How to fix the "AttributeError: module 'datetime' has no attribute 'now'" in Python
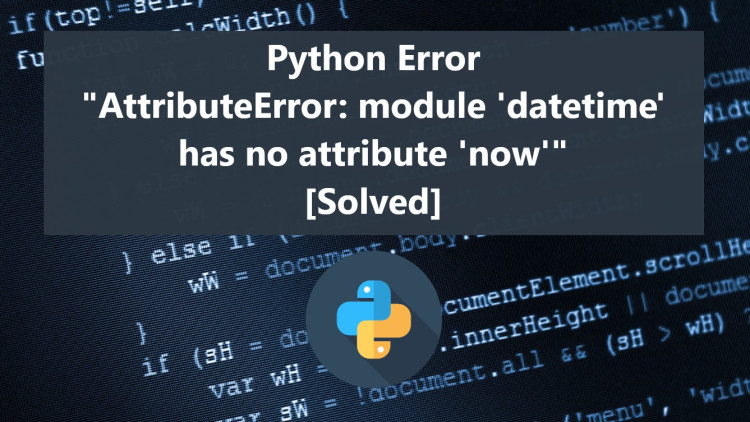
If you are facing the Python Attribute Error that says "AttributeError: module 'datetime' has no attribute 'now'", this article will help you understand why this error occurred and how to fix it. I will be giving also sample scripts that simulate the error to occur and explain why and solve it.
Why does "AttributeError: module 'datetime' has no attribute 'now'" occurs?
The "AttributeError: module 'datetime' has no attribute 'now' tells you that the module known as datetime has no attribute called now. It is because we are trying to output the current date and time straight after the datetime.
Here's a simple and best sample that simulates the scenario
test.py
- import datetime
- print(datetime.now())
The script above shows that the datetime module is being imported to the Python file script and trying to output the current date and time using the now attribute directly. Unfortunately, this script will return an Attribute Error stating that the said module does not have or contain a now which is actually true.
The error occurred because we are trying to get the current date and time directly from the module which should not be. The now method that we are trying to get is from the datetime class of the datetime module. This kind of error can be solved easily in many ways.
Solution #1: Defining the datetime class
One of these error solutions is to define the datetime class first as the attribute of the datetime module. Check out the sample script below.
- import datetime
- print(datetime.datetime.now())
- #Outputs the current date when executing the script
The script above shows that we must get the datetime class first from the datetime module so we can call the now() method. We must understand that we just only imported the datetime module and not directly the datetime class. We can check all the attributes and methods of the datetime module by running the following script.
- import datetime
- help(datetime)
Solution 2: Importing the datetime
We can also solve this problem by importing only the datetime class and not the whole module. To do that we can do something like the following.
- #From datetime module import datetime class
- from datetime import datetime
- print(datetime.now())
This time we can now directly call the now() method because we only imported the datetime class. We can also make an alias for the datetime class like the following.
- from datetime import datetime as dt
- print(dt.now())
If you try to execute the following Python script, you will spot the difference.
Importing the Module
- import datetime
- print(datetime)
Result
Importing the Class
- import datetime
- print(datetime)
Result
There you go! That is how you can solve the "AttributeError: module 'datetime' has no attribute 'now'" Python error and how you can prevent it from occurring in your current and future projects.
Explore more on this website for more Tutorials and Free Source Codes.
Happy Coding =)
- Add new comment
- 10353 views