C#: Retrieving and Deleting with SQL Sever
Submitted by janobe on Monday, July 4, 2016 - 09:10.
In this tutorial, I will teach you how to delete and retrieve the data in C#.net and SQL server 2005. With this, you can retrieve and delete the data in the database. This method is very helpful when developing a system because you can delete the test data that you have saved in the database.
3. Open Microsoft Visual Studio and create new Windows Form Application for c#.
4. Do the Form just like this.
5. Go to the Solution Explorer, hit the “View Code” to fire the code editor.
6. Declare all the classes and variable that are needed.
8. Go back to the Design View, double-click the Form and do the following codes for the connection between C# and SQL server 2005 express edition .
9. Go back to the Design View again, double-click the button and do the following codes in the method for deleting the records in the SQL database.
For all students who need a programmer for your thesis system or anyone who needs a source code in any programming languages. You can contact me @ :
Email – [email protected]
Mobile No. – 09305235027 – TNT
So, let’s get started:
1. Create a database and name it “employeedb”. 2. Do the following query for creating a table in the SQL Server database.- /****** Object: Table [dbo].[tbltest] Script Date: 06/13/2016 00:10:30 ******/
- SET ANSI_NULLS ON
- GO
- SET QUOTED_IDENTIFIER ON
- GO
- CREATE TABLE [dbo].[tblemployee](
- [ID] [INT] IDENTITY(1,1) NOT NULL,
- [Firstname] [nvarchar](50) NULL,
- [Lastname] [nvarchar](50) NULL,
- [Address] [nvarchar](MAX) NULL,
- [ContactNo] [nvarchar](50) NULL,
- [EmailAdd] [nvarchar](50) NULL
- ) ON [PRIMARY]
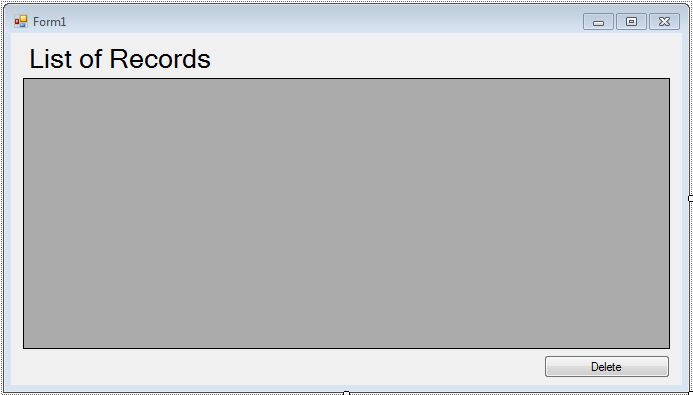
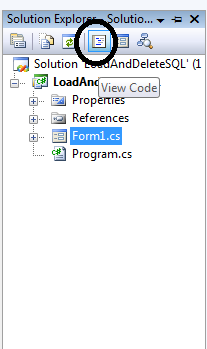
Note: Put using 'System.Data.SqlClient;' above the namespace to access sql server library.
7. Create a method for displaying records in the dataGridview from SQL database.
- private void LoadRecords()
- {
- try
- {
- //create a query for retrieving data in the database.
- query = "SELECT * FROM tblemployee";
- //initialize new Sql commands
- //hold the data to be executed.
- cmd.Connection = con;
- cmd.CommandText = query;
- //initialize new Sql data adapter
- //fetching data in the database.
- da.SelectCommand = cmd;
- //initialize new datatable
- //refreshes the rows in specified range in the datasource.
- da.Fill(dt);
- //Get and set the data source of a Datagridview
- dataGridView1.DataSource = dt;
- }
- catch (Exception ex)
- {
- MessageBox.Show(ex.Message);
- }
- finally
- {
- da.Dispose();
- }
- }
- //connection between SQL server database to c#
- con.ConnectionString = "Data Source=.\\SQLEXPRESS;Database=employeedb;trusted_connection=true;";
- //calling a retrieve method
- LoadRecords();
- try
- {
- //opening connection
- con.Open();
- //CREATE a DELETE query;
- query = "DELETE FROM tblemployee WHERE ID=" + dataGridView1.CurrentRow .Cells[0].FormattedValue ;
- //it holds the DATA TO be executed.
- cmd.Connection = con;
- cmd.CommandText = query;
- //EXECUTE the DATA.
- INT RESULT = cmd.ExecuteNonQuery();
- //validate IF the RESULT OF the executed query.
- IF (res > 0)
- {
- MessageBox.Show("Data has been deleted in the SQL database");
- //calling a retrieve method
- LoadRecords();
- }
- ELSE
- {
- MessageBox.Show("SQL QUERY ERROR");
- }
- //closing connection
- con.Close();
- }
- catch (Exception ex)//catch exeption
- {
- //displaying an error message.
- MessageBox.Show(ex.Message);
- }