How to Read File Line By Line in PHP?
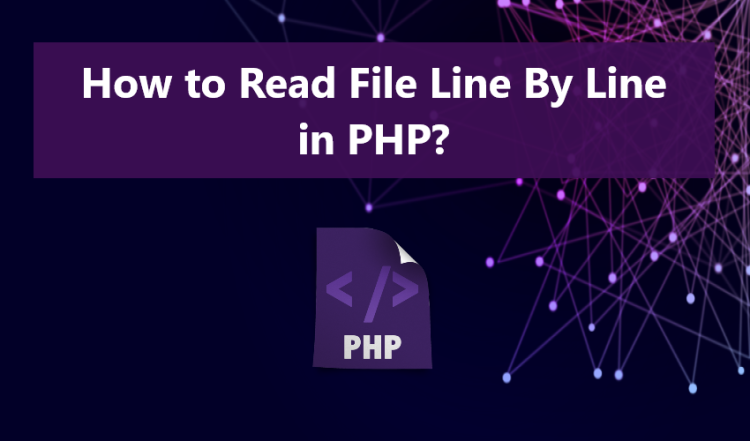
In this tutorial, we will explore various methods to Read Files Line by Line in PHP. PHP offers numerous built-in functions and approaches to accomplish this task. Moreover, this article aims to provide beginners and newcomers to the PHP language with valuable references to enhance their knowledge and skills.
Why is it Necessary to Read Files Line by Line in PHP?
Developing web applications or APIs can be complex, often requiring intricate logic and algorithms to meet project requirements. Reading the content of other files is a common practice in PHP projects, especially for systems or applications that handle and process large volumes of data, such as Importing and Exporting Table Data.
Reading files line by line in PHP is a technique that enables us to manage each line of a file's content. One practical example of this process is the implementation of an Environment Variable (.env file) in our projects. Developers often use this type of file to store and define essential constants and variables crucial to the project's development phase.
What are the 5 Ways for Reading Files Line by Line in PHP?
As mentioned earlier, there are multiple ways to achieve the task of reading each line of a file in PHP. Below are five PHP built-in methods or functions to accomplish this:
- Using the fgets() function
- Using the file() function
- Using the file_get_contents() function
- Using the SplFileObject class
- Using the stream_get_line() function
Take a look at the following code snippets to gain a better understanding of how to use these PHP functions and objects to achieve the feature discussed in this article.
Using the fgets() function
The fgets() function in PHP is designed to retrieve lines from a file. It requires a valid file pointer, which can be created using functions like fopen() and fsockopen(). The code snippets below illustrate how to use this function to read a file line by line:
- <?php
- /**
- * Using the `fgets()` function
- */
- $envFile = "sample-env.txt";
- // Iterator Number
- $i = 0;
- if($file){
- // If file is open
- // Skipping the empty line and Comment line
- continue;
- $i++;
- // Output Line Content
- echo "[Line #{$i}]: {$line}";
- }
- }
- ?>
Using the file() function
PHP offers a built-in function called file() that is specifically designed for reading and retrieving the entire lines of a file, returning them as an array. This function provides multiple flags that are highly useful depending on how we want to read the file. One such flag is FILE_SKIP_EMPTY_LINES, which is used to skip empty lines while reading the file. Refer to the code snippet below for a clearer understanding:
- <?php
- /**
- * Using the `file()` function
- */
- $envFile = "sample-env.txt";
- // Iterator Number
- $i = 0;
- // If having data
- foreach($lines as $line){
- // Skipping the empty line and Comment line
- continue;
- $i++;
- // Output Line Content
- echo "[Line #{$i}]: {$line}";
- }
- }
- ?>
Using the file_get_contents() function
The file_get_contents() function in PHP is designed to retrieve and read the entire content of a file, returning it as a string. This function can also be utilized to achieve our desired feature. By combining it with preg_split() or explode() functions, we can split the string into lines. Please refer to the code snippet below for a more comprehensive understanding:
- /**
- * Using the `file_get_contents()` function
- */
- $envFile = "sample-env.txt";
- // Iterator Number
- $i = 0;
- // If lines exists
- foreach($lines as $line){
- // Skipping the empty line and Comment line
- continue;
- $i++;
- // Output Line Content
- echo "[Line #{$i}]: {$line}\n";
- }
- }
- ?>
Using the SplFileObject class or object
In addition, PHP offers a built-in object-oriented programming class (OOP) called SplFileObject, specifically designed for creating new file objects. This class encompasses various properties and methods that prove valuable for handling and reading file content. One such method is fgets(), which is employed to retrieve lines from the file.
- <?php
- /**
- * Using the `SplFileObject` class
- */
- $envFile = "sample-env.txt";
- $file = new SplFileObject($envFile);
- // Iterator Number
- $i = 0;
- while(!$file->eof()){
- // Getting the current line
- // Skipping the empty line and Comment line
- continue;
- $i++;
- // Output Line Content
- echo "[Line #{$i}]: {$line}\n";
- }
- ?>
Using the stream_get_line() function
Lastly, the stream_get_line() function provides another way to read file content line by line. This function is designed to retrieve lines from a streamed file up to a specified delimiter. Its functionality is nearly similar to the fgets() function.
- <?php
- /**
- * Using the `stream_get_line` function
- */
- $envFile = "sample-env.txt";
- // Iterator Number
- $i = 0;
- if($file){
- // File is open
- // Skipping the empty line and Comment line
- continue;
- $i++;
- // Output Line Content
- echo "[Line #{$i}]: {$line}\n";
- }
- }
- ?>
Conclusion
Reading File Content Line by Line in PHP is a frequently employed feature when handling or processing file content in PHP. This approach can be accomplished through various methods using valuable built-in objects and functions provided by PHP, including the approaches I've outlined in this tutorial.
And there you have it! I hope that this tutorial will assist you in implementing your desired algorithms in your current PHP project or provide you with new knowledge that could prove beneficial for your future endeavors.
Feel free to explore further on this website for additional Free Source Code, Tutorials, and Articles covering a wide range of programming and technology topics and updates.