Digital Stopwatch using Visual Basic.Net
Submitted by joken on Sunday, September 8, 2013 - 16:53.
In this tutorial I'm going to teach you on how to create a simple digital stopwatch that will able you to start the timer when the “Start” button is click, then pause the timer when the “Pause” button is clicked. And you can also clicke the “Continue” button if you want to continue running the timer. And this program has the feature that able’s you to restart the timer anytime you want as long as you click the “Reset” button and finally you can stop the timer if you wish to stop it and the timer will go back to its original value. Below is the sample output in this code.
To start building our application open visual basic in your computer and create a new project and name it as "Digital Stopwatch". And then add five (5) buttons in your form: one textbox, one label and one timer. After this design your user interface that looks as shown above. And after designing the user interface, let's proceed in adding a functionality to our application.
First double click the form to show the code view and below Public class add this declaration of variables.
Then we will create a sub procedure for running the timer and we will name this sub as “runningtime” and here’s it looks like.
After this, we need also to create another two sub procedure that is responsible for stopping and restarting the timer and name this as “stop time” and “restart”. Here's the code for these two procedures.
Since we have already set our sub procedures lets now add code to our timer. To do this double click our timer and add this single code.
Next we need to add this code to our form load and these how it looks like.
Next we will add code also for “Start” button and here’s the code.
For our “Stop” button.
And for “Reset” button.
And then for our “Pause” button.
And finally our “Exit” button.
Then after completing all the steps you can now start to test your program. That’s it for now fellas see you on my next tutorial focusing on manipulating the time using “Timer control”. Thanks for reading.
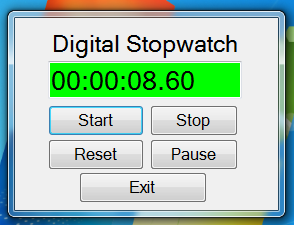
- Dim a As String
- Dim b As String
- Dim c As String
- Dim d As String
- Dim y As String
- Dim z As String
- Dim h As String
- Dim m As String
- Dim s As String
- Dim u As String
- Dim v As String
- Sub runningtime()
- v = v + 1
- txttime.Text = a + b + ":" + c + d + ":" + y + z + "." + u + v
- 'check if u is less then 9
- v = 0
- 'incretment u by 1
- u = u + 1
- 'display for miliseconds
- txttime.Text = a + b + ":" + c + d + ":" + y + z + "." + u + v
- 'check if z is still less than 9
- 'set the milisecond to 0
- v = 0
- u = 0
- 'increment
- z = z + 1
- 'display for second(s)
- txttime.Text = a + b + ":" + c + d + ":" + y + z + "." + u + v
- v = 0
- u = 0
- z = 0
- y = y + 1
- txttime.Text = a + b + ":" + c + d + ":" + y + z + "." + u + v
- v = 0
- u = 0
- z = 0
- y = 0
- d = d + 1
- txttime.Text = a + b + ":" + c + d + ":" + y + z + "." + u + v
- v = 0
- u = 0
- z = 0
- y = 0
- d = 0
- c = c + 1
- txttime.Text = a + b + ":" + c + d + ":" + y + z + "." + u + v
- v = 0
- u = 0
- z = 0
- y = 0
- d = 0
- c = 0
- b = b + 1
- txttime.Text = a + b + ":" + c + d + ":" + y + z + "." + u + v
- v = 0
- u = 0
- z = 0
- y = 0
- d = 0
- c = 0
- b = b + 1
- txttime.Text = a + b + ":" + c + d + ":" + y + z + "." + u + v
- v = 0
- u = 0
- z = 0
- y = 0
- d = 0
- c = 0
- b = 0
- a = a + 1
- 'this is the final output if reach the hour value
- txttime.Text = a + b + ":" + c + d + ":" + y + z + "." + u + v
- '1 sec = 1000ms
- '1 min = 60000ms
- '1 hr = 36000000ms
- End If
- End Sub
- Sub restart()
- a = "0"
- b = "0"
- c = "0"
- d = "0"
- y = "0"
- z = "0"
- u = "0"
- v = "0"
- h = a + b
- m = c + d
- s = y + z
- End Sub
- Sub stoptime()
- Timer1.Enabled = False
- a = "0"
- b = "0"
- c = "0"
- d = "0"
- y = "0"
- z = "0"
- u = "0"
- v = "0"
- h = a + b
- m = c + d
- s = y + z
- 'display as "00:00:00.00"
- txttime.Text = h + ":" + m + ":" + s + "." + u + v
- End Sub
- Private Sub Timer1_Tick(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Timer1.Tick
- 'this single code, it simply call the runningtime() sub procedure
- runningtime()
- End Sub
- Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
- 'it do the initialization
- a = "0"
- b = "0"
- c = "0"
- d = "0"
- y = "0"
- z = "0"
- u = "0"
- v = "0"
- h = a + b
- m = c + d
- s = y + z
- 'display as "00:00:00.00"
- txttime.Text = h + ":" + m + ":" + s + "." + u + v
- End Sub
- Private Sub BtnStart_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles BtnStart.Click
- 'start the timer
- Timer1.Start()
- 'check if the text of these button is set to continue set by pause button then it set to start
- If BtnStart.Text = "Continue" Then
- BtnStart.Text = "Start"
- End If
- End Sub
- stoptime()
- restart()
- Timer1.Enabled = False
- BtnStart.Text = "Continue"