Creating Cannon and Camera Movement
Submitted by razormist on Wednesday, March 14, 2018 - 22:20.
Creating Cannon
We will now create the object that will shoot the enemy and the structure. To do that first locates the sprite inside the Sprites directory.- Drag the body sprite of the cannon to the Scene View.
After that drag another sprite as a child for the body to complete the cannon GameObject.
- Next is to set the component to each of the GameObject.
- After setting up the components add a GameObject to Cannon as a child. This will be the spawning point for the bullet. Set its components as shown below.
- Then Create two GameObject as child for the Player GameObject. This will be the wheel for the cannon to be able to stand on the ground. Set it component as shown below.
- Go to the Scripts folder and create a new folder inside of it called Player.
- Create a C# script called Cannon.
- Write these block of code inside the Cannon Class:
- public GameObject cannonBullet;
- public Transform cannonTip;
- public Slider powerLevel;
- public AudioClip cannonShot;
- public int maxShot;
- public bool readyToShoot;
- public int shot;
- private int minLevel, maxLevel, timeDelay;
- private bool isMax, isCharging;
- private AudioSource audioSource;
- private float minRot, maxRot;
- private Vector3 currentRotation, touchPos;
- private int counter = 0;
- private Vector2 direction = Vector2.zero;
- void Awake(){
- audioSource = GetComponent<AudioSource> ();
- }
- // Use this for initialization
- void Start () {
- readyToShoot = true;
- shot = maxShot;
- timeDelay = 50;
- minLevel = 0;
- maxLevel = 50;
- powerLevel.maxValue = maxLevel;
- powerLevel.value = minLevel;
- maxRot = 20;
- minRot = -25;
- Vector3 currentRotation = transform.rotation.eulerAngles;
- }
- // Update is called once per frame
- void Update () {
- if (GameplayController.instance.gameInProgress) {
- if (readyToShoot) {
- if(Application.platform == RuntimePlatform.Android){
- TouchCannonShoot ();
- }else if(Application.platform == RuntimePlatform.WindowsEditor){
- CannonShoot ();
- }
- }
- if(Application.platform == RuntimePlatform.Android){
- TouchCannonMovement ();
- }else if(Application.platform == RuntimePlatform.WindowsEditor){
- CannonMovement ();
- }
- }
- }
- void TouchCannonShoot(){
- if(Input.touchCount > 0){
- Touch touch = Input.GetTouch (0);
- touchPos = Camera.main.ScreenToWorldPoint (touch.position);
- RaycastHit2D hit = Physics2D.Raycast (touchRayHit , Vector2.zero);
- if(hit.collider != null){
- if(hit.collider.CompareTag("Player")){
- if(touch.phase == TouchPhase.Stationary){
- if (shot != 0) {
- UpdatePowerLevel ();
- isCharging = true;
- }
- }else if(touch.phase == TouchPhase.Ended){
- if (shot != 0) {
- GameObject newCannonBullet = Instantiate (cannonBullet, cannonTip.position, Quaternion.identity) as GameObject;
- newCannonBullet.transform.GetComponent<Rigidbody2D> ().AddForce (cannonTip.right * powerLevel.value, ForceMode2D.Impulse);
- if (GameController.instance != null && MusicController.instance != null) {
- if (GameController.instance.isMusicOn) {
- audioSource.PlayOneShot (cannonShot);
- }
- }
- shot--;
- powerLevel.value = 0;
- readyToShoot = false;
- isCharging = false;
- }
- }
- }
- }
- /*if(touch.phase == TouchPhase.Stationary){
- if (shot != 0) {
- UpdatePowerLevel ();
- }
- }else if(touch.phase == TouchPhase.Ended){
- if (shot != 0) {
- GameObject newCannonBullet = Instantiate (cannonBullet, cannonTip.position, Quaternion.identity) as GameObject;
- newCannonBullet.transform.GetComponent<Rigidbody2D> ().AddForce (cannonTip.right * powerLevel.value, ForceMode2D.Impulse);
- if (GameController.instance != null && MusicController.instance != null) {
- if (GameController.instance.isMusicOn) {
- audioSource.PlayOneShot (cannonShot);
- }
- }
- shot--;
- powerLevel.value = 0;
- readyToShoot = false;
- }
- }*/
- }
- }
- void CannonShoot(){
- if (Input.GetKey (KeyCode.Space)) {
- if(shot != 0){
- UpdatePowerLevel ();
- }
- }else if(Input.GetKeyUp(KeyCode.Space)){
- if (shot != 0) {
- GameObject newCannonBullet = Instantiate (cannonBullet, cannonTip.position, Quaternion.identity) as GameObject;
- newCannonBullet.transform.GetComponent<Rigidbody2D> ().AddForce (cannonTip.right * powerLevel.value, ForceMode2D.Impulse);
- if (GameController.instance != null && MusicController.instance != null) {
- if (GameController.instance.isMusicOn) {
- audioSource.PlayOneShot (cannonShot);
- }
- }
- shot--;
- powerLevel.value = 0;
- readyToShoot = false;
- }
- }
- }
- void UpdatePowerLevel(){
- if (!isMax) {
- powerLevel.value += timeDelay * Time.deltaTime;
- if(powerLevel.value == maxLevel){
- isMax = true;
- }
- } else {
- powerLevel.value -= timeDelay * Time.deltaTime;
- if(powerLevel.value == minLevel){
- isMax = false;
- }
- }
- }
- void CannonMovement(){
- if (Input.GetKey (KeyCode.UpArrow)) {
- currentRotation.z += 50f * Time.deltaTime;
- } else if (Input.GetKey (KeyCode.DownArrow)) {
- currentRotation.z -= 50f * Time.deltaTime;
- }
- currentRotation.z = Mathf.Clamp(currentRotation.z, minRot, maxRot);
- transform.rotation = Quaternion.Euler (currentRotation);
- }
- void TouchCannonMovement(){
- if(Input.touchCount > 0){
- Touch touch = Input.GetTouch (0);
- if (touch.position.x < 300 && !isCharging && touch.position.y > 160) {
- if (touch.phase == TouchPhase.Moved) {
- Vector2 touchDeltaPosition = touch.deltaPosition;
- direction = touchDeltaPosition.normalized;
- if (direction.y > 0 && touchDeltaPosition.x > -10 && touchDeltaPosition.x < 10) {
- currentRotation.z += 50f * Time.deltaTime;
- } else if (direction.y < 0 && touchDeltaPosition.x > -10 && touchDeltaPosition.x < 10) {
- currentRotation.z -= 50f * Time.deltaTime;
- }
- }
- }
- }
- currentRotation.z = Mathf.Clamp(currentRotation.z, minRot, maxRot);
- transform.rotation = Quaternion.Euler (currentRotation);
- }
- After creating the script, drag the script to the Cannon inspector as a component.
- Next we will create a power shot indicator for the cannon, this will tell how much force the shot will can reach the enemies. To do that go to GameObject and UI, select then the Slider.
- Set all the Components of the Slider as shown below to optimize the game.
Slider
Background
Fill
Creating Cannon Bullet
We will now create the object that will destroy enemies and structures when collide. To do that first locates the sprite inside the Sprites directory.- Drag the sprite GameObject to the Scene View.
- Next set the component of the Bomb GameObject.
- Go to the Scripts folder and select Player. Create a C# script called Cannon Bullet.
- Write these block of code inside the CannonBullet Class:
- public GameObject explosionEffect;
- public bool isIdle;
- private Rigidbody2D rigidBody2D;
- private bool cannonShot, isExplode;
- void Awake(){
- rigidBody2D = GetComponent<Rigidbody2D> ();
- }
- // Use this for initialization
- void Start () {
- cannonShot = true;
- }
- // Update is called once per frame
- void Update () {
- if (GameplayController.instance.gameInProgress) {
- if (cannonShot && rigidBody2D.velocity.sqrMagnitude <= 0) {
- DestroyBullet ();
- }
- }
- }
- void DestroyBullet(){
- Destroy (gameObject);
- if (!isExplode) {
- isExplode = true;
- GameObject newExplosionEffect = Instantiate (explosionEffect, transform.position, Quaternion.identity) as GameObject;
- Destroy (newExplosionEffect, 3f);
- }
- }
- After creating the script, drag the script to the Bomb inspector as a component.
- Lastly we will create an effect for the bomb. To do that go to GameObject and Select Particle System. Particle System is a built in module in Unity for creating a stunning effects for the Game.
- Rename the Particle System as Explosion Effect and set the components of the Particle System as shown below.
- After creating the effect, Create a new Directory inside the Prefabs called FX, this directory will hold all the needed effects for the game. Then drag the effects inside the newly created directory to create a prefab and delete it because we will just spawn the gameobject everytime time the bomb explode.
- Next is attach all the needed Gameobject to the Script of the Bomb and Cannon. After that drag the Player and Bomb gameobject to Player directory to create a prefab, and then delete Bomb in the Scene View after that.
Cannon
Bomb
- Lastly create a Shot indicator that tells how much bomb is left to be shot. To do that go to the GameObject and UI, then select Image. And also a Text UI as a child for the Image and set there component as shown below.
Image
Text
Creating Camera Movement
We will create the camera that follow the bomb when shot. To that first create two GameObject, this will limit the Camera movement around the screen. Then set the component of the newly created gameobject.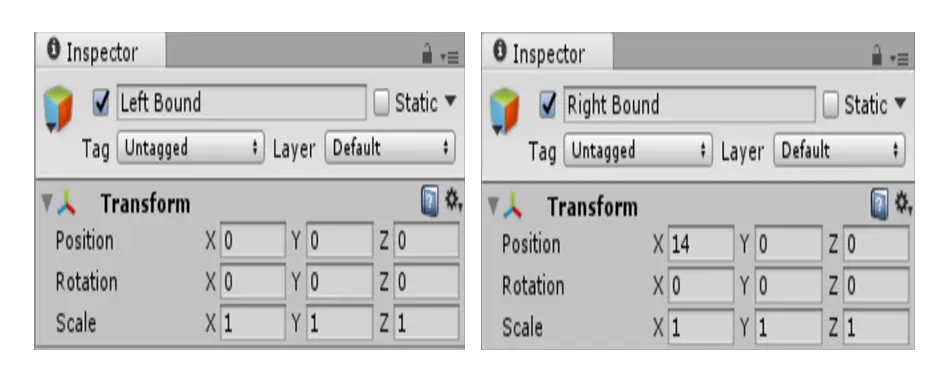
- public Transform target;
- public Transform leftBound;
- public Transform rightBound;
- public float speed = 5f;
- public bool isFollowing;
- private float arrowX;
- public bool allowToMove;
- private float dragSpeed = 0.05f;
- private float timeSinceShot;
- private Vector3 velocity = Vector3.zero;
- private Vector3 startPosition;
- private Vector3 camPos;
- // Use this for initialization
- void Start () {
- startPosition = transform.localPosition;
- }
- // Update is called once per frame
- void Update () {
- if (GameplayController.instance.gameInProgress) {
- if (isFollowing) {
- if (GameObject.FindGameObjectWithTag ("Player Bullet") != null) {
- MoveCameraFollow ();
- }
- } else {
- if (!GameplayController.instance.player.GetChild (0).transform.GetComponent<Cannon> ().readyToShoot) {
- MoveCameraBackToStart ();
- AfterShotMoveAgain ();
- allowToMove = false;
- } else {
- timeSinceShot = 0;
- allowToMove = true;
- }
- }
- if (Application.platform == RuntimePlatform.Android) {
- TouchMoveCamera ();
- } else if (Application.platform == RuntimePlatform.WindowsEditor) {
- MoveCamera ();
- }
- }
- }
- void MoveCameraFollow(){
- if(target != null){
- }
- }
- void MoveCameraBackToStart(){
- transform.position = Vector3.MoveTowards (transform.position, startPosition, Time.deltaTime * 5f);
- }
- void AfterShotMoveAgain(){
- timeSinceShot += Time.deltaTime;
- if (timeSinceShot > 2f) {
- if(startPosition == transform.position){
- GameplayController.instance.player.GetChild (0).transform.GetComponent<Cannon> ().readyToShoot = true;
- }
- }
- }
- void MoveCamera(){
- arrowX = Input.GetAxis ("Horizontal");
- Transform cam = gameObject.transform;
- if (allowToMove) {
- if (arrowX != 0) {
- float camX = cam.position.x;
- camX = Mathf.Clamp (camX, leftBound.transform.position.x, rightBound.transform.position.x);
- }
- }
- }
- void TouchMoveCamera(){
- if(allowToMove){
- /*if(Input.touchCount > 0){
- Touch touch = Input.GetTouch (0);
- Transform cam = gameObject.transform;
- if(touch.phase == TouchPhase.Began){
- cam = touch.position;
- }else if(touch.phase == TouchPhase.Moved){
- }
- }*/
- if(Input.touchCount > 0){
- Touch touch = Input.GetTouch (0);
- Transform cam = gameObject.transform;
- if (touch.position.x > 300) {
- if(touch.phase == TouchPhase.Began || touch.phase == TouchPhase.Stationary){
- camPos = touch.position;
- }else if(touch.phase == TouchPhase.Moved){
- cam.position = cam.position + (new Vector3 ((camPos.x - touch.position.x), 0, 0) * dragSpeed * Time.deltaTime);
- float camX = cam.position.x;
- camX = Mathf.Clamp (camX, leftBound.transform.position.x, rightBound.transform.position.x);
- }
- }
- }
- }
- }
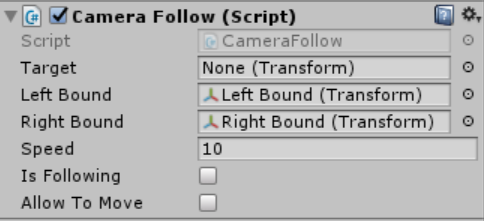
Note: Due to the size or complexity of this submission, the author has submitted it as a .zip file to shorten your download time. After downloading it, you will need a program like Winzip to decompress it.
Virus note: All files are scanned once-a-day by SourceCodester.com for viruses, but new viruses come out every day, so no prevention program can catch 100% of them.
FOR YOUR OWN SAFETY, PLEASE:
1. Re-scan downloaded files using your personal virus checker before using it.
2. NEVER, EVER run compiled files (.exe's, .ocx's, .dll's etc.)--only run source code.
Add new comment
- 221 views