How to Fix the "PHP Warning: Array to string conversion" error in PHP
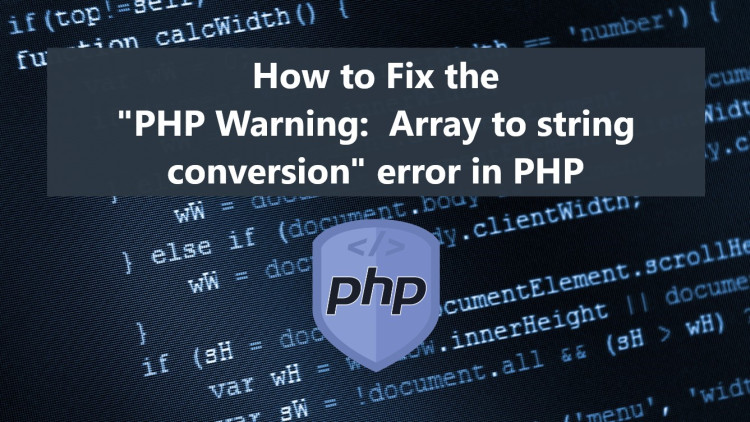
If you are facing a PHP warning or error that says "Warning: Array to string conversion", this article will help you understand why this kind of PHP error occurs and how you can solve this and prevent this error from occurring in your future projects.
What is PHP Array?
In PHP, an Array exists as an ordered map which is map is a type that binds values to keys. This type is designed to be used in a variety of applications; it can be used as an array, list (vector), dictionary, collection, stack, queue, hash table (an implementation of a map), and possibly more.
Why does the "PHP Warning: Array to string conversion" warning occurs?
Basically, the "PHP Warning: Array to string conversion ..." occurs when the developer is trying or unintentionally outputting an array of data. An Array of data cannot be converted into a string that's why this PHP warning occurs when we try it.
Although this is only a PHP Warning, the script may still continue to execute the succeeding PHP script for some instances such as your project is set up that the error won't display on the font-end but will only display an "Array" text instead of what we intended to output.
Here's an example PHP script that simulates the PHP Warning:
- <?php
- // Sample Array
- $myArray = [ 'Foo', 'Bar', 'Hello World'];
- echo $myArray;
- ?>
Or,
- <?php
- // Sample Array
- $myArray = [ 'Foo', 'Bar', 'Hello World'];
- print($myArray);
- ?>
Both PHP scripts will return a PHP Warning like the following images:
Fortunately, this kind of PHP Warning can be easily fixed and lots of built-in PHP functions and statements can be useful to solve this.
"PHP Warning: Array to string conversion ..." [Solved]
Here are the following solutions that can solve the said PHP Warning.
Solution 1: Using print_r()
The print_r() is a built-in function in PHP that prints human-readable information about the given variable which means this function will output the variable details and its values even for arrays or objects.
- <?php
- // Sample Array
- $myArray = [ 'Foo', 'Bar', 'Hello World'];
- ?>
Result
Solution 2: Using var_dump()
The var_dump() function is a built-in function that dumps the variable information.
- <?php
- // Sample Array
- $myArray = [ 'Foo', 'Bar', 'Hello World'];
- ?>
Result
Solution 3: Using implode()
The implode() function is a built-in function that joins the array items with a string as their separator.
- <?php
- // Sample Array
- $myArray = [ 'Foo', 'Bar', 'Hello World'];
- ?>
Result
Solution 4: Using foreach loop
The foreach loop provides an easy way to iterate over arrays. This structure only works on arrays and objects.
- <?php
- // Sample Array
- $myArray = [ 'Foo', 'Bar', 'Hello World'];
- foreach($myArray as $value){
- echo $value. "\n";
- }
- ?>
Result
Solution 5: Using json_encode()
The json_encode() function is a built-in function that represents the JSON string of a value.
- <?php
- // Sample Array
- $myArray = [ 'Foo', 'Bar', 'Hello World'];
- ?>
Result
There you go! That's how we can fix the "PHP Warning: Array to string conversion". I hope this article helps you understand why this PHP warning occurs and how to fix this.
Explore more on this website for more Tutorials and Free Source Codes.