Python ValueError: Trailing Data [Solved]
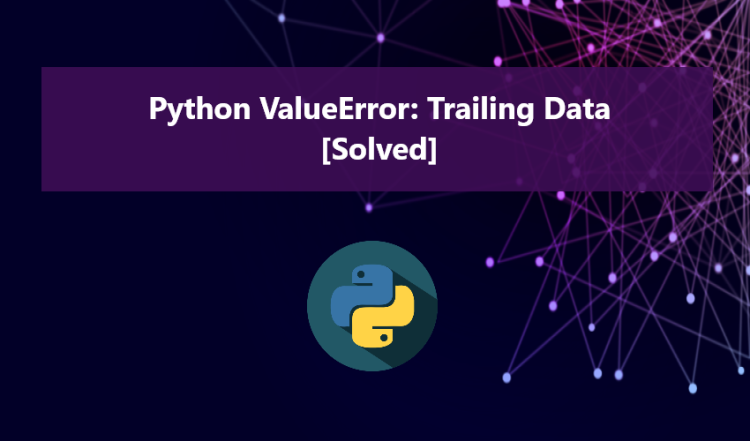
In this article, we will explore the causes of and solutions for the Python ValueError, specifically when it appears as ValueError: Trailing data. If you are currently facing this error during the development of your Python project, this article aims to provide insights into the reasons behind the error and how to rectify it.
In Python, there are various types of errors or exceptions that may occur during development, one of which is the ValueError, including the error discussed in this article. The ValueError occurs when a function receives an argument of the correct data type but is provided with an inappropriate or invalid value for that data type. It indicates that there is an issue with the value provided or passed to a function or operation.
Here are some other exceptions that are commonly encountered in Python development:
- "ValueError: cannot convert float NaN to integer"
- "TypeError: cannot convert the series to class 'float'"
- "AttributeError: 'DataFrame' object has no attribute 'concat'"
If you would like to gain a better understanding of these exceptions, you can click on the error messages to be redirected to their dedicated articles.
What Causes the Python ValueError: Trailing data Error?
The ValueError: Trailing data error in Python is often raised or triggered when data provided with the correct data type contains invalid additional data at the end. This error also occurs when using the Pandas Module to read a JSON file that has an invalid format.
To illustrate this error, let's assume we have a sample JSON file named data.json with the following content:
- {"ID": 1,"name": "Mark Cooper","age" : 27}
- {"ID": 2,"name": "Samantha Lou","age" : 27}
- {"ID": 3,"name": "Goerge Wilson","age" : 33}
Next, we will attempt to read the file's content as JSON data using the Pandas Module, specifically the read_json() method.
- import pandas as pd
- #JSON File path
- JSONpath = "./data.json"
- try:
- #Read JSON File
- convertedJSON = pd.read_json(JSONpath)
- print(convertedJSON)
- except ValueError as e:
- print("ValueError:", e)
Executing the provided sample snippet will result in an exception that reads "ValueError: Trailing data", causing the script execution to terminate abruptly. This error occurs due to an invalid JSON format, where the data is structured as multiple top-level JSON objects.
How to Fix the Python "ValueError: Trailing Data"
In the scenario and sample snippet provided above, you can resolve the "ValueError: Trailing data" error in Python by combining the object data in the JSON file. This solution is applicable when you have access to the loaded JSON file. Instead of the previous JSON file structure I provided above, you can format the data as follows:
- [{"ID": 1,"name": "Mark Cooper","age" : 27},
- {"ID": 2,"name": "Samantha Lou","age" : 27},
- {"ID": 3,"name": "Goerge Wilson","age" : 33}]
Furthermore, the Pandas Module provides the read_json() method with an argument called lines, which is designed to read the JSON file object line by line. Using this approach, the error can be avoided without the need to combine the object data within the file. Here's a snippet that demonstrates this solution:
- import pandas as pd
- #JSON File path
- JSONpath = "./data.json"
- try:
- #Read JSON File
- convertedJSON = pd.read_json(JSONpath, lines=True)
- print(convertedJSON)
- except ValueError as e:
- print("ValueError:", e)
Conclusion
In simple terms, the ValueError: Trailing data occurs when we attempt to execute a function or operation with the correct argument data type but in an invalid format. This error also surfaces when we try to read a JSON file with an invalid format using the Pandas read_json() method. By applying the provided solutions above, we can easily resolve this error.
And there you have it! I hope this article has provided you with insights into the error and helped you address it in your project development. Explore more on this website for moew Free Source Codes, Tutorials, and Articles covering various programming languages.
Happy Coding =)
- 699 views