Rolling Numbers Game in Visual Basic 2008
Submitted by janobe on Thursday, April 24, 2014 - 11:34.
This time I will teach you how to create Rolling Numbers Game in Visual Basic 2008. In these features the numbers are rolled randomly and it has a Progress Bar that serves as a timer.
Let's begin:
Open the Visual Basic 2008, create a Windows Application and do the Form just like this.
Double click the Timer and do the following code. The event is, everytime the timer ticks, the numbers in the Textbox will randomly roll. And if the progress bar reached its maximum value, the timer and the number in the Textbox will stop.
Go back to the Design Views, double click the Button and do the following code for starting the timer and assigning the Progress Bar of a minimum value.
The complete source code is included and you can dowload it.
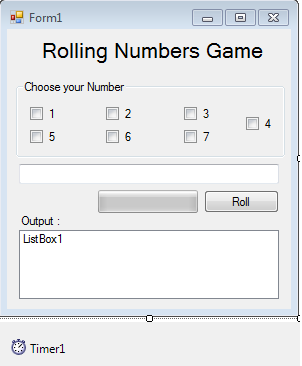
- Private Sub Timer1_Tick(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Timer1.Tick
- 'DECLARING A RANDOM VARIABLE TO STORE THE NUMBERS ON IT.
- 'IT REPRESENTS A PSUEDO RANDOM GENERATOR.
- Dim num As Random = New Random()
- 'DECLARING AN INTEGER VARIABLE TO STORE THE NONNEGATIVE RANDOM NUMBERS.
- 'STARTS WITH THE MINIMUM VALUE TO THE MAXIMUM VALUE
- 'NEXT REPRESENTS TO RETURN A RANDOM NUMBER WITHIN A SPECIFIED RANGE.
- Dim result As Integer = num.Next(1, 7)
- 'PUT THE RESULT IN THE TEXTBOX.
- TextBox1.Text = result.ToString
- 'PROGRESSBAR INCREASED BY 1.
- ProgressBar1.Value += 1
- 'THE CONDITION IS, WHEN THE PROGRESSBAR VALUE REACHED TO 100
- 'THEN THE TIMER WILL STOP AND THE NUMBER IN THE TEXTBOX WILL STOP ROLLING TOO.
- If ProgressBar1.Value = 100 Then
- 'FILTERING THE CHECKBOX.
- 'IF THE CHECKBOX1 IS CHECKED THE MESSAGE WILL APPEAR IN THE LISTBOX.
- If CheckBox1.CheckState = CheckState.Checked Then
- If TextBox1.Text = CheckBox1.Text Then
- 'ADD THE STRING MESSAGE IN THE LISTBOX.
- ListBox1.Items.Add("The number " & TextBox1.Text & " won!")
- End If
- End If
- If CheckBox2.CheckState = CheckState.Checked Then
- If TextBox1.Text = CheckBox2.Text Then
- ListBox1.Items.Add("The number " & TextBox1.Text & " won!")
- End If
- End If
- If CheckBox3.CheckState = CheckState.Checked Then
- If TextBox1.Text = CheckBox3.Text Then
- ListBox1.Items.Add("The number " & TextBox1.Text & " won!")
- End If
- End If
- If CheckBox4.CheckState = CheckState.Checked Then
- If TextBox1.Text = CheckBox4.Text Then
- ListBox1.Items.Add("The number " & TextBox1.Text & " won!")
- End If
- End If
- If CheckBox5.CheckState = CheckState.Checked Then
- If TextBox1.Text = CheckBox5.Text Then
- ListBox1.Items.Add("The number " & TextBox1.Text & " won!")
- End If
- End If
- If CheckBox6.CheckState = CheckState.Checked Then
- If TextBox1.Text = CheckBox6.Text Then
- ListBox1.Items.Add("The number " & TextBox1.Text & " won!")
- End If
- End If
- If CheckBox7.CheckState = CheckState.Checked Then
- If TextBox1.Text = CheckBox7.Text Then
- ListBox1.Items.Add("The number " & TextBox1.Text & " won!")
- End If
- End If
- Timer1.Stop()
- End If
- End Sub
- Private Sub btnRoll_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnRoll.Click
- 'CLEARING THE LISTBOX
- ListBox1.Items.Clear()
- 'timer starts
- Timer1.Start()
- 'PROGRESSBAR VALUE RETURNS TO 0 EVERYTIME YOU CLICK THE BUTTON
- ProgressBar1.Value = 0
- End Sub
Add new comment
- 97 views