A Beginner’s Guide to Asymptotic Analysis of Algorithms
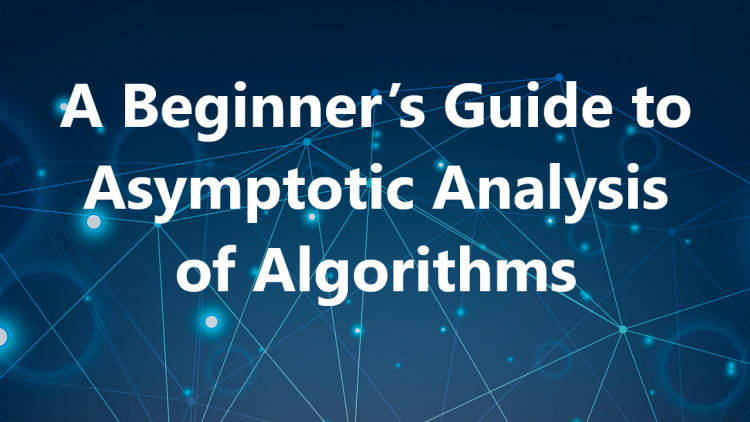
In this beginner's guide to asymptotic analysis of algorithms, we aim to make the concept easily understandable, even for those who are new to algorithmic analysis and might not be well-versed in mathematical jargon.
Asymptotic analysis is a way of gauging the efficiency of algorithms without diving into intricate details. It focuses on how an algorithm's performance changes as the input size grows substantially. Think of it as assessing how well your code handles increasingly larger problems.
Suppose you want to compare the fuel efficiency of two cars on long-distance journeys. Instead of measuring the exact fuel consumption for every mile, you'd likely consider how their fuel efficiency behaves when driving extremely long distances, like 1,000 miles or 10,000 miles. This general perspective gives you an idea of which car is better for extended trips.
Similarly, in the world of computing, we often need to know how an algorithm performs when dealing with massive amounts of data. Asymptotic analysis helps us by using straightforward notations like "Big O" to describe how an algorithm's time or space requirements change as the input size becomes very large. It focuses on the most significant part of the algorithm's behavior.
For instance, if an algorithm's time complexity is expressed as O(n^2), it means that when you double the input size, the time it takes to run the algorithm will increase fourfold. On the other hand, if another algorithm has a time complexity of O(n), doubling the input size will approximately double the runtime. This type of analysis provides a high-level view of an algorithm's efficiency without requiring precise measurements for specific input sizes.
Asymptotic analysis aids programmers and computer scientists in making informed choices when selecting algorithms for their tasks. It provides a simple way to understand how an algorithm's efficiency behaves as the problem size increases, without getting bogged down in specific numbers or implementation details.
Efficiency in algorithm design is crucial because it influences how quickly a program can complete its tasks and how many computational resources it consumes. By considering various factors, such as time complexity, space complexity, best-case, average-case, and worst-case scenarios, constant factors, input sensitivity, caching, parallelization, algorithmic paradigms, real-world constraints, and benchmarking, you can choose or design algorithms that meet your requirements while using resources effectively.
In the realm of asymptotic analysis, three notations—Big O, Omega, and Theta—play a significant role in describing how algorithms or functions behave:
-
Big O Notation (O): Think of Big O as an upper bound, indicating the worst-case scenario for an algorithm's performance. It answers the question: "In the worst situation, how much time will our algorithm take?" For example, O(n) signifies that the time taken by the algorithm grows linearly with the input size.
-
Omega Notation (Ω): In contrast, Omega serves as a lower bound, representing the best-case scenario for an algorithm's performance. It answers the question: "No matter what, how fast can our algorithm run?" For instance, Ω(1) implies that the algorithm will always take at least constant time.
-
Theta Notation (Θ): Theta notation offers a balanced view, presenting a tight bound on an algorithm's performance. It answers the question: "How does our algorithm perform in both the best and worst cases?" If an algorithm is Θ(n), it indicates that its performance grows linearly with the input size in both the best and worst scenarios.
These notations simplify the communication of efficiency and performance characteristics of algorithms without delving into intricate details.
To analyze the asymptotic complexity of an algorithm, follow these straightforward steps:
-
Identify the Input Size: Determine what aspect of the algorithm's efficiency you're evaluating, often the input size, like the number of elements in a list.
-
Count Basic Operations: Break down the algorithm into its fundamental operations. For example, if you're sorting a list, count the number of times you compare two elements or swap them.
-
Focus on the Worst Case: Analyze the algorithm's behavior in the worst-case scenario, where the input is most challenging for the algorithm. This provides insight into the algorithm's worst performance.
-
Remove Constants: Eliminate constant factors from your analysis. Concentrate on the most significant factors that affect the algorithm's performance.
-
Use Big O Notation: Express the algorithm's complexity using "Big O" notation, describing how the algorithm's runtime or memory usage grows as the input size increases. For example, if doubling the input size approximately doubles the runtime, it might be expressed as O(n).
-
Compare with Other Algorithms: Finally, compare the results with other algorithms to determine which one is more efficient. In general, the algorithm with a lower Big O notation runs faster.
By following these steps, you can assess the efficiency of an algorithm without getting lost in technical details, helping you make informed decisions in selecting the right algorithms for your projects.
Conclusion
In conclusion, understanding how algorithms perform as problems get bigger is important for making software work better and faster. Asymptotic analysis helps us do that. We saw that it's like comparing cars for long trips, but for computer programs. It's not too complicated and can help you make your code smarter and faster.
We also learned about Big O, Omega, and Theta notations, which describe how algorithms work in different situations. Big O tells us the worst-case scenario, Omega is the best-case, and Theta gives a balanced view.
When you want to analyze an algorithm's efficiency, you follow some simple steps: Identify the input size, count basic operations, focus on the worst case, ignore constants, use Big O notation, and compare with other algorithms. It's like comparing cars for a long trip – you want to know which one is best. These steps can help you choose the best algorithm for your needs without getting into complex math.
So, by understanding asymptotic analysis, you can make your code work faster and use less memory, which is great for any programmer, whether you're a student, a self-taught coder, or just curious about how software works.
Add new comment
- 139 views