Count Number of Words using Regex in VB.NET
Submitted by donbermoy on Tuesday, June 10, 2014 - 21:24.
Today in VB.NET, we will create again a program that counts the number of words in VB.NET. But here, we will now use Regex. I already made a tutorial about how to create this program but i used Split method with (CChar(" ")) that time. See here: Word Count Program in VB.NET
Now, let's start this tutorial!
1. Let's start with creating a Windows Form Application for this tutorial by following the following steps in Microsoft Visual Studio: Go to File, click New Project, and choose Windows Application.
2. Next, add one TextBox named TextBox1 and a Button named Button1 labeled as "Count Number of Words". You must design your interface like this:
3. Import System.Text.RegularExpressions because we will use Regex syntax here.
4. Now put add this code for your code module. This code is for Button1_Click. This will trigger to count the number of words in your TextBox.
Initialize variable txt as string that will hold the inputted value of our textbox1.
Instantiate a Regex variable named parser that has \w+. A / as an escaped character indicates to match with a character, w means to match any word character, and + here indicates to match the previous element more times.
Declare variable totalMatches As Integer that will match the parser variable using Matches and count method in regex.
Display the number of words and put toString method to make the totalMatches as string.
Full source code:
Output:
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
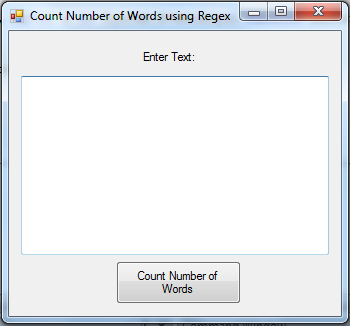
- Imports System.Text.RegularExpressions
- Dim txt As String = TextBox1.Text
- Dim parser As New Regex("\w+")
- Dim totalMatches As Integer = parser.Matches(txt).Count
- MessageBox.Show("Number of words: " & _
- totalMatches.ToString)
- Imports System.Text.RegularExpressions
- Public Class Form1
- Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
- Dim txt As String = TextBox1.Text
- Dim parser As New Regex("\w+")
- Dim totalMatches As Integer = parser.Matches(txt).Count
- MessageBox.Show("Number of words: " & _
- totalMatches.ToString)
- End Sub
- End Class
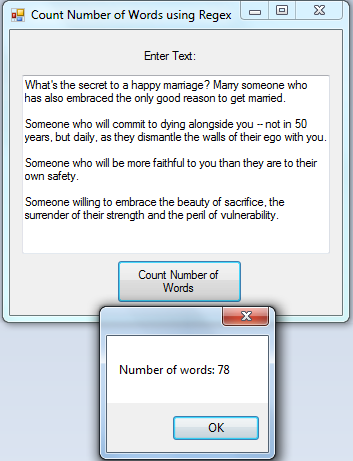