Exploring the Common Design Techniques of Algorithms: A Comprehensive Guide
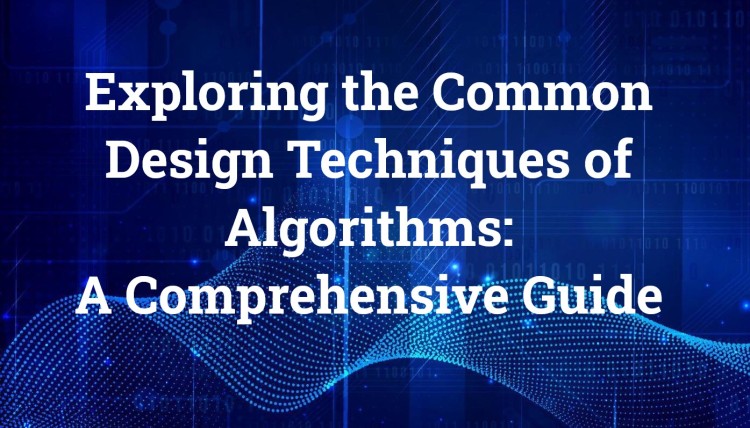
Welcome to our comprehensive guide on algorithm design techniques. In this article, we will delve into the common principles that underpin the creation of effective algorithms. Algorithms are like the recipes of the digital world, guiding computers to perform various tasks. Whether you're a beginner or an experienced coder, understanding these essential design techniques will empower you to tackle problems, streamline processes, and write efficient code. So, let's embark on this journey together, demystifying the world of algorithms and equipping you with the knowledge you need to become a better problem solver and developer.
Algorithm design is the process of creating a set of step-by-step instructions or a plan for solving a specific problem or performing a task. It involves thinking through how to solve a problem efficiently and effectively, so that a computer or any other machine can follow the instructions and produce the desired outcome.
In simple terms, algorithm design is like giving a clear, precise recipe for a computer to follow. Just as a recipe tells you exactly what ingredients to use and the steps to follow in cooking a meal, an algorithm provides a computer with a clear set of instructions to complete a task.
Good algorithm design aims to find the most efficient and effective way to solve a problem, which can save time and resources. It often involves breaking down a complex problem into smaller, more manageable steps, ensuring that the instructions are easy to understand and follow. Algorithm design is a fundamental skill in computer science and is used in various applications, from sorting data to finding routes on maps, and much more.
There are many different algorithm design techniques, each with its own strengths and weaknesses. Some of the most common techniques include:
Brute-force
A brute-force algorithm is a simple and straightforward method for solving problems. It works by trying out all possible solutions, one by one, until it finds the right answer. It's like checking every item in a store to find a specific one, even if it takes a lot of time and effort.
Here's how it works:
- Start with the first possible solution.
- Check if it's the correct answer.
- If it is, great! You're done.
- If not, move on to the next possible solution and repeat steps 2 and 3.
- Keep doing this until you find the right answer or go through all the possibilities.
Brute-force algorithms are simple to understand and can solve many problems, but they can be slow, especially when there are a lot of possibilities to check. So, they're not always the most efficient way to solve problems, but they can be a good starting point when you're trying to figure out a solution.
Divide and Conquer
The "divide and conquer" algorithm design technique is a way to solve big problems by breaking them down into smaller, more manageable pieces.
Here's how it works:
- Divide: First, you split the big problem into smaller, similar sub-problems. This makes it easier to handle.
- Conquer: Next, you solve each of these smaller sub-problems. This might involve further dividing them into even tinier parts until they become simple enough to solve easily.
- Combine: Finally, you put together the solutions of the smaller sub-problems to get the solution to the original big problem.
This technique is like breaking a big task into smaller, more manageable tasks, solving them one by one, and then putting the results together to solve the main task. It's a smart way to tackle complex problems and is commonly used in various fields, including computer science, mathematics, and problem-solving in everyday life.
Greedy Algorithms
A "greedy algorithm" is a way of solving problems step by step, where at each step, you choose the best option available without worrying about the future. It's like making choices that seem good right now, without thinking too far ahead.
Here's how it works:
- Start with an empty solution or an initial state.
- At each step, you have several options or choices. The greedy algorithm selects the option that appears to be the best at that moment based on a specific criterion. This criterion depends on the problem you're trying to solve. It could be choosing the largest or smallest item, the nearest location, the cheapest option, etc.
- After making a choice, you may update your solution or state to reflect that choice. This means you might remove the chosen item from the list, add it to your solution, or adjust some variables.
- Steps 2 and 3 are repeated until you've made all the necessary choices or reached a stopping condition.
- The final solution is the result of all the choices made along the way.
Greedy algorithms are simple and quick, but they might not always give the absolute best solution in the end. Sometimes, a more thoughtful strategy could lead to a better outcome. However, in many cases, greedy algorithms work well and provide a reasonably good solution, making them a handy tool for solving various problems in a straightforward way.
Dynamic Programming
Dynamic programming is a method for solving problems by breaking them down into smaller, simpler parts and storing the solutions to those smaller parts so they can be reused. This technique is often used when solving problems that can be divided into overlapping sub-problems. Instead of solving the same sub-problem multiple times, dynamic programming saves time by solving it once and remembering the answer.
Here's how it works:
- Identify the problem: First, you recognize a problem that can be broken into smaller, overlapping sub-problems. This problem can be a math puzzle, an optimization task, or something else.
- Divide and conquer: Break the main problem into smaller, simpler sub-problems. Solve these sub-problems one by one and store their solutions.
- Memoization: Store the solutions to the sub-problems in a table or array so you can look them up when needed. This saves time by preventing you from solving the same sub-problem multiple times.
- Combine solutions: Use the solutions to the sub-problems to build the solution to the main problem. By reusing the stored solutions, you avoid redundant work and make the process more efficient.
Dynamic programming is especially useful for solving problems with overlapping sub-problems, like many optimization and search problems. It's a handy technique to optimize your problem-solving process and save time and resources.
Backtracking
Backtracking is a problem-solving technique where you try different solutions to a problem and backtrack when you find that your current approach is not working. It's like exploring a maze by taking different paths and going back when you hit a dead end.
Here's how it works:
- You start with a problem that can have multiple solutions, like finding the best route on a map or solving a puzzle.
- You make a choice and follow a path to see if it leads to a solution.
- If it doesn't work or you get stuck, you backtrack to the previous step and try a different choice.
- You keep repeating this process until you find a solution or explore all possible options.
Backtracking is often used for problems where you don't know the solution in advance, and you need to try different combinations or options to figure it out. It's a trial-and-error method that helps you find the right answer by exploring different paths and eliminating those that don't work.
Randomized Algorithms
A randomized algorithm design technique involves introducing randomness or chance into the algorithm's process to make it work better for certain types of problems. Instead of following a fixed set of steps every time, a randomized algorithm makes some decisions randomly. This randomness can lead to more efficient solutions or faster results in some cases.
Here's how it works:
- Start with a problem that's hard to solve using a regular, non-random approach.
- Introduce randomness by making some random decisions during the problem-solving process.
- Repeat these random steps multiple times to increase the chances of finding a good solution.
- Finally, use the best result among the random outcomes as the solution to the problem.
Randomized algorithms are particularly useful when dealing with problems where exact, deterministic solutions are difficult or time-consuming to find. By incorporating randomness, these algorithms can often achieve their goals more quickly, and the randomness can help in avoiding worst-case scenarios.
Think of it like a game of chance – sometimes you might win big, and other times you might not do as well, but over many rounds, you can expect to achieve good results on average.
Parallel Algorithms
Parallel algorithm design is a way to make computer programs run faster by doing multiple tasks at the same time. Instead of doing things one after the other, parallel algorithms split the work into smaller parts and do them simultaneously. It's like having many workers working together to finish a big job more quickly.
Here's how it works:
- The first step is to break down the main task into smaller, independent subtasks. For example, if you have a big list of numbers to sort, you can split the list into smaller sections.
- Each subtask is assigned to a different worker, which could be a separate core in a multi-core processor or even a completely different computer in a network. Each worker knows what to do with its assigned subtask.
- All the workers start working on their assigned subtasks simultaneously. They don't need to wait for one worker to finish before another one starts. This is what makes it "parallel".
- Sometimes, the workers need to communicate and share information to complete the overall task. For example, in the sorting example, they might need to exchange sorted sections to merge them into a fully sorted list.
- Once all the workers have completed their subtasks, the results are combined to produce the final outcome of the main task. In our sorting example, the sorted sub-lists are merged together to create the fully sorted list.
The idea behind parallel algorithm design is to save time and increase efficiency. By doing multiple things at the same time, you can often get your task done faster, especially when dealing with tasks that can be split into independent parts. This approach is commonly used in modern computing to take full advantage of the available processing power.
Branch and Bound
The "branch and bound" algorithm design technique is a way to solve complex problems by breaking them into smaller parts and systematically exploring possible solutions. It's like dividing a big puzzle into smaller pieces to find the best solution more efficiently.
Here's how it works:
- Branching: The algorithm starts with an initial solution and explores different choices or branches to improve it. This is like trying out different paths in a maze to find the way out.
- Bounding: At each step, the algorithm calculates a bound or estimate to figure out if it's worth exploring a particular branch further. It can discard branches that are unlikely to lead to a better solution, saving time and effort.
- Pruning: When a branch is proven to be less promising than the current best solution, it's pruned or cut off. This helps focus the search on more promising branches, similar to eliminating dead-end paths in a maze.
By repeatedly branching, bounding, and pruning, the algorithm homes in on the best solution step by step. It's commonly used for optimization problems where you're trying to find the best solution from many possibilities, such as scheduling tasks or finding the shortest route.
Overall, the branch and bound technique is a structured way to efficiently explore different options and find the best solution for complex problems.
In conclusion, algorithm design is a fundamental aspect of computer science and problem-solving, enabling us to create efficient step-by-step instructions for solving various tasks. We've explored several common algorithm design techniques that cater to different problem-solving scenarios. These techniques include brute-force, divide and conquer, greedy algorithms, dynamic programming, backtracking, randomized algorithms, parallel algorithms, and branch and bound. Each of these methods has its own strengths and weaknesses, making them valuable tools for tackling diverse problems.
These techniques provide a structured approach to problem-solving, whether you're looking for the best solution, optimizing processes, or navigating complex scenarios. They empower both beginners and experienced developers to craft algorithms that save time and resources while ensuring that computer systems produce desired outcomes.
By understanding and applying these fundamental algorithm design techniques, you'll be better equipped to address a wide range of challenges in the digital world. As you embark on your journey as a problem solver and developer, these techniques will be valuable tools in your toolkit, helping you streamline processes and write efficient code.
Add new comment
- 193 views