Python TypeError: 'list' object cannot be interpreted as an integer [Solved]
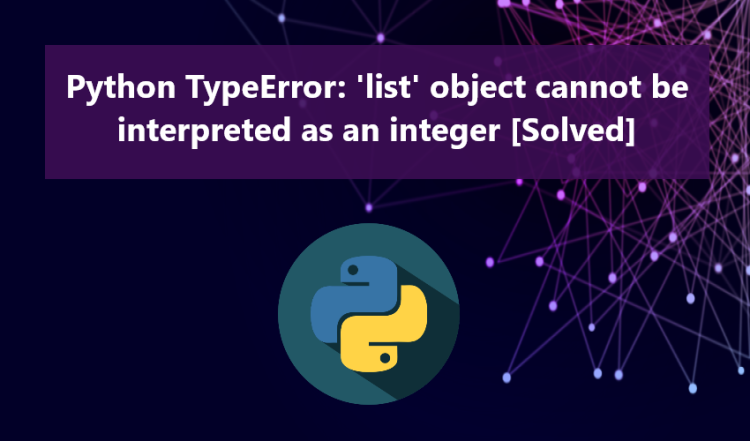
In this article, we will thoroughly explore the root causes and solutions for the `Python TypeError
` that manifests as `TypeError: 'list' object cannot be interpreted as an integer
`. If you're currently grappling with this error during your project development, this article will provide valuable insights into the error's nature and guide you on resolving it. Additionally, we will include code snippets to aid in better comprehension.
What is TypeError in Python?
In Python, a `TypeError
` stands as an exception that emerges when an operation is executed on an object with an improper or incompatible data type. It serves as a signal that the code is attempting to use an object in a manner that is not suitable for its data type. Python's dynamic typing allows variables to shift data types during runtime, rendering TypeError a frequent occurrence when the interpreter encounters unexpected data types.
This type of error can sometimes manifest as the following:
- TypeError: can't subtract offset-naive and offset-aware datetimes
- TypeError: bad operand type for unary +: 'str'
- TypeError: can't compare offset-naive and offset-aware datetimes
You can click the error messages above to access their respective dedicated articles for more in-depth insights.
Why does the Python TypeError: 'list' object cannot be interpreted as an integer occur?
In Python, the error `TypeError: 'list' object cannot be interpreted as an integer
` arises when you attempt to use a list as an integer in an operation where Python anticipates an integer. This error is triggered by a mismatch in the data types of the objects involved in the operation.
Below, you'll find some of the reasons behind the occurrence of `TypeError: 'list' object cannot be interpreted as an integer
`. We've also included sample code snippets that demonstrate the error and its solutions.
Using a `list` Object as an Argument for the `range()
` Function
The error is triggered when you attempt to use a `list` object as an argument in the `range()
` function. This error occurs because the function expects the argument to be an integer, but a `list` object has been provided instead.
- sampleList = [6, 23, 14]
- for data in range(sampleList):
- print(data)
To resolve this error, it's crucial to ensure that you provide the correct argument data type to the `range()
` function, which expects an integer data type. To achieve this, you can utilize the `len
` function to determine the length of the list object, allowing you to iterate through the data appropriately.
- sampleList = [6, 23, 14]
- for i in range(len(sampleList)):
- print(sampleList[i])
Another approach is to utilize the `enumerate()
` function instead of relying on the `range()
` function to iterate through the list object.
- sampleList = [6, 23, 14]
- for key, value in enumerate(sampleList):
- print(value)
Using a `list` Object as an Argument for the `pop()
` Function
Similar to the first reason mentioned, when you attempt to use a list object as an argument for the `pop()
` function, it will result in a `TypeError: 'list' object cannot be interpreted as an integer
`.
Example
- sampleList = [6, 23, 14]
- sampleList.pop([1])
- print(sampleList)
To resolve the error, it's essential to provide an integer as the argument for the `pop()
` function.
- sampleList = [6, 23, 14]
- sampleList.pop(1)
- print(sampleList)
Conclusion
In conclusion, the `TypeError: 'list' object cannot be interpreted as an integer
` error in Python occurs when you attempt to use a list object as the argument to a function that requires an integer argument. To fix the error, you must ensure to provide the correct data type to the function.
And there you go! I hope this article will help you address the error that you are currently grappling with. Explore more on this website for more Free Source Codes, Tutorials, and Articles that covers various programming language.
Happy Coding =)
- 3961 views