How to fix the 'a session had already been started' PHP error?
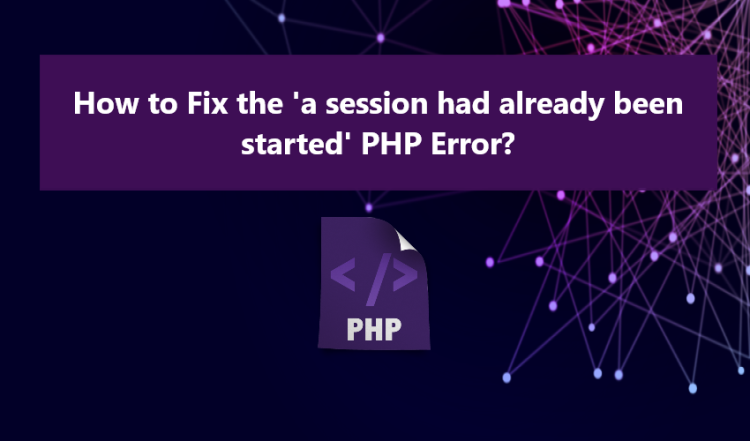
This article delves into the reasons behind the occurrence of the PHP warning or error message 'a session had already been started' and provides solutions to prevent this error from arising. Within this context, a straightforward code snippet will be presented to illustrate the emergence of the error, followed by another snippet that rectifies the issue.
Constructing a web application utilizing PHP as the primary programming language can be complicated depending to the scope of the project. During the developmental stage, it's possible to encounter errors that arise due to various factors, potentially leading to abrupt termination or even application crashes. If you currently find yourself grappling with the PHP error message, specifically 'a session had already been started', this article is poised to assist you in comprehending and circumventing the problem.
What is PHP SESSION?
The PHP SESSION refers to an associative array comprising session variables that remain accessible to the ongoing script. It provides a means to temporarily store data that remains accessible across all pages of a website. Frequently, it is employed to retain information pertaining to users who have logged into the website, serving the purpose of authentication.
The subsequent section outlines various features commonly employed in web applications that utilize PHP SESSION:
Why does 'a session had already been started' PHP error/warning occurs?
The PHP Error or Warning that displays the message 'a session had already been started', or the notice appears as PHP Notice: session_start(): Ignoring session_start() because a session is already active (in the most recent PHP version), emerges when attempting to initiate a PHP session using the session_start() function. This error occurs due to redundant invocations of the said function within the code.
Root causes contributing to the occurrence of this error:
- Included File(s) Also Call 'session_start()': If included files also contain calls to the 'session_start()' function, it can lead to multiple session initiations.
- Multiple session_start() Calls: When there are multiple instances of the 'session_start()' function being called in the code, it can result in conflicts and the associated error.
Sample Snippet
Here's an example snippet that illustrate a scenario to the error to occur.
- <?php
- // Load Other PHP File Script
- include_once('./config.php'); //This file contains session_start() script also
- // Start Session
- // Set Session data
- $_SESSION['user_id'] = 1;
- $_SESSION['name'] = "Mark Cooper";
- // Output
- echo "[{$_SESSION['user_id']}] {$_SESSION['name']}";
- ?>
Solution
To effectively fix the 'session has already been started' error message in PHP, a straightforward solution involves introducing a conditional parameter into your code to initially verify if a session is already active. To achieve this, you can employ a comparison between the PHP predefined constant PHP_SESSION_NONE and the built-in function session_status().
PHP_SESSION_NONE serves as an inherent PHP constant with a value of 1, signifying that sessions are enabled but not yet initiated.
The PHP session_status() function is a built-in utility that examines the current status of the session and subsequently returns this status.
Presented below is a concise code snippet that effectively illustrates the outlined solution:
- <?php
- // Load Other PHP File Script
- include_once('./config.php'); //This file contains session_start() script also
- if(session_status() == PHP_SESSION_NONE){
- // Start Session it is not started yet
- }
- // Set Session data
- $_SESSION['user_id'] = 1;
- $_SESSION['name'] = "Mark Cooper";
- // Output
- echo "[{$_SESSION['user_id']}] {$_SESSION['name']}";
- ?>
The solution provided above can be use only if you are using PHP version >= 5.4.
If you are using the PHP verion 5.4, the snippet below is the alternative solution.
- <?php
- // Load Other PHP File Script
- include_once('./config.php'); //This file contains session_start() script also
- // Start Session it is not started yet
- }
- // Set Session data
- $_SESSION['user_id'] = 1;
- $_SESSION['name'] = "Mark Cooper";
- // Output
- echo "[{$_SESSION['user_id']}] {$_SESSION['name']}";
- ?>
Conclusion
To put it simple, the PHP error or warning displaying the message 'a session has already been started', or the PHP Notice: session_start(): Ignoring session_start() because a session is already active, arises when an attempt is made to initiate a PHP session multiple times. This error can be resolved by ensuring that the session_start() function is invoked only once within the script on the page. Furthermore, incorporating a conditional check to verify whether the session has already commenced is a valuable approach and considered one of the best practices when initializing a PHP session.
There you go! I hope this article helps you with what you are looking for to fix the error that you are currently facing. Explore more on this website for more Free Source Codes, Tutorials, and Articles in any proramming languages available.