family:cursive; font-size:18px; color:blue;">MiddleName
</th>
<th style="text-align:center; font-family:cursive; font-size:18px; color:blue;">Address
</th>
<th style="text-align:center; font-family:cursive; font-size:18px; color:blue;">Email
</th>
<th style="text-align:center; font-family:cursive; font-size:18px; color:blue;">Action
</th>
<?php
$query=mysql_query("select * from member")or die(mysql_error());
while($row=mysql_fetch_array($query)){
$id=$row['member_id'];
?>
<td style="text-align:center; font-family:cursive; font-size:18px;"><?php echo $row['firstname'] ?></td>
<td style="text-align:center; font-family:cursive; font-size:18px;"><?php echo $row['lastname'] ?></td>
<td style="text-align:center; font-family:cursive; font-size:18px;"><?php echo $row['middlename'] ?></td>
<td style="text-align:center; font-family:cursive; font-size:18px;"><?php echo $row['address'] ?></td>
<td style="text-align:center; font-family:cursive; font-size:18px;"><?php echo $row['email'] ?></td>
<td style="text-align:center; font-family:cursive; font-size:18px;">
<input name="selector[]" type="checkbox" value="<?php echo $id; ?>">
<?php } ?>
<button class="btn btn-success pull-right" style="font-family:cursive;" name="submit_mult" type="submit">
Update Data
Creating Edit Page
After the user select desired data to update. This page where the user can edit the data to update into the database table as shown in the image below.
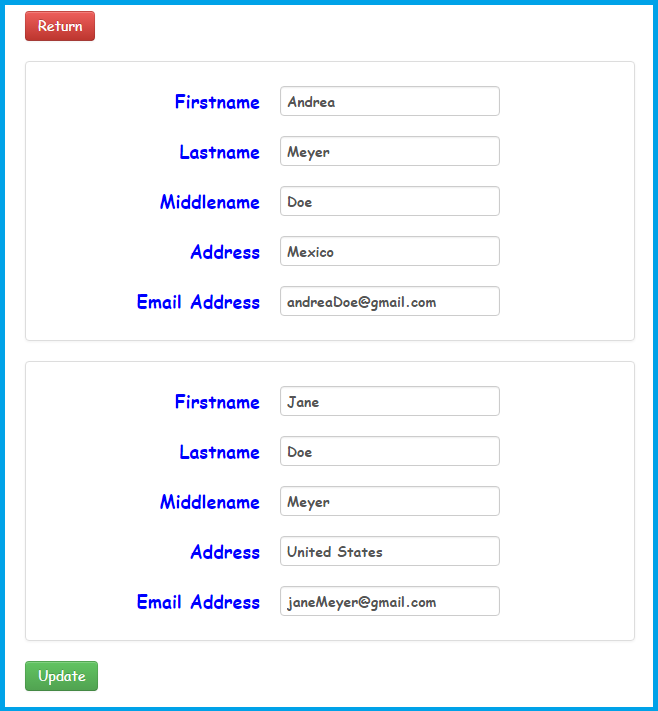
Here's the source code of the image above.
<a href="index.php" style="margin-left: 165px; font-family:cursive;" class="btn btn-danger">Return
</a>
<form class="form-horizontal" action="edit_save.php" method="post">
<?php
include('dbcon.php');
$id=$_POST['selector'];
$N = count($id);
for($i=0; $i < $N; $i++)
{
$result = mysql_query("SELECT * FROM member where member_id='$id[$i]'");
while($row = mysql_fetch_array($result))
{ ?>
<div class="thumbnail" style="margin:auto; width:600px;">
<div style="margin-left: 70px; margin-top: 20px;">
<div class="control-group">
<label class="control-label" for="inputEmail" style="font-family:cursive; font-weight:bold; font-size:18px; color:blue;">Firstname
</label>
<input name="member_id[]" type="hidden" value="<?php echo $row['member_id'] ?>" />
<input name="firstname[]" type="text" style="font-family:cursive; font-weight:bold;" value="<?php echo $row['firstname'] ?>" />
<div class="control-group">
<label class="control-label" for="inputEmail" style="font-family:cursive; font-weight:bold; font-size:18px; color:blue;">Lastname
</label>
<input name="lastname[]" type="text" style="font-family:cursive; font-weight:bold;" value="<?php echo $row['lastname'] ?>" />
<div class="control-group">
<label class="control-label" for="inputEmail" style="font-family:cursive; font-weight:bold; font-size:18px; color:blue;">Middlename
</label>
<input name="middlename[]" type="text" style="font-family:cursive; font-weight:bold;" value="<?php echo $row['middlename'] ?>" />
<div class="control-group">
<label class="control-label" for="inputEmail" style="font-family:cursive; font-weight:bold; font-size:18px; color:blue;">Address
</label>
<input name="address[]" type="text" style="font-family:cursive; font-weight:bold;" value="<?php echo $row['address'] ?>" />
<div class="control-group">
<label class="control-label" for="inputEmail" style="font-family:cursive; font-weight:bold; font-size:18px; color:blue;">Email Address
</label>
<input name="email[]" type="text" style="font-family:cursive; font-weight:bold;" value="<?php echo $row['email'] ?>" />
<?php
}
}
?>
<input name="" class="btn btn-success" style="margin-left: 165px; font-family:cursive;" type="submit" value="Update">
Database Name
multi_edit.sql
Kindly click the
"Download Code" button below for full source code. Thank you very much.
Hope that this tutorial will help you a lot.
Share us your thoughts and comments below. Thank you so much for dropping by and reading this tutorial post. For more updates, don’t hesitate and feel free to visit this website more often and please share this with your friends or email me at
[email protected]. Practice Coding. Thank you very much.