Python AttributeError: 'str' object has no attribute 'read' [Solved]
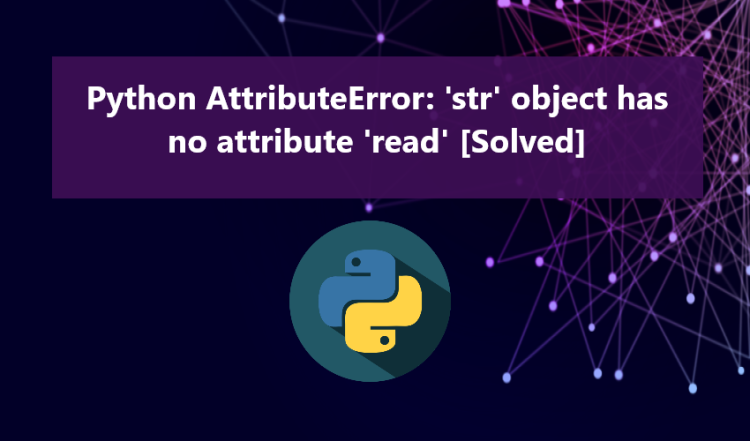
In this article, we will explore the causes and potential solutions for the Python AttributeError that specifically appears as AttributeError: 'str' object has no attribute 'read'. If you are new to Python programming and encountering this error during your project development, this article will provide you with insights into the reasons behind this issue and guide you on how to resolve it.
What is `AttributeError
` in Python?
In Python, an AttributeError
represents an exception that occurs when you attempt to access an attribute or method of an object that is either nonexistent or inaccessible. This error is a frequent occurrence in the Python programming language and is typically resolved by ensuring that the attributes you're trying to access exist and are correctly spelled. Apart from the error we'll address in this article, AttributeError
can also manifest with error messages like the following:
- AttributeError: module 'datetime' has no attribute 'now'
- AttributeError: 'list' object has no attribute 'lower'
- AttributeError: 'DataFrame' object has no attribute 'concat'
For further information about the Python errors mentioned above, you can click the error messages themselves to navigate to their respective dedicated articles.
Why does the Python `AttributeError: 'str' object has no attribute 'read'` occur?
The Python AttributeError: 'str' object has no attribute 'read'
error occurs when you try to utilize or access the read()
method or attribute on a string object. The read()
method is commonly employed for reading content or data from a file object and is not intended for use with strings.
Here are the typical causes of this error:
- Direct File Content Reading: This error occurs when trying to read file content directly using the
read()
method on a filename string object. - Using the JSON Built-in Library: The error can also arise when attempting to load file data using
json.load()
without first opening the file.
Example #1
Here's a basic Python code snippet that replicates the error when you try to directly read the file content from a filename string object.
- FileName = "data.json"
- data = FileName.read()
- print(data)
The AttributeError: 'str' object has no attribute 'read'
occurs when you try to read file content from a filename string object without correctly opening the file.
To resolve this error, you can easily fix it by using the open()
method to open the file and set it as a file object, which will enable you to use the read()
method.
Solution
- FileName = "data.json"
- with open(FileName, 'r') as file:
- data = file.read()
- print(data)
Example #2
In Python, you can also load file content, particularly JSON data, using the built-in library called json
and its load()
method. Implementing this method is one of the reasons why the AttributeError: 'str' object has no attribute 'read'
error occurs. Here's the script below that demonstrates the usage of json.load()
and replicates the error:
- import json
- FileName = "data.json"
- dataJSON = json.load(FileName)
Similar to the first example, the reason for this error is trying to read the filename string object as if it were a file object. To resolve this issue, you can employ the same approach as in the initial example, which involves using the open()
method. Refer to the following code snippet for a clearer understanding:
- import json
- FileName = "data.json"
- dataJSON = json.load(open(FileName))
- print(dataJSON)
Conclusion
In conclusion, the AttributeError: 'str' object has no attribute 'read'
arises when you try to use the read()
method on a string object instead of a file object. This error can be readily resolved by following the solutions I've presented above.
And there you have it! I hope this article will help you address the error that you are currently struggling with in you Python Project Developement. Explore more on this website for more Free Source Codes, Tutorials, and Articles that covers various programming languages.
Happy Coding =)
- 7589 views