Python Error TypeError: cannot convert the series to class 'float' [Solved]
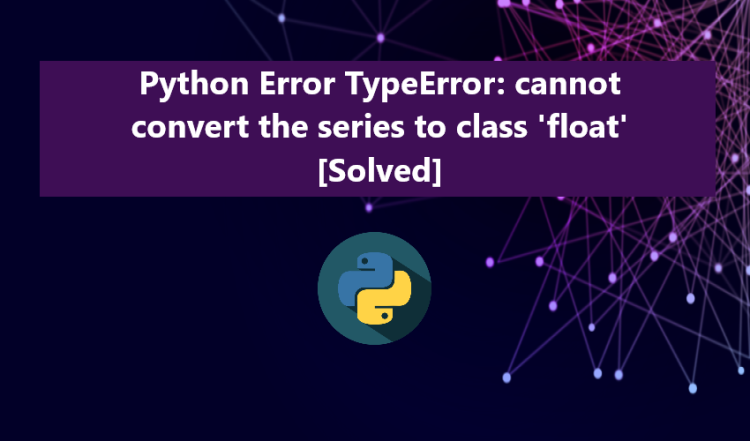
In this article, we will delve into the causes and solutions for a Python TypeError, specifically the error message that reads "TypeError: cannot convert the series to class 'float'". If you are new to the Python programming language and find yourself encountering this error during the development phase of your Python project, read on for insights into the root causes and remedies to resolve this issue.
The Python programming language is equipped with various types of errors and exceptions that can potentially disrupt the runtime of your application, causing it to crash or terminate abruptly. One such error is the TypeError. This type of error typically arises from the improper usage of object types within a function, operation, or method.
Here is a list of some TypeError occurrences that may arise during Python project development:
- "TypeError: 'dict_values' object is not subscriptable"
- "TypeError: can't subtract offset-naive and offset-aware datetimes"
- "TypeError: 'int' object is not callable"
To learn more about these specific Python errors, click on the error message itself to access the dedicated article discussing it.
What Causes the Python "TypeError: cannot convert the series to class 'float'" Error?
The "TypeError: cannot convert the series to class 'float'" error occurs when we attempt to convert a series of numerical data into a float, but the data within the series cannot be converted to a float. This error typically arises when non-numeric data is mixed with the series object or when we try to convert a pandas Series or DataFrame object's numbers into floats using Python's built-in function or method, known as float().
Scenario #1
Let's assume that we have defined an object containing a pandas DataFrame value, which includes a column with a series of numbers. Here's an example snippet illustrating this scenario:
- import pandas as pd
- # Simulate a TypeError: cannot convert the series to class float
- # Sample Data
- data = {'column1': [6.23, 15.7, 10.14]}
- #creating DataFrame using pandas DataFrame
- df = pd.DataFrame(data)
- #converting DF list item to float type
- result = float(df['column1'])
- #output
- print(result)
In the provided scenario, the data in the 'column1' DataFrame Column is being converted using Python's built-in method or function called float(). While the float() method can successfully convert a numeric value into a floating-point number, it encounters difficulties when attempting to convert a series of data. Consequently, running the given Python code will immediately halt script execution and generate the "TypeError: cannot convert the series to class 'float'" exception.
Solution
In this case, we can easily resolve the error by using the pandas astype method to convert the DataFrame's column data into floating-point values instead of using the built-in Python method. Here's a code snippet that illustrates this solution:
- data = {'column1': [6.23, 15.7, 10.14]}
- #creating DataFrame using pandas DataFrame
- df = pd.DataFrame(data)
- #converting DF list item to float type
- result = df['column1'].astype(float)
- #output
- print(result)
Scenario #2
Although the solution I provided works perfectly for the 1st Scenario (Scenario #1), there's still a possibility that you may encounter another error on your end due to the presence of mixed data types within the series object data.
Let's consider a situation where our DataFrame includes a non-numeric value within the object column that we're attempting to convert into a float type. Here's an example snippet illustrating this scenario:
- import pandas as pd
- # Sample Data
- data = {'column1': [6.23, 'twenty-three' , 15.7, 10.14]}
- #creating DataFrame using pandas DataFrame
- df = pd.DataFrame(data)
- #converting DF list item to float type
- result = df['column1'].astype(float)
- #output
- print(result)
In the context of this scenario and the sample snippet provided above, another exception will be raised, which reads "ValueError: could not convert string to float: 'twenty-three'". This error occurs when an attempt is made to convert series object data, which includes non-numeric values, into floating-point values.
Solution 2.1
We can still apply the same fix as we did in Scenario #1, but with a few additional tweaks to enable the conversion of the object data type.
Pandas also offers a method known as to_numeric(). This method allows us to convert Series or DataFrame data to numeric data types. In this case, non-numeric values will be converted to Null, enabling us to convert the DataFrame Column. Refer to the snippet below for a clearer understanding of this approach:
- import pandas as pd
- # Sample Data
- data = {'column1': [6.23, 'twenty-three' , 15.7, 10.14]}
- #creating DataFrame using pandas DataFrame
- df = pd.DataFrame(data)
- #remove non-numeric values
- df['column1'] = pd.to_numeric(df['column1'], errors='coerce')
- #converting DF list item to float type
- result = df['column1'].astype(float)
- #output
- print(result)
Solution 2.2
Furthermore, we can also handle the Null values in the DataFrame by setting or filling them with a default numeric value, such as 0. See the following example below:
- import pandas as pd
- # Sample Data
- data = {'column1': [6.23, 'twenty-three' , 15.7, 10.14]}
- #creating DataFrame using pandas DataFrame
- df = pd.DataFrame(data)
- #remove non-numeric values
- df['column1'] = pd.to_numeric(df['column1'], errors='coerce')
- #conver null values to 0
- df['column1'].fillna(0, inplace=True)
- #converting DF list item to float type
- result = df['column1'].astype(float)
- #output
- print(result)
Conclusion
In simple terms, the "TypeError: cannot convert the series to class 'float'" occurs when attempting to convert Series or DataFrame data into a float data type. Follow the recommended solutions to resolve the issue on your end and prevent it from recurring.
And there you have it! I hope you find this article valuable for enhancing your Python programming knowledge and skills. Explore more on this website for more Free Source Codes, Tutorials, and Articles for the latest updates and news covering various programming languages.
Happy Coding =)
- 4258 views