How to Remove Multiple Element from a List in Python
In this tutorial, we will program "How to Remove Multiple Elements from a List in Python." We will learn how to efficiently remove multiple elements from a list. The objective is to identify and remove only the elements you wish to remove from the list. I will provide a sample program to demonstrate the actual coding process in this tutorial.
This topic is very easy to understand. Just follow the instructions I provide, and you'll be able to do it yourself with ease. The program I’ll show you demonstrates the proper way to remove multiple elements. I'll do my best to provide a simple method for removing only the selected elements. So, let's start coding!
Getting Started:
First you will have to download & install the Python IDLE's, here's the link for the Integrated Development And Learning Environment for Python https://www.python.org/downloads/.
Creating Main Function
This is the main function of the application. The following code will display a simple GUI in terminal console that will display program. To do this, simply copy and paste these blocks of code into the IDLE text editor.- def addList(no):
- i=0
- indexes_to_remove=[]
- while i < no:
- el=int(input("Enter a number to remove from a list: "))
- indexes_to_remove.append(el)
- i+=1
- if i == no:
- break
- return indexes_to_remove
- ret = False
- while True:
- print("\n================= Remove Multiple Element from a List =================\n\n")
- numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
- print("My List: ", numbers)
- print("\n")
- no= int(input("How many elements? "))
- print("\n")
- for i in sorted(addList(no), reverse=True):
- del numbers[i-1]
- print("New list:", numbers)
- opt = input("\nDo you want to try again?(yes/no): ")
- if opt.lower() == 'yes':
- ret=False
- elif opt.lower() == 'no':
- ret=True
- print("Exiting program....")
- else:
- print("Please enter yes/no:")
- break
- if ret == False:
- continue
This Python script removes multiple elements from a predefined list based on user input. It first asks how many elements the user wants to remove, then prompts for the indexes of these elements. The program deletes the specified elements from the list and displays the updated list. The process repeats until the user decides to exit.
Output:
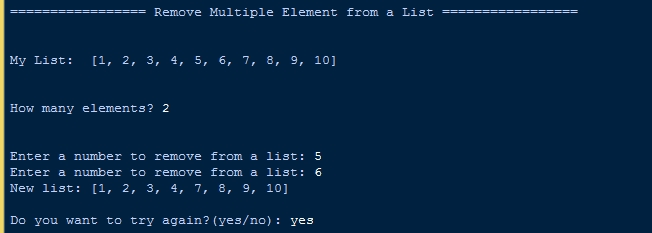
There you have it we successfully created How to Remove Multiple Element from a List in Python. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for Python Language
Add new comment
- 27 views