How To Create WCF Service
Submitted by thusitcp on Wednesday, October 15, 2014 - 23:24.
Language
Introduction
In this tutorial you are going to learn WCF one of the key features of Visual Studio. WCF stand for windows communication foundation. WCF is one of powerful introduction of .net framework and provide grate solutions for space left by the web services. Using WCF we can build secure, reliable, transacted solutions that integrate across platforms.
Building a distributed system is a very common task for many programmers. However, doing so involves confronting numerous design challenges. WCF has overcome most of the issues with most of distributed system previously had.
Features of WCF
Adding a DataContract
Right Click the project, select -> Add new Item, select -> web, select -> Class.
Name it -> Customer.cs
Service Interface
Implementing the service.
Usually WCF project can have lots of services but to implement successfully you should have at least one service.
Testing service.
It is very simple. Just press Ctrl + F5 then WCF test client will open. Now you can test you service without implement on server
- 1
- Service Orientation
- Interoperability
- Multiple Message Patterns
- Service Metadata
- Data Contracts
- Security
- Multiple Transports and Encodings
- Reliable and Queued Messages
- Durable Messages
- Transactions
- AJAX and REST Support
- Extensibility

- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Web;
- using System.Runtime.Serialization;
- namespace EchoService
- {
- [DataContract]
- public class Customer
- {
- [DataMember]
- public string customerName { get; set; }
- [DataMember]
- public string customerAddress { get; set; }
- [DataMember]
- public int customerAge { get; set; }
- [DataMember]
- public string customerCity { get; set; }
- }
- }
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Runtime.Serialization;
- using System.ServiceModel;
- using System.Text;
- namespace EchoService
- {
- // NOTE: You can use the "Rename" command on the "Refactor" menu to change the interface name "ICustomerService" in both code and config file together.
- [ServiceContract]
- public interface ICustomerService
- {
- [OperationContract]
- string GetCustomerName(string name);
- [OperationContract]
- string GetCustomerAddress(string address);
- [OperationContract]
- Customer GetCustomerDetails();
- [OperationContract]
- List<Customer> GetCustomerS();
- }
- }
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Runtime.Serialization;
- using System.ServiceModel;
- using System.Text;
- namespace EchoService
- {
- // NOTE: You can use the "Rename" command on the "Refactor" menu to change the class name "CustomerService" in code, svc and config file together.
- public class CustomerService : ICustomerService
- {
- public string GetCustomerName(string name)
- {
- return string.Format("Customer Name Is : {0}", name);
- }
- public string GetCustomerAddress(string address)
- {
- return string.Format("Customer Address Is : {0}", address);
- }
- public Customer GetCustomerDetails()
- {
- newCustomer.customerName = "Smith";
- newCustomer.customerAddress = "River Avanue NW11E London";
- newCustomer.customerAge = 25;
- newCustomer.customerCity = "London";
- return newCustomer;
- }
- public List<Customer> GetCustomerS()
- {
- newCustomer1.customerName = "Smith";
- newCustomer1.customerAddress = "River Avanue NW11E London";
- newCustomer1.customerAge = 25;
- newCustomer1.customerCity = "London";
- Clist.Add(newCustomer1);
- newCustomer2.customerName = "Jhone";
- newCustomer2.customerAddress = "No 125/2 Texas US";
- newCustomer2.customerAge = 40;
- newCustomer2.customerCity = "Texas";
- Clist.Add(newCustomer2);
- return Clist;
- }
- }
- }
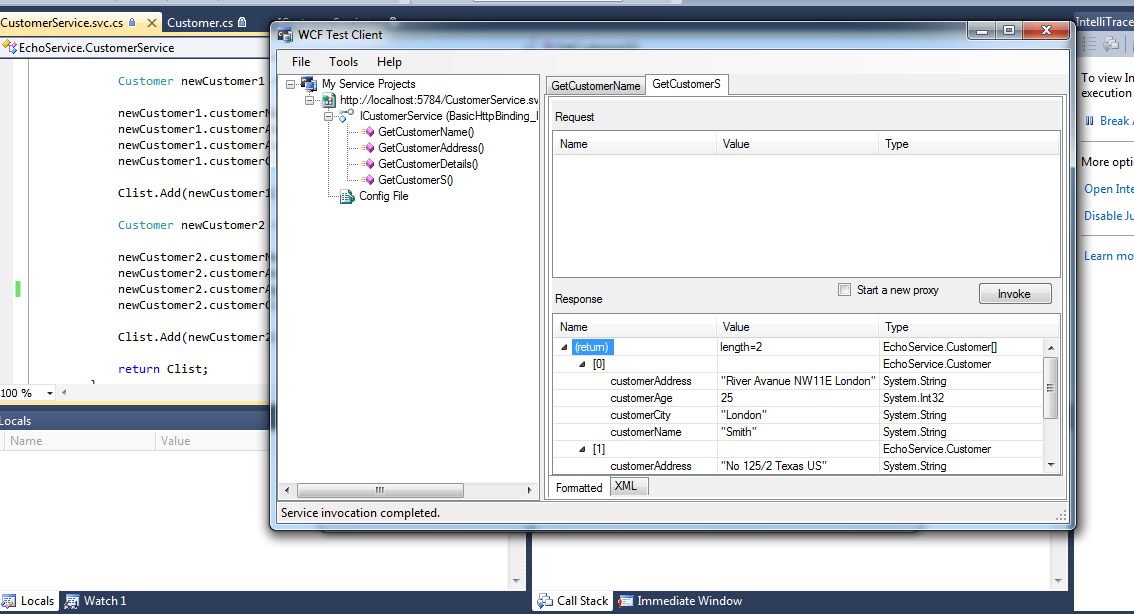
Note: Due to the size or complexity of this submission, the author has submitted it as a .zip file to shorten your download time. After downloading it, you will need a program like Winzip to decompress it.
Virus note: All files are scanned once-a-day by SourceCodester.com for viruses, but new viruses come out every day, so no prevention program can catch 100% of them.
FOR YOUR OWN SAFETY, PLEASE:
1. Re-scan downloaded files using your personal virus checker before using it.
2. NEVER, EVER run compiled files (.exe's, .ocx's, .dll's etc.)--only run source code.
Add new comment
- 199 views