Dynamic Web Landing Page using JavaScript and jQuery Free Source Code
Introduction
This is a mini-project called Dynamic Web Landing Page. I am sharing this simple source code developed in HTML, CSS, JavaScript and jQuery to give the new programmers or students a reference of manipulating the HTML Elements. The source code outputs a simple site landing page that contains dynamic content which are the date, time, and some other texts. The data are being stored using the web browser's Local Storage.
About the Dynamic Web Landing Page
I developed this project using the following:
- HTML
- CSS
- JavaScript
- jQuery
This Dynamic Web Landing Page changes the background and images are depending to current date. Background Images changes to Dawn, Morning, Afternoon, and Dusk. Users can update the Name of the person to greet and 'Today's Up' text content. The digital clock shown on the page automatically updates every second. Date time is based on the clients' date and time. Users can also override the manipulation logic, by clicking the provided buttons located at the bottom of the page. When the user clicks the button, the DOM Manipulation will be based on the selected button and it will provide a static sample time. Users can also get back to the live time by selecting the 'Live' button.
Features
- DOM Manipulation
- Live Digital Clock and Date
- Editable Name to Greet
- Editable Text Content
- DOM Manipulation Override Buttons
The following script is a JS code that manipulating the digital clock.
- function date_time() {
- const date_now = new Date();
- const year = date_now.getFullYear()
- const month = date_now.getMonth()
- const day = date_now.getDate()
- const wday = date_now.getDay()
- const hour = date_now.getHours()
- const mins = date_now.getMinutes()
- const sec = date_now.getSeconds()
- const months = ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sept', 'Oct', 'Nov', 'Dec']
- const days = ['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday']
- const timeAbbr = hour >= 12 ? "PM" : "AM";
- hr = hour % 12 || 12;
- if (hour == 5 && mins <= 35) {
- $('body').css('background-image', 'var(--dawn)')
- } else if (hour >= 6 && hour < 12) {
- $('body').css('background-image', 'var(--morning)')
- $('body').css('color', 'var(--light-font-color)')
- } else if (hour >= 12 && hour < 17) {
- $('body').css('background-image', 'var(--afternoon)')
- $('body').css('color', 'var(--light-font-color)')
- } else if (hour == 18) {
- $('body').css('background-image', 'var(--dusk)')
- $('body').css('color', 'var(--light-font-color)')
- } else if (hour > 18) {
- $('body').css('background-image', 'var(--evening)')
- $('body').css('color', 'var(--light-font-color)')
- }
- if (hour < 12) {
- greetings.text('Good Morning, ')
- } else if (hour < 18) {
- greetings.text('Good Afternoon, ')
- } else if (hour >= 18) {
- greetings.text('Good Evening, ')
- }
- time = String(hr).padStart(2, 0) + ":" + String(mins).padStart(2, 0) + ":" + String(sec).padStart(2, 0) + "<br/>" + timeAbbr
- dd = days[wday] + ", " + months[month] + " " + day + ", " + year
- clock.html(time)
- date.text(dd)
- }
The source code was developed only for educational purposes only. You can download the source code for free and modify it the way you wanted.
Sample Snapshot of the Application
Dawn
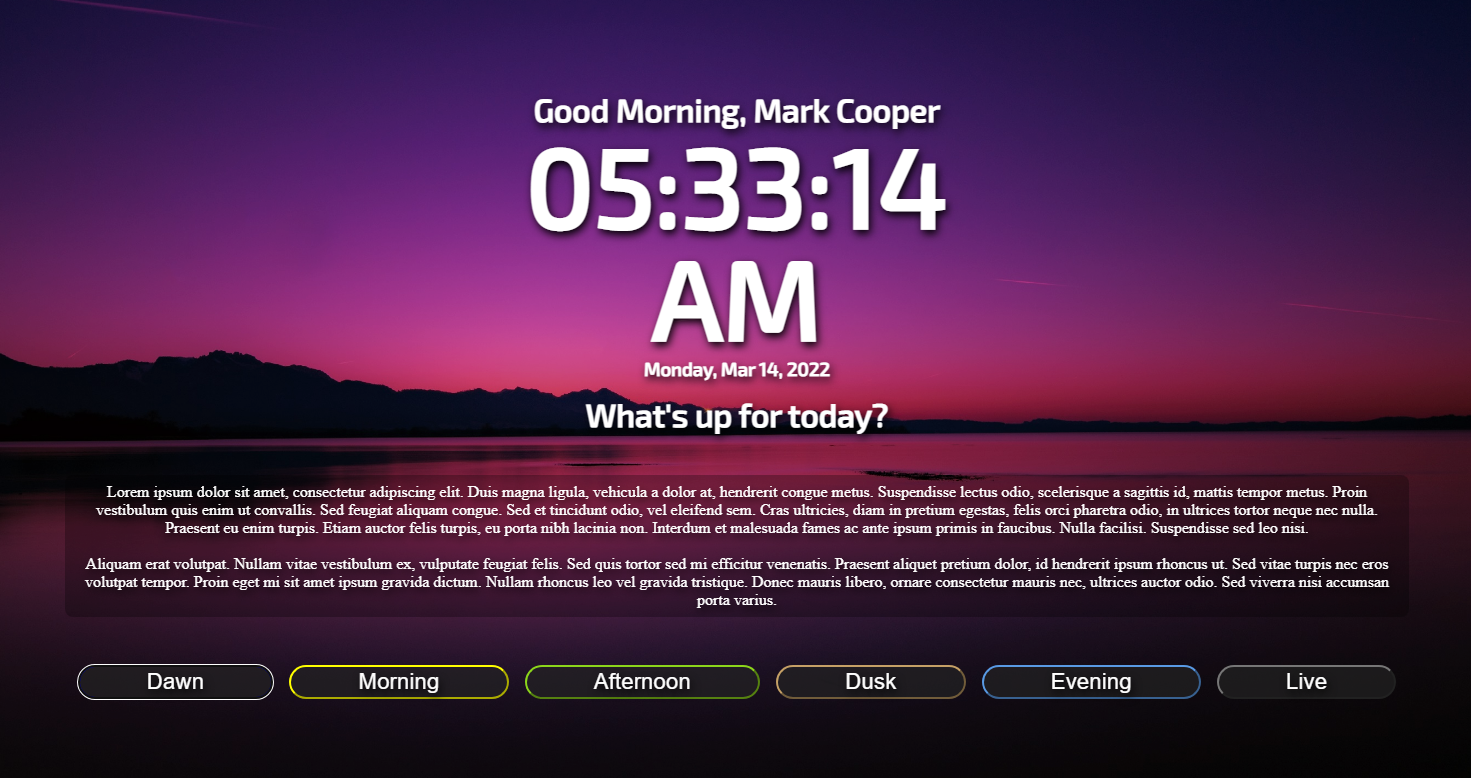
Morning
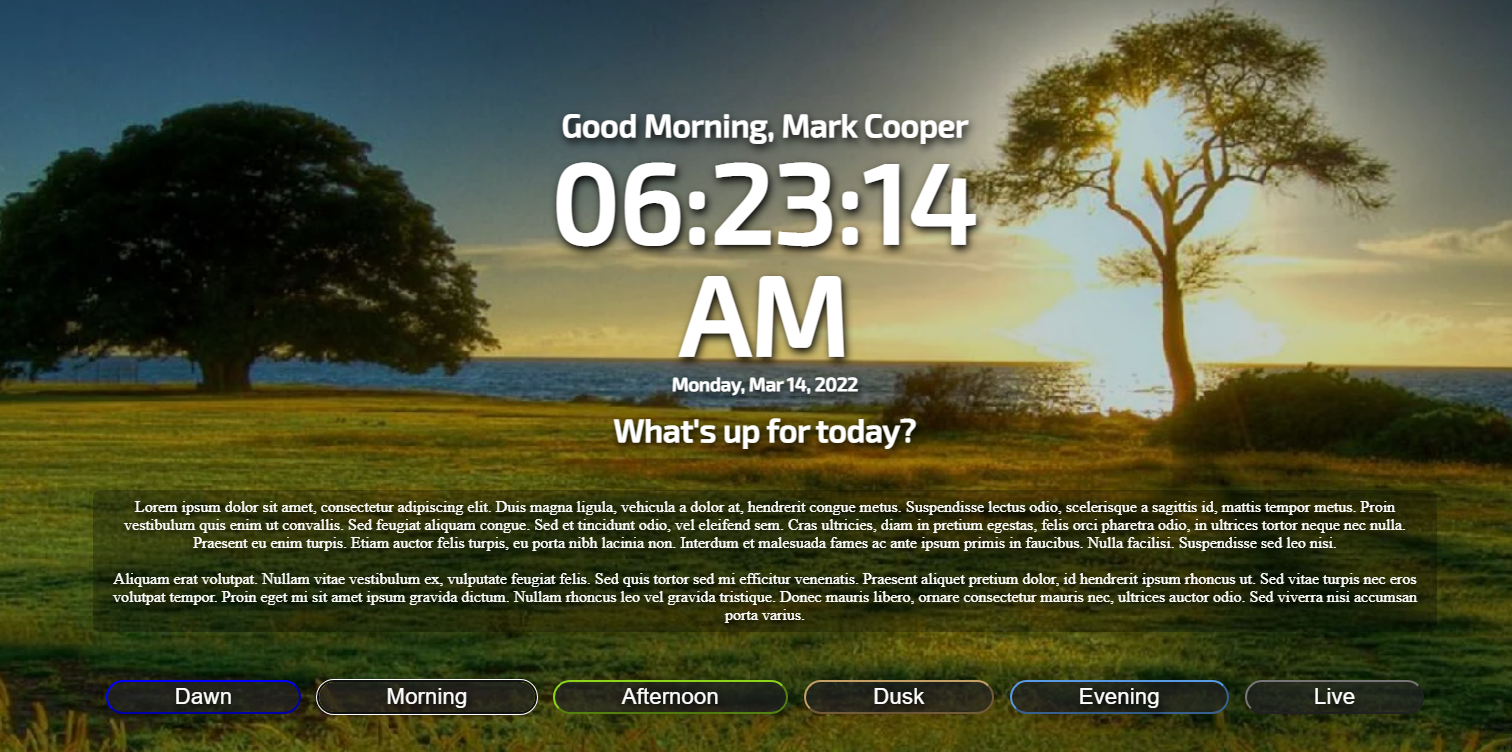
Afternoon
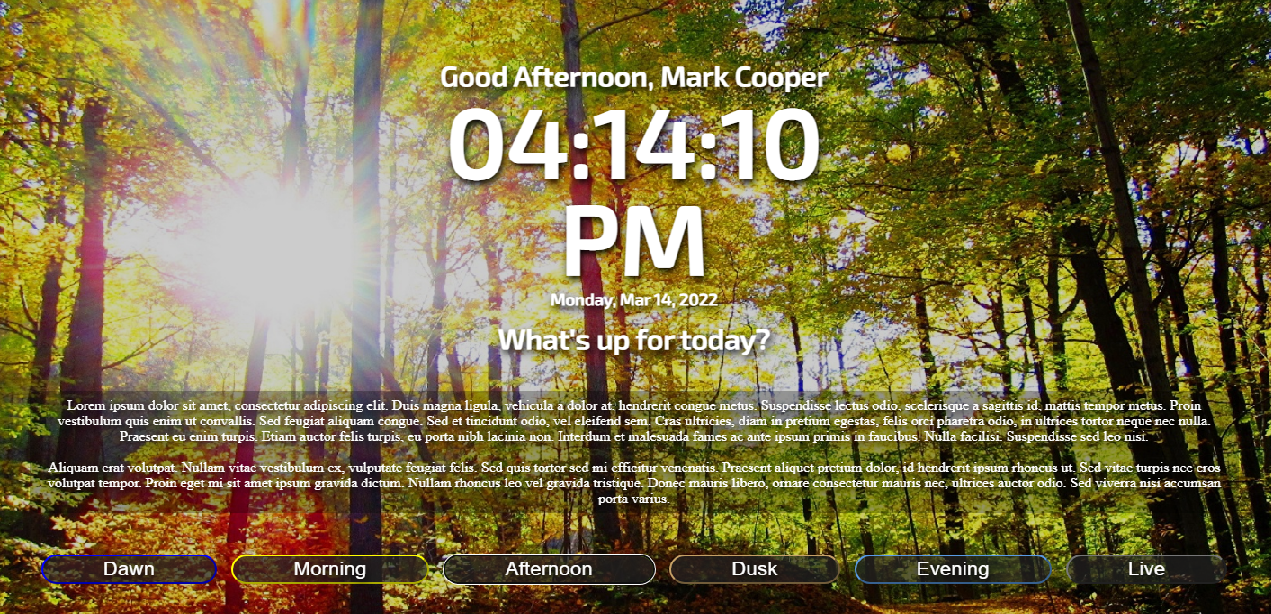
Dusk
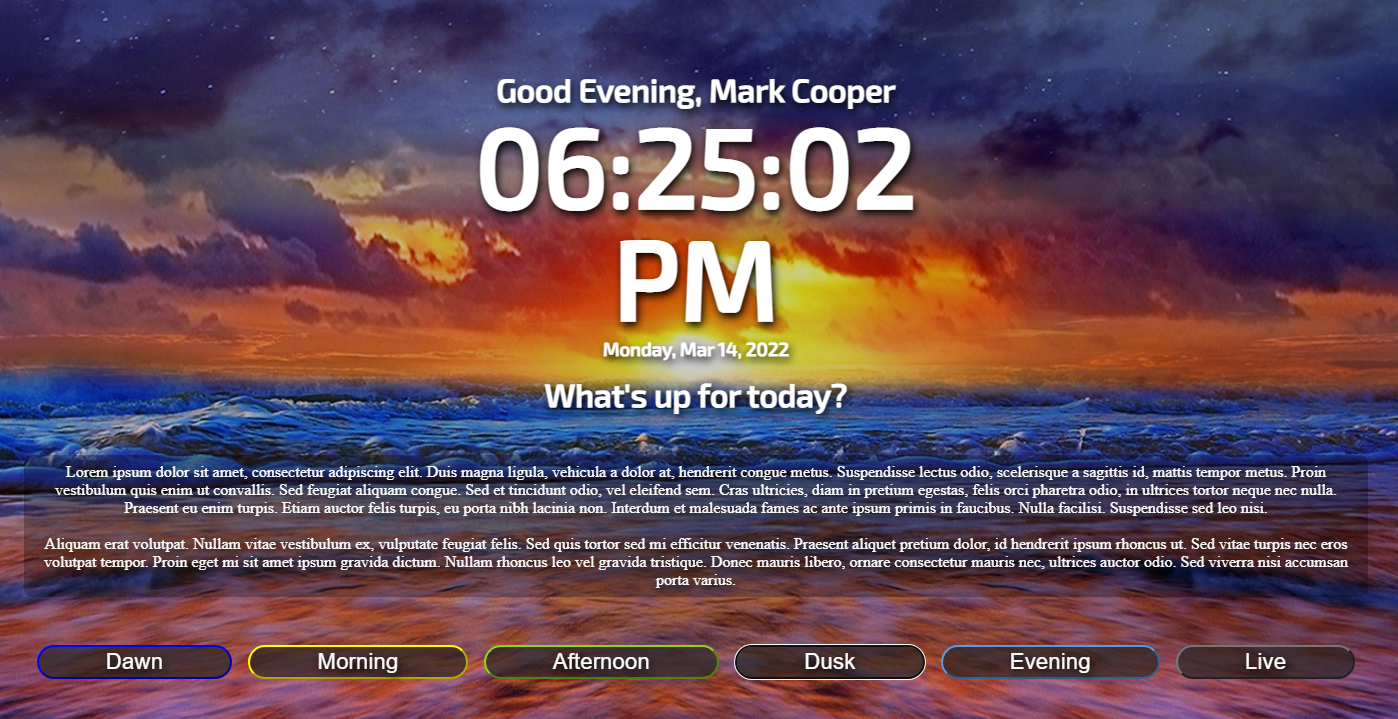
Evening
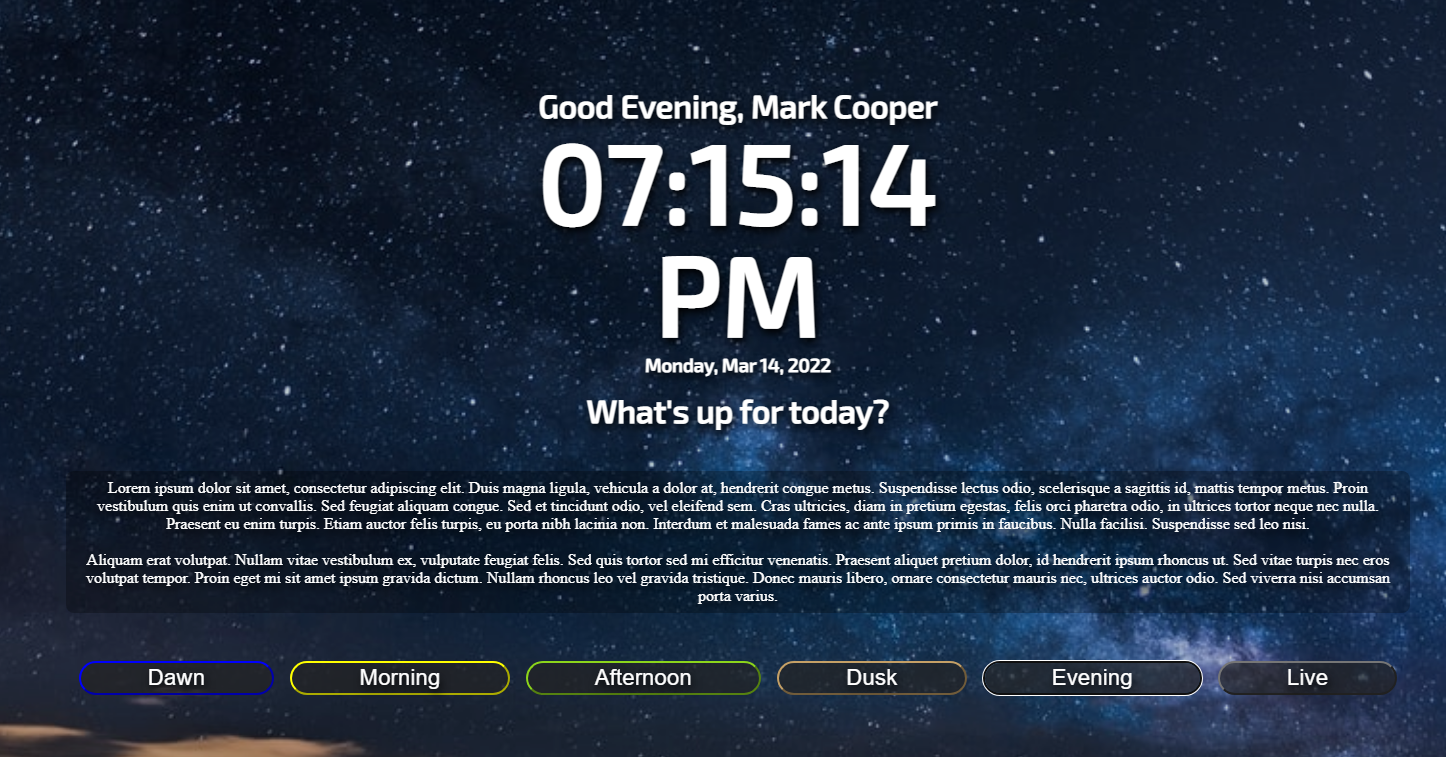
How to Run ??
- Download the provided source code zip file. (download button is located below)
- Extract the downloaded source code zip file in your desired location.
- Locate the index.html file inside the extracted source code folder.
- Open the index.html file in your preferred web browser.
- Or, Browse the Dynamic Web Landing Page in a browser. i.e. file:///C:/users/default/desktop/landing_page/index.html
DEMO VIDEO
That's it. I hope this simple Dynamic Web Landing Page in JavaScript will help you with what you are looking for and you'll find something useful for your future web application projects.
Explore more on this website for more Free Source Codes and Tutorials.
Enjoy :)
Note: Due to the size or complexity of this submission, the author has submitted it as a .zip file to shorten your download time. After downloading it, you will need a program like Winzip to decompress it.
Virus note: All files are scanned once-a-day by SourceCodester.com for viruses, but new viruses come out every day, so no prevention program can catch 100% of them.
FOR YOUR OWN SAFETY, PLEASE:
1. Re-scan downloaded files using your personal virus checker before using it.
2. NEVER, EVER run compiled files (.exe's, .ocx's, .dll's etc.)--only run source code.
Add new comment
- 1325 views