Python ValueError: Mixing dicts with non-Series may lead to ambiguous ordering [Solved]
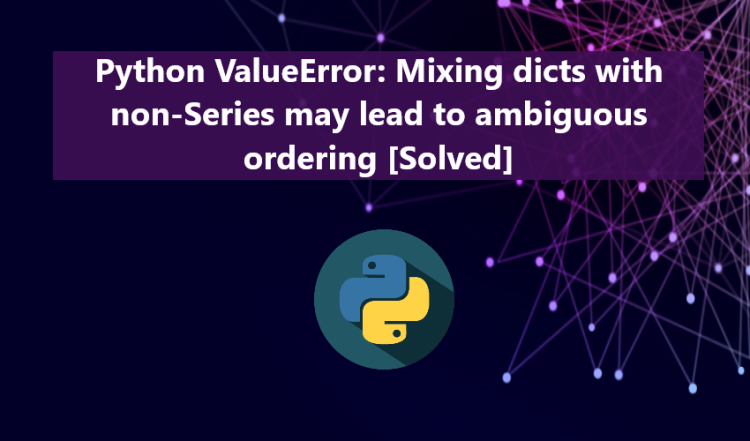
This article aims to thoroughly investigate the root causes and provide solutions for the Python ValueError message, which specifically presents as "ValueError: Mixing dicts with non-Series may lead to ambiguous ordering.". If you are currently grappling with this particular error in your Python project, please keep reading to gain a deeper understanding and discover how to both prevent and resolve this issue.
Python is a high-level, adaptable, and interpreted programming language known for its readability and user-friendliness. When developing applications or software with Python, various types of errors can arise due to coding mistakes. Some of these categorized as Python ValueError. This error type occurs when a function or operation receives an argument of the correct data type but with an inappropriate or invalid value. In addition to the specific error discussed in this article, Python ValueError can also manifest as the following exceptions:
You can click the provided error messages above to access their dedicated articles for in-depth information on these exceptions.
Why does the Python "ValueError: Mixing dicts with non-Series may lead to ambiguous ordering" occur?
The "ValueError: Mixing dicts with non-Series may lead to ambiguous ordering" Python error arises when attempting to convert a JSON object with an incompatible structure into a Pandas DataFrame
. Pandas
is a robust Python library used for data manipulation and analysis, and enforcing a strict data ordering. Combining ordered structures with unordered dictionaries can result in ambiguity regarding how the data should be merged or combined.
Below is a Python code snippet that demonstrates the occurrence of this error:
- import json
- import pandas as pd
- jsonFile = json.load(open('data.json'))
- # Set json data into Pandas DataFrame
- dataF = pd.DataFrame(jsonFile)
- # Output DataFrame
- print(dataF)
Below is a sample JSON data file for reference:
- {
- "status":{
- "code": 200,
- "msg": "success"
- },
- "data":[
- {
- "id":1,
- "name":"John Smith",
- },
- {
- "id":2,
- "name":"Claire Blake",
- },
- {
- "id":3,
- "name":"Mark Cooper",
- },
- {
- "id":4,
- "name":"Samantha Lou",
- },
- {
- "id":5,
- "name":"George Wilson",
- }
- ]
- }
Possible Solutions
Accessing Data by Key
To resolve the Mixing dicts with non-Series may lead to ambiguous ordering
error in Python, you can access the data by specifying the key associated with the data you intend to construct into a Pandas DataFrame
. You can achieve this by setting dataF['data']
to the desired tabular data.
- import json
- import pandas as pd
- jsonFile = json.load(open('data.json'))
- # Set json data into Pandas DataFrame
- dataF = pd.DataFrame(jsonFile['data'])
- # Output DataFrame
- print(dataF)
If you wish to merge or combine another JSON data object with the existing DataFrame
, you can employ the assign
method. Please refer to the following code snippet for a more comprehensive understanding of this process:
- import json
- import pandas as pd
- jsonFile = json.load(open('data.json'))
- # Set json data into Pandas DataFrame
- dataF = pd.json_normalize(jsonFile['data'])
- #merge status data with the dataframe
- dataF = dataF.assign(**jsonFile['status'])
- # Output DataFrame
- print(dataF)
Utilizing the Pandas json_normalize
Method
Alternatively, you can employ the json_normalize
method instead of using a DataFrame
to normalize the semi-structured JSON data into a flat table. To achieve the same outcome as the first solution provided, you'll still need to access the key associated with the stored data you wish to retrieve. Refer to the following example for a clearer understanding of this approach:
- import json
- import pandas as pd
- jsonFile = json.load(open('data.json'))
- # Retrieving Data Object into Flat Table
- dataF = pd.json_normalize(jsonFile, 'data')
- # Output DataFrame
- print(dataF)
In the snippet above, we've included two arguments in the json_normalize
method. The first argument is jsonFile
, representing the loaded JSON file object, and the second argument is data
, which serves as the key to extract the desired data from the JSON file.
Conclusion
In summary, the Python "ValueError: Mixing dicts with non-Series may lead to ambiguous ordering"
error typically occurs when using the Pandas library's DataFrame
method and attempting operations that combine Pandas Series or DataFrames with Python Dictionaries, potentially leading to ambiguous ordering. You can easily resolve this error by following one of the solutions provided in this article.
And there you have it! I hope that this article will assist you in addressing errors encountered in your current Python projects. Explore further on this website for additional Free Source Codes, Tutorials, and Articles covering various programming languages.