PHP Fatal error: Uncaught TypeError: array_merge(): Argument # must be of type array [Solved]
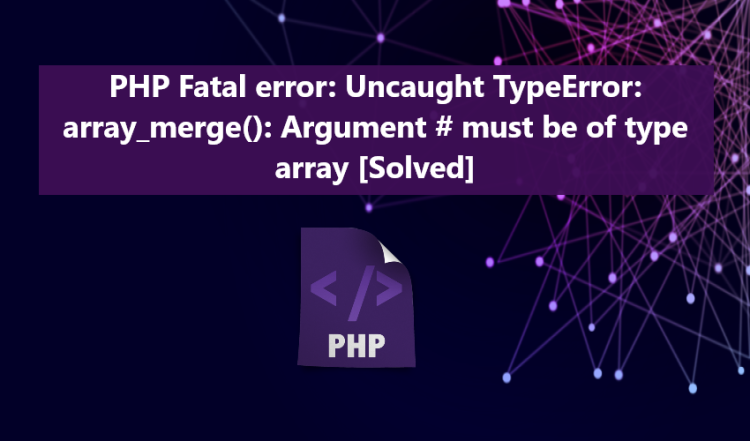
This article explores the causes and solutions for the PHP Fatal Error message that states "PHP Fatal error: Uncaught TypeError: array_merge(): Argument # must be of type array." Here, we will delve into the origins of this error and offer guidance on how to resolve this PHP fatal error. If you are currently grappling with this issue during your development phase, this article aims to provide you with insights and includes PHP code snippets that both illustrate the error and demonstrate how to avoid or fix it.
In PHP, a Fatal Error can bring your entire script execution to a halt or crash your application during runtime. These types of errors often occur when we attempt to use built-in functions or methods with incorrect arguments, types, and so on.
A PHP Fatal Error can manifest with the following message:
- "PHP Fatal error: Uncaught TypeError: implode(): Argument '...'"
- "PHP Fatal error: Call to a member function on a non-object"
- "Fatal Error: Cannot Redeclare class"
To gain deeper insights into the listed PHP errors, click on the respective error messages to be redirected to their dedicated article pages.
What Causes the "Fatal error: Uncaught TypeError: array_merge(): Argument # must be of type array" in PHP?
The "Fatal error: Uncaught TypeError: array_merge(): Argument # must be of type array" error occurs when we attempt to merge a list of arrays using PHP's built-in function called array_merge(). This error is triggered when a non-array argument is provided to the function.
The array_merge() built-in function in PHP is intended to merge a collection of items into one. This function requires a minimum of two arguments, and each of these must be of type array.
Here's an example PHP snippet that illustrates a scenario that triggers the emergence of the error:
- <?php
- // Example Group of data #1
- $data1 = [
- "id" => 1,
- "name" => "Mark Cooper"
- ];
- // Example Group of Data #2
- // Merging the 2 Group of Data into 1
- ?>
In the provided scenario, the value of the $data2 variable is decoded JSON data, which results in it being of type object or stdClass. This type mismatch is what triggers the error. As mentioned earlier, the array_merge() function requires its arguments to be of type array. Using an object or a string type will immediately stop execution and generate the "Fatal error: Uncaught TypeError: array_merge(): Argument # must be of type array".
Solutions
The straightforward solution for the "Fatal error: Uncaught TypeError: array_merge(): Argument # must be of type array" is to ensure that we provide arguments of type array to the array_merge() function.
In alignment with the previously mentioned scenario, we can prevent the error by simply converting the defined object into an array. We can accomplish this by defining the decoded JSON data as an array to force the object's conversion into an array. Here's a code snippet that demonstrates this solution:
- <?php
- // Example Group of data #1
- $data1 = [
- "id" => 1,
- "name" => "Mark Cooper"
- ];
- // Example Group of Data #2
- // Merging the 2 Group of Data into 1
- ?>
Furthermore, we can also implement a straightforward function or add a conditional parameter that validates and converts the defined variables into an array type. This approach is considered a best practice and proves valuable, particularly when dealing with dynamic values for the defined variables. Here's a code snippet that illustrates this approach:
- <?php
- function convertToArray($data){
- }else{
- return [];
- }
- }
- return $data;
- }
- // Example Group of data #1
- $data1 = [
- "id" => 1,
- "name" => "Mark Cooper"
- ];
- // Example Group of Data #2
- // validating the defined group of data as type of array
- $data1 = convertToArray($data1);
- $data2 = convertToArray($data2);
- // Merging the 2 Group of Data into 1
- ?>
Conclusion
In straightforward terms, the "Fatal error: Uncaught TypeError: array_merge(): Argument # must be of type array" error in PHP occurs when we use the array_merge() built-in function and attempt to supply non-array arguments. While this mistake can lead to application crashes, it can be easily rectified by following the solutions provided in this article.
So there you have it! I hope this article addresses the error you are currently facing and equips you with knowledge to prevent it from occurring in the future. Explore more on this website for additional Free Source Codes, Tutorials, and articles covering the latest news, bug fixes, and updates across various programming languages.
Happy Coding =)
- 563 views