How to fix 'Warning: array_key_exists(): The First Argument Should Be Either a String or an Integer' error in PHP
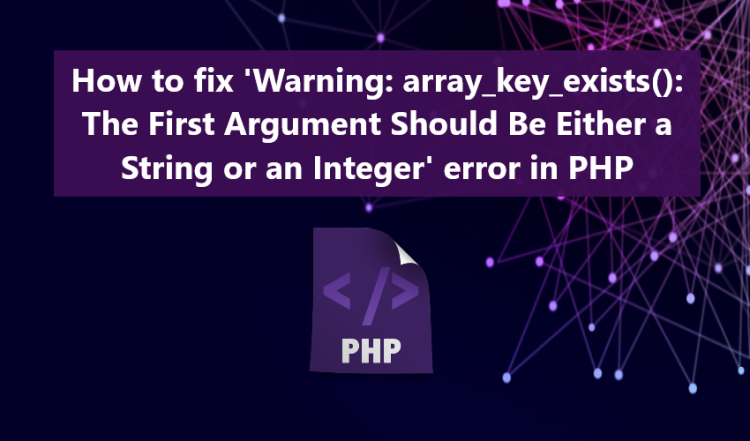
This article delves into exploring the causes and solutions for a PHP error that can potentially manifest while working on an application using the PHP language, specifically the 'Warning: array_key_exists(): The First Argument Should Be Either a String or an Integer' error. If you happen to encounter this error, this article aims to assist you in addressing it. I will provide some code snippets that simulate the error scenario and provide resolutions to correct or prevent it.
Why does the 'Warning: array_key_exists(): The First Argument Should Be Either a String or an Integer' occurs?
The 'Warning: array_key_exists(): The First Argument Should Be Either a String or an Integer' error occurs when we try to use an invalid argument within a PHP class or function. In this context, the array_key_exists() function is the point of concern. This error is categorized as a type error, as it verifies whether the provided argument corresponds to the expected type(s) of the class or function.
In later PHP versions, this error now appears as 'PHP Fatal error: Uncaught TypeError: Illegal offset type in ...'. Recent PHP updates have introduced changes in how error messages are presented, making it easier for developers to understand the root cause of errors. Alongside the mentioned error type, there exists a variety of other type errors that developers may come across. An example of such an error is the 'PHP Fatal error: Uncaught TypeError: implode(): Argument ...', which indicates that the provided argument types don't match the expected types.
Here's an example snippet that returns the said error:
- <?php
- // Sample array to Check
- $arr = [
- "a" => "foo",
- "b" => "bar"
- ];
- $keys = ['a', 'b', 'c'];
- // Checking the existence of a specific test
- if ($check) {
- echo "The key 'a' is existing in the array!";
- } else {
- echo "The key 'a' does not exists!";
- }
- ?>
How to fix or prevent 'Warning: array_key_exists(): The First Argument Should Be Either a String or an Integer' or 'PHP Fatal error: Uncaught TypeError: Illegal offset type in ...'?
The solution to this kind of PHP TypeError is simple which is by confirming that the arguments provided for the array_key_exists() function correspond to the anticipated types. Furthermore, consider incorporating conditional parameters that assess the argument's validity prior to function execution. This strategy safeguards against the emergence of errors and mitigates the risk of process halts or code failures.
Here are some snippets that can be use to fix the error in the provided simulation snippet:
Using an Integer or String type on the first argument
- <?php
- // Sample array to Check
- $arr = [
- "a" => "foo",
- "b" => "bar"
- ];
- $keys = ['a', 'b', 'c'];
- // Checking the existence of a specific test
- if ($check) {
- echo "The key 'a' is existing in the array!";
- } else {
- echo "The key 'a' does not exists!";
- }
- ?>
Adding Conditional Checks
- <?php
- // Sample array to Check
- $arr = [
- "a" => "foo",
- "b" => "bar"
- ];
- $keys = ['a', 'b', 'c'];
- foreach($keys as $k => $v){
- // Checking the existence of a specific test
- if ($check) {
- echo "The key '{$keys[$k]}' is existing in the array! \n";
- } else {
- echo "The key '{$keys[$k]}' does not exists! \n";
- }
- }
- }
- ?>
or
- <?php
- // Sample array to Check
- $arr = [
- "a" => "foo",
- "b" => "bar"
- ];
- $key = ['a', 'b', 'c'];
- // Checking the existence of a specific test
- if ($check) {
- echo "The key '{$key}' is existing in the array! \n";
- } else {
- echo "The key '{$key}' does not exists! \n";
- }
- }else{
- echo "Invalid type of key";
- }
- ?>
There you go! I hope this article helps you with your current situation for fixing the error in your PHP development phase.
If you also come accross with the following PHP errors, by clicking the provided links will redirect you to the articles that will help you to understand and fix it.
- How to fix Notice: Undefined index ...?
- How to fix Trying to access array offset on value of type null?
- How to fix PHP Warning: foreach() argument must be of type array|object ..?
Explore more on this website for more Tutorials, Free Source Codes, and Articles about fixing some error in different programming languages.
Happy Coding =)
- 394 views